Python 3: Input in cmd to return functions
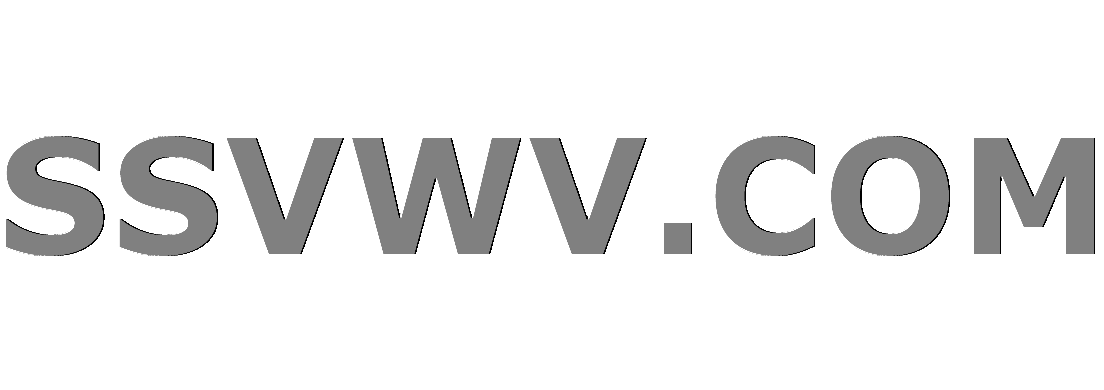
Multi tool use
Python 3: Input in cmd to return functions
I'm currently working my way through "Learn Python the Hard Way" and got to the first exercise about functions. It's simply creating a few functions and printing them out like in the previous examples in the book`.
Code:
def print_two(*args):
arg1, arg2 = args
print("arg1: %r, arg2: %r" % (arg1, arg2))
def print_two_again(arg1, arg2):
print("arg1: %r, arg2: %r" % (arg1, arg2))
def print_one(arg1):
print("arg1: %r" % (arg1))
def print_none():
print("I got nothing.")
print_two("Zed","Shaw")
print_two_again("Zed","Shaw")
print_one("First!")
print_none()
Output in cmd:
C:Users[USER]Google DrivePythonLearn Python the Hard Way>python ex18.py
arg1: 'Zed', arg2: 'Shaw'
arg1: 'Zed', arg2: 'Shaw'
arg1: 'First!'
I got nothing.
I want to play around a bit with this, so instead of just giving me the above four lines when I run it, I want to be able to input the name of the function and then return the result. I tried with the following, but maybe I just don't understand how Python works?
x = input("> ")
print(x)
I'm not quite sure on the terminology but it would give me the following in cmd:
C:Users[USER]Google DrivePythonLearn Python the Hard Way>python ex18.py
> print_none() # This is something I write myself
I got nothing.
I tried to change the question, does it make sense now?
– Dennis Christiansen
Jul 1 at 14:47
Yeah, so you'd like to specify a method name and then execute it and print a result to the console.
– user8035311
Jul 1 at 14:49
Yeah, that´s what I'm trying to do.
– Dennis Christiansen
Jul 1 at 14:52
I rolled back your latest edit (it's still available from the revision history) - your question should remain strictly a question, though you are most welcome to post an answer of your own, and even accept it to mark this question as resolved. See also help.
– tripleee
Jul 2 at 9:58
2 Answers
2
The question might have been a little vauge, but I found a solution myself. What I was looking for was the "import" function of the script into cmd, which then allows input the commands and return the lines that were hardcoded before:
C:Users[User]Google DrivePythonLearn Python the Hard Way>python
Python 3.7.0 (v3.7.0:1bf9cc5093, Jun 27 2018, 04:59:51) [MSC v.1914 64 bit (AMD64)] on
win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import ex18
arg1: 'Zed', arg2: 'Shaw'
arg1: 'Zed', arg2: 'Shaw'
arg1: 'First!'
I got nothing.
>>> ex18.print_none()
I got nothing.
>>>
def print_two_again(arg1, arg2):
print("arg1: %r, arg2: %r" % (arg1, arg2))
def print_one(arg1):
print("arg1: %r" % (arg1))
if __name__ == '__main__':
x = input("F name:")
eval(x)
Example:
/home/denis/zmqPG/bin/python /home/denis/PycharmProjects/useless/so2.py
F name:print_one(5)
arg1: 5
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Return a result of a method call?
– user8035311
Jul 1 at 14:38