Word Counter in c doesnt work
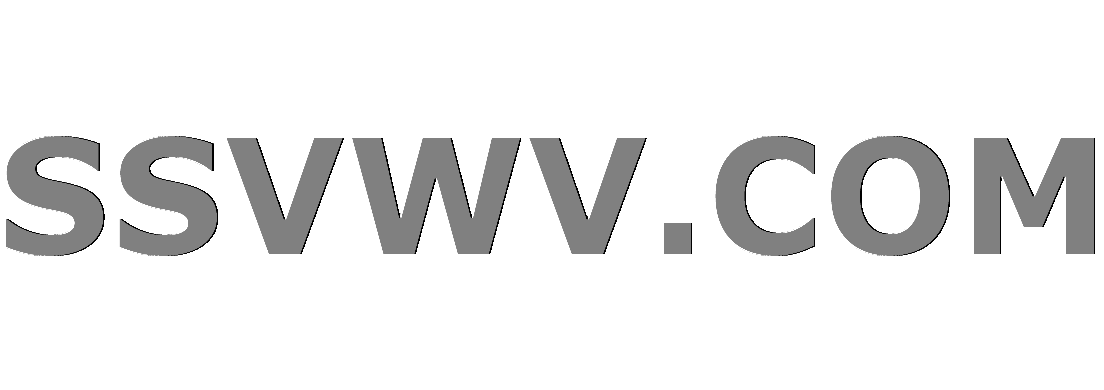
Multi tool use
Word Counter in c doesnt work
I have a c code like below.
I want to count the number of words in a text delimited with a delimiter.
The code compiles but stops.
What is the problem?
This is my code below.
#include <stdio.h>
#include <string.h>
int WordCount(char *text,char delimiter)
{
char *s;
int count = 0;
strcpy(s,text);
while(*s){
if(*s==delimiter){
count++;
}
}
return count;
}
int main(void)
{
char *line = "a,b,c,d,e";
printf("%dn",WordCount(line,','));
return 0;
}
3 Answers
3
You forgot to increment the pointer s
, thus you had an infinite loop, and instead of copying the string (for which you would need to allocate memory), just let it point to the input.
s
int WordCount(char *text,char delimiter)
{
char *s = text;
int count = 0;
// strcpy(s,text);
while(*s){
if(*s==delimiter){
count++;
}
++s;
}
return count;
}
char *s;
int count = 0;
strcpy(s,text);
s
is an uninitialized pointer not an array object.
s
char *s;
- allocate memory for s
in stack or heap.
char *s;
s
Mistakes in your program
Modify your code like below
...
char *s = NULL;
int count = 0;
s = text;
while(*s);
{
if (*s == delimiter)
{
count++;
}
s++;
}
...
He doenst need to make a copy, thus why allocate?
– trumpetlicks
Feb 1 '13 at 19:30
updated my answer
– rashok
Feb 1 '13 at 20:53
So now you are always updating s with s++, and if s[count] == the delimeter, you essentially are double counting, because you increment count also then referencing using s[count]
– trumpetlicks
Feb 1 '13 at 21:05
typo mistake...
– rashok
Feb 1 '13 at 21:08
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You arent incrementing your pointer s to actually MOVE through the string either. Im pretty sure that your WordCount routine might be an infinite loop situation.
– trumpetlicks
Feb 1 '13 at 19:29