Promise.all does not check for/store all promises
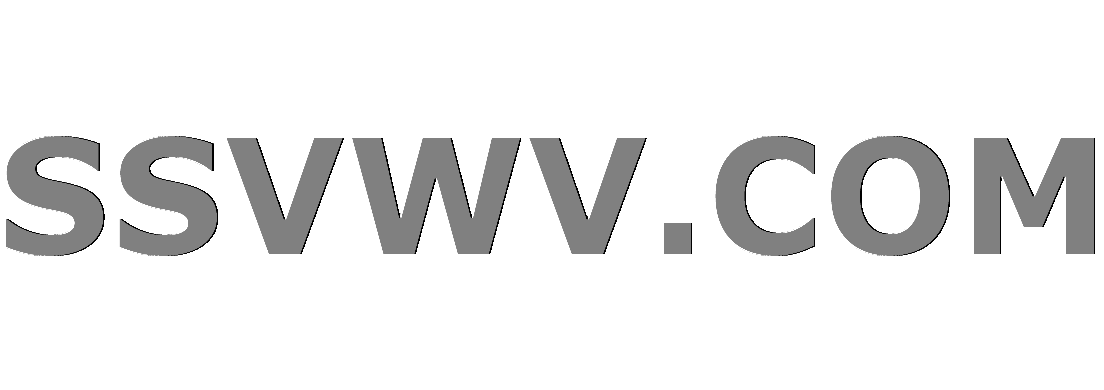
Multi tool use
Promise.all does not check for/store all promises
I have problem with promise.All not catching all the promises to resolve...
short example:
const express = require('express');
const app = express();
const router = express.Router();
const request = require('request');
router.get('/', function(req, res)
{
var promiseArray = ;
function promiseResolve (data) {
return new Promise (function (resolve) {
resolve(data);
});
}
var data1 = 1;
promiseArray.push(promiseResolve(data1));
var data2 = 2;
promiseArray.push(promiseResolve(data2));
var data3 = 3;
promiseArray.push(promiseResolve(data3));
console.log(promiseArray);
**// all is fine**
**// but when we add promise in some asynchronous operation like... request for example:**
var test = request('https://www.google.com', function (err, res, content) {
if (res.statusCode == 200)
{
promiseArray.push(promiseResolve(content));
console.log(content);
}
});
Promise.all(promiseArray).then(function(data) {
console.log(data);
});
**// it does not store promise in a array... I thought that at first promise.All always 'check' if all promises are stored, then execute those by resolve, reject...**
res.render('index');
});
module.exports = router;
comment are in code, but long story short - promise.All does not check for/store all promises.
Some more text for SO 'algorithm'... and more... and more... and more...
Promise.all(promiseArray)
also, your
promiseResolve
function is not required, as Promise.all can handle non-promises in the array– Jaromanda X
Jul 2 at 0:35
promiseResolve
yea but I thought promise.All check/wait for all promises to be in array... but I guess it's not and it's default behavior... so I need to use callback or another promise?
– Thave1957
Jul 2 at 0:36
no, it waits for all promises in the array to resolve - answer below is what you need - and, as I pointed out, you don't need the
promiseResolve
function for two reasons ... 1) Promise.resolve
does the same thing and 2) Promise.all promisifies any non-promises in the array– Jaromanda X
Jul 2 at 0:37
promiseResolve
Promise.resolve
Promise.all
waits for all Promises that are in the array at the moment Promise.all
is called, but not for Promises that happen to be added to the array later.– CertainPerformance
Jul 2 at 0:37
Promise.all
Promise.all
1 Answer
1
At the time Promise.all(promiseArray)
runs, promiseArray
is only composed of the first three Promises - the fourth one from request
does not get added to promiseArray
until after the request goes through, by which time the Promise.all
has already resolved. You'll have to explicitly construct a Promise
for the request
so that promiseArray
has all necessary Promises
before Promise.all
is called:
Promise.all(promiseArray)
promiseArray
request
promiseArray
Promise.all
Promise
request
promiseArray
Promises
Promise.all
promiseArray.push(new Promise((resolve, reject) => {
request('https://www.google.com', function (err, res, content) {
if (res.statusCode == 200) resolve(content);
else reject('bad statusCode');
});
}));
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
well, that last promise isn't in the array when you call
Promise.all(promiseArray)
- because it is added to the array asynchronously ..– Jaromanda X
Jul 2 at 0:34