BringToFront() does not work with ConstraintLayout
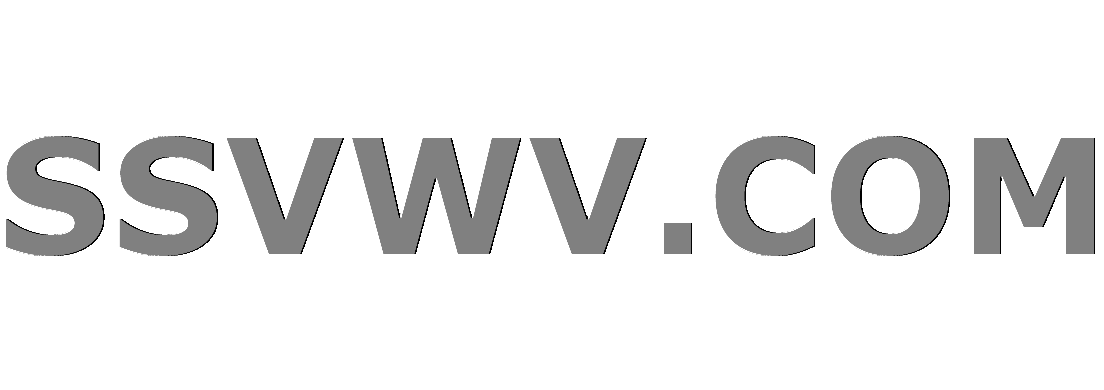
Multi tool use
BringToFront() does not work with ConstraintLayout
I need to bring an ImageView up above all the layouts to show that it is overlapping to the TextView. Unluckily, none of what I've tried from SO helped me. This is what's happening.
main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/txtTitle"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/holo_orange_light"
android:gravity="center"
android:padding="10dp"
android:text="Students"
android:textSize="25sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<FrameLayout
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/txtTitle">
<android.support.constraint.ConstraintLayout
android:id="@+id/constraintLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/fraLayout1"
android:elevation="2dp"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<ImageView
android:id="@+id/imgKOala"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:layout_marginTop="-55dp"
android:src="@drawable/koala" />
</FrameLayout>
</android.support.constraint.ConstraintLayout>
</FrameLayout>
</android.support.constraint.ConstraintLayout>
main.java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ImageView imgKOala = (ImageView) findViewById(R.id.imgKOala);
imgKOala.bringToFront();
imgKOala.invalidate();
imgKOala.requestLayout();
ViewCompat.setTranslationZ(imgKOala, 1000);
}
How can I properly make the ImageView appear above the TextView Students?
This is only a representation of what I really want to achieve.
Why are you calling invalidate after bringToFront?
– Ricardo
Jul 2 at 13:22
3 Answers
3
Try this one
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/txtTitle"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/holo_orange_light"
android:gravity="center"
android:padding="10dp"
android:text="Students"
android:textSize="25sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imgKOala"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:layout_marginTop="-55dp"
android:src="@mipmap/ic_launcher">
</android.support.constraint.ConstraintLayout>
I really need the ImageView to be deeper than the TextView.
– Mr. Nacho
Jul 2 at 12:47
Set an elevation on the ImageView
. 2dp
seems to work.
ImageView
2dp
android:elevation="2dp"
Hope this helped!
The bringToFront
and Z-order of views are all related to the view's parent view, so if you call bringToFront
on your imgKOala
, it just at the front of its parent view fraLayout1
. If you want imgKOala
overlap the TextView
, you should evaluate the FrameLayout
which is sibling to the TextView
.
bringToFront
bringToFront
imgKOala
fraLayout1
imgKOala
TextView
FrameLayout
TextView
In you layout file, your FrameLayout
have the property:
FrameLayout
app:layout_constraintTop_toBottomOf="@id/txtTitle"
and ImageView
:
ImageView
android:layout_marginTop="-55dp"
so your ImageView is not fully displayed, because the top 55dp of it is outside of its parent.
Try the code below:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/txtTitle"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/holo_orange_light"
android:gravity="center"
android:padding="10dp"
android:text="Students"
android:textSize="25sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
<FrameLayout
android:id="@+id/frameLayout"
android:layout_width="0dp"
android:layout_height="0dp"
android:elevation="2dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<android.support.constraint.ConstraintLayout
android:id="@+id/constraintLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/fraLayout1"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<ImageView
android:id="@+id/imgKOala"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:src="@mipmap/koala" />
</FrameLayout>
</android.support.constraint.ConstraintLayout>
</FrameLayout>
</android.support.constraint.ConstraintLayout>
just set android:elevation=2dp
on the FrameLayout which is sibling to the TextView, and the code in your onCreate()
method can be removed.
android:elevation=2dp
onCreate()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
you want to keep imageview on top of all your views right ?
– Umair
Jul 2 at 12:28