Javascript Promise.all not show last
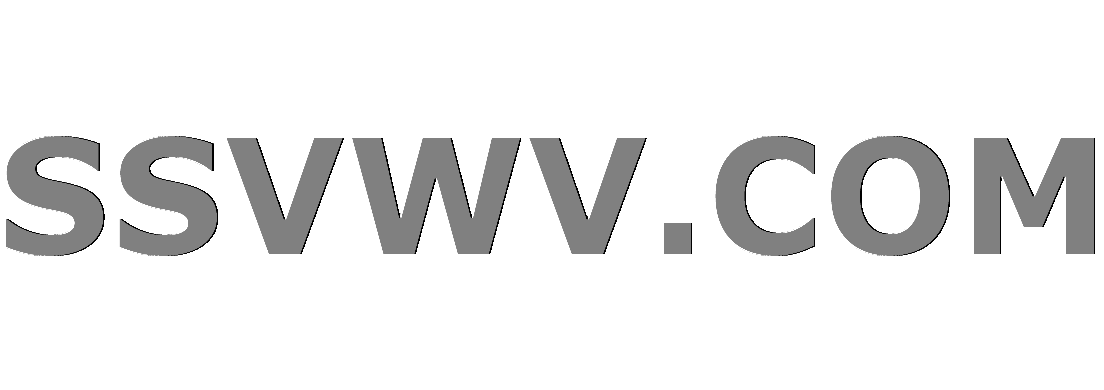
Multi tool use
Javascript Promise.all not show last
I have a few functions which return a promise.
There's a start function which executes another function.
As these are promises and I'm using Promise.all, in theory, Promise.all
should show only after all the other promises are completed.
Promise.all
Here is the code:
arr = ;
function start() {
var aPromiseMain = new Promise((resolve, reject) => {
myfunction();
arr.push('start');
resolve(console.log('I am start'))
})
return aPromiseMain
}
start();
function myfunction() {
var aPromise = new Promise((resolve, reject) => {
setTimeout(() => {
arr.push('I am myfunction');
resolve(console.log('I am myfunction'))
}, 2000)
})
return aPromise
}
Promise.all(arr).then(values => {
console.log(values);
});
What's happening instead is the order is as follows:
myfunction
It should be:
myfunction
How can I fix this?
The problem is that if I add the string into resolve('I am start'); I can only pass one, so if in the same function I wanted to pass 2 different values in different part of the code I would get issues
– Paul B
Jul 2 at 13:50
No no; the returned Promise instances are all ignored. Those are the things that need to go into the array, not strings. It has nothing to do with what you pass to
resolve()
.– Pointy
Jul 2 at 13:50
resolve()
your implementation is not correct. Promise.all is where you pass an array of promises. Instead, you are passing an array of string. You don't have to use Promise.all for your current implementation. If you want to do chaining of promises, then you can use Promise.all.
– Ajay Suvarna
Jul 2 at 13:53
So basically you are just doing
Promise.all([1,2,3]).then(console.log)
– palaѕн
Jul 2 at 13:57
Promise.all([1,2,3]).then(console.log)
4 Answers
4
From the author's description and comments, it looks like they might need to run the promises in series, so here is an alternative:
arr = ;
function start() {
console.log("I have started");
var aPromiseMain = new Promise((resolve, reject) => {
myfunction().then((result) => {
console.log('I am finished');
});
})
return aPromiseMain
}
function myfunction() {
var aPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve(console.log('I am myfunction'))
}, 2000)
})
return aPromise
}
start();
Your array should be an array of promises, not an array of strings.
arr = ;
function start() {
var aPromiseMain = new Promise((resolve, reject) => {
resolve('I am start')
})
return aPromiseMain
}
function myfunction() {
var aPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('I am myfunction')
}, 2000)
})
return aPromise
}
arr.push(start());
arr.push(myfunction());
Promise.all(arr).then(values => {
console.log(values);
console.log("I am done");
});
Well
myfunction()
is called from inside the start()
function, so that's where the promise would be pushed onto the array.– Pointy
Jul 2 at 13:51
myfunction()
start()
the myfunction() promise gets executed twice here, although it's only logged once
– ADyson
Jul 2 at 13:53
There is some confusion: you are using
promise.all
to execute the promises in parallel, but from your description, it sounds like you need to use a_promise.then()
to run them in series. Which one do you need?– ouni
Jul 2 at 13:54
promise.all
a_promise.then()
Yes myfunction() is run twice
– Paul B
Jul 2 at 13:57
Removed the extra myfunction call, thanks.
– ouni
Jul 2 at 13:59
Your code arr.push('start');
(for example) simply adds a string to the array. Promise.all needs access to the actual Promise objects, not strings. It cannot do anything useful with strings. Also sending the result of console.log
as the value to the resolve
functions makes no sense - the idea of this parameter is to give the listener some data back as a result - console.log logs things, but it doesn't return data.
arr.push('start');
console.log
resolve
This example pushes the Promise objects into the array so that Promise.all will wait for each to complete before executing, and also sends a meaningful response when the Promise resolves.
arr = ;
function start() {
var aPromiseMain = new Promise((resolve, reject) => {
console.log("start");
myfunction();
resolve('I am start');
});
arr.push(aPromiseMain);
return;
}
start();
function myfunction() {
var aPromise = new Promise((resolve, reject) => {
setTimeout(() => {
console.log("myfunction");
resolve('I am myfunction');
}, 2000)
})
arr.push(aPromise);
return;
}
Promise.all(arr).then(values => {
console.log(values);
});
function start() {
return new Promise((resolve, reject) => {
myfunction();
arr.push('start');
resolve(console.log('I am start'))
})
}
function myfunction() {
return new Promise((resolve, reject) => {
setTimeout(() => {
arr.push('I am myfunction');
resolve(console.log('I am myfunction'))
}, 2000)
})
}
var arr = [start(), myfunction()]
Promise.all(arr).then(values => {
console.log(values);
});
Try that way.
why did you not remove the unnecessary pushing of strings into the array?
– ADyson
Jul 2 at 13:54
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You're never putting any of those Promise values into the array; you're just pushing strings.
– Pointy
Jul 2 at 13:46