Serializing List to JSON in C# creates backslashes in front of quotes
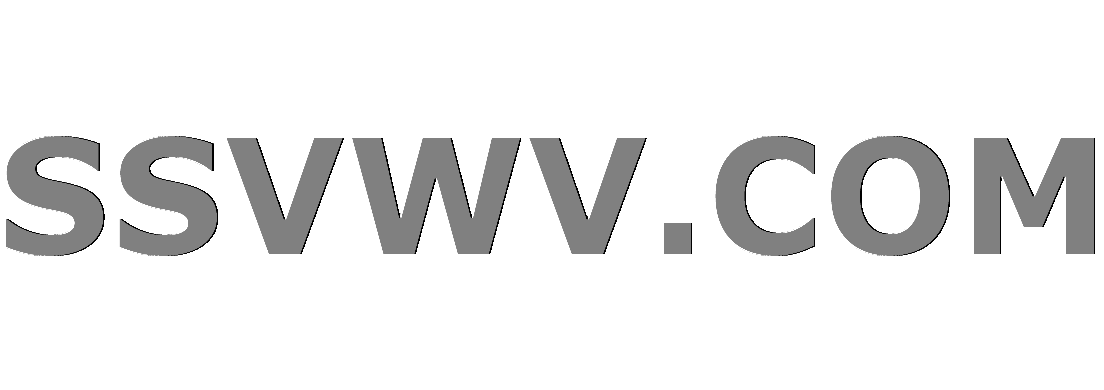
Multi tool use
Serializing List to JSON in C# creates backslashes in front of quotes
I am trying to serialize a List in C# and it is mostly working fine except that it creates backslashes in front of the double quotes. I have read online that this is a result from serializing the data twice and I have tried different approaches to removing the backslashes but they are not really working for me.
C# code (Using NewtonSoft.Json Library):
List<string> list_element_object = new List<string>();
foreach (var list_element in total_lists)
{
/* Code to get all of the 'element_lists' data
Which is eventually used to create the 'columns' data below */
var columns = new Dictionary<string, string>
{
{"@type", "Element_Lists" },
{"Name", Element_List_Name },
{"Description", Element_List_Description},
{"URL", Element_URL }
};
var serialized = JsonConvert.SerializeObject(columns);
// Add the serialized object to the list
list_element_object.Add(serialized);
}
// Serlialize the list containing the data and store into a ViewBag variable to use in a View
ViewBag.Element_Data_Raw = JsonConvert.SerializeObject(list_element_object);
Output:
"{"@type":"Elements","Name":"Some_Element_Name","Description":"Some_Element_Description","URL":"Some_Element_URL"}"
Expected Output:
"{"@type":"Elements","Name":"Some_Element_Name","Description":"Some_Element_Description","URL":"Some_Element_URL"}"
Any help is greatly appreciated!
columns
@john Is there a more efficient way of achieving this type of serialization without the backslashes in front of the quotes?
– Jeff P.
Jul 2 at 0:44
And why do you add the already serialized data to a list and serialize that list again?
– sticky bit
Jul 2 at 0:44
@Jeff: Simply put, don't serialize the dictionary data twice (once by itself, and then once as an item in a list of strings).
– john
Jul 2 at 0:46
the first output, where did you get it? debug? or dump it to response/text file? on debug, all double quotes will be escaped (have slashes).
– Bagus Tesa
Jul 2 at 0:50
1 Answer
1
I'm still not 100% certain on your question, but this should produce normal JSON output, which I guess is what you want. As noted by Bagus Tesa in the comments, double quotes will be escaped in the debugger display. Since you're double-serializing a dictionary (i.e. first you're serializing it to a string, and then you're serializing that string), you're bound to have escaped strings in your current output.
List<Dictionary<string, string>> list_element_object = new List<Dictionary<string, string>>();
foreach (var list_element in total_lists)
{
/* Code to get all of the 'element_lists' data
Which is eventually used to create the 'columns' data below */
var Element_List_Name = list_element.Element_List_Name;
var Element_List_Description = list_element.Element_List_Description;
var Element_URL = list_element.Element_URL;
var columns = new Dictionary<string, string>
{
{"@type", "Element_Lists" },
{"Name", Element_List_Name },
{"Description", Element_List_Description},
{"URL", Element_URL }
};
// Add the serialized object to the list
list_element_object.Add(columns);
}
// Serlialize the list containing the data and store into a ViewBag variable to use in a View
ViewBag.Element_Data_Raw = JsonConvert.SerializeObject(list_element_object);
Changing the list to store dictionaries, and then serializing only the list will produce JSON like this (I've formatted it for easy viewing):
[
{
"@type": "Element_Lists",
"Name": "Some_Element_Name",
"Description": "Some_Element_Description",
"URL": "Some_Element_URL"
}
]
Try it online
This answer is perfect and I realize why my error was occurring from my code. So you were right in that i was serializing my List twice, which resulted in the backslashes in front of the double quotes. After observing your first line of code,
List<Dictionary<string, string>> list_element_object = new List<Dictionary<string, string>>();
, I was able to create a list of Dictionary items and then eventually serialize that list only once. By using this approach, I only serialized this data once and it didn't create the backslashes. Thank you!– Jeff P.
Jul 2 at 1:30
List<Dictionary<string, string>> list_element_object = new List<Dictionary<string, string>>();
You're welcome. I'm glad I could help :)
– john
Jul 2 at 1:31
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Of course it does. You're serializing a dictionary, adding it to a list (i.e. a list of strings) and then serializing that list. If this isn't what you want, you should add
columns
itself (not serialized) to a list and then serialize that list. If you want something else, please can you update your question to reflect what you actually expected to happen.– john
Jul 2 at 0:43