(Scala) error handling
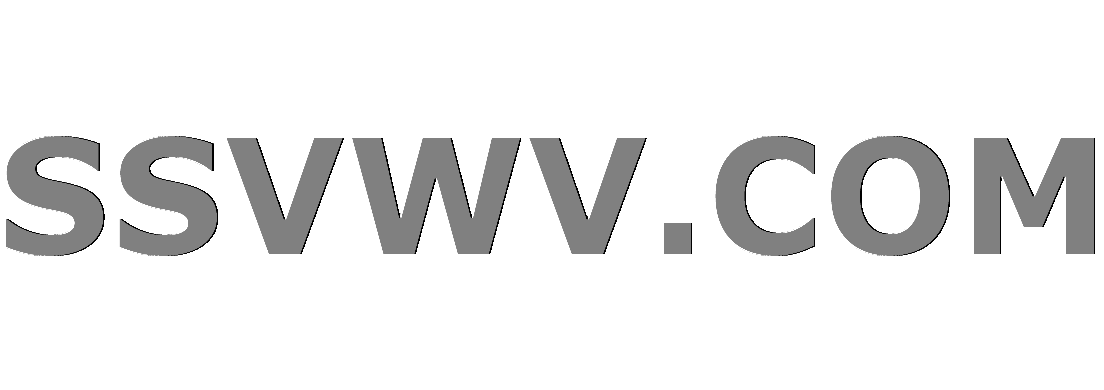
Multi tool use
(Scala) error handling
I'm currently making a function that returns an object. But If i find something invalid in the input I want to return something to indicate that problem. I just can not wrap my head around on working this out.. What is the correct way to handle errors here? Using Options? But returning None does not specify any specific problems. Am i getting something wrong?:)
case class myClass(value: Int)
def myFunction(input: Int) : myClass
{
//return myClass if succeded
//else return some error - what do I return here?
}
//I've read that to handle some error scenarios you should do something like this:
sealed trait SomeError
object SomeError{
case object UnknownValue extends SomeError
case object SomeOtherError extends SomeError
case object OutOfBounds extends SomeError
}
//But how do I return myClass and this at the same time in case of an error?
Thank you so much!! ^^
1 Answer
1
It's true, Option
can't give you details about your error. For that you can use Either
which is more flexible. Either
is extended by Left
and Right
. On success, one returns a Right
, on failure a Left
(by convention).
Option
Either
Either
Left
Right
Right
Left
e.g.
sealed trait MyTrait
case class MyClass(value: Int) extends MyTrait
def myFunction(input: Int): Either[String, MyTrait] = {
if (input >= 0) Right(MyClass(input)) else Left("input was negative")
}
Or using SomeError
,
SomeError
def myFunction(input: Int): Either[SomeError, MyTrait] = {
if (input >= 0) Right(MyClass(input)) else Left(OutOfBounds)
}
@JoeDortman No.
Either
is right-biased, so you should use Right
for the "correct" results, and Left
for "improvement proposals".– Andrey Tyukin
Jul 2 at 12:47
Either
Right
Left
you can remember it with
Right
is "right"– Joel Berkeley
Jul 2 at 12:48
Right
Thanks! Helped me a lot here! ^^
– JoeDortman
Jul 2 at 12:50
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Oh okay! So using Either should, in this case, use 'myClass'(left) and 'SomeError'(right)? ^^
– JoeDortman
Jul 2 at 12:45