Calling a function inside render function - ReactJS?
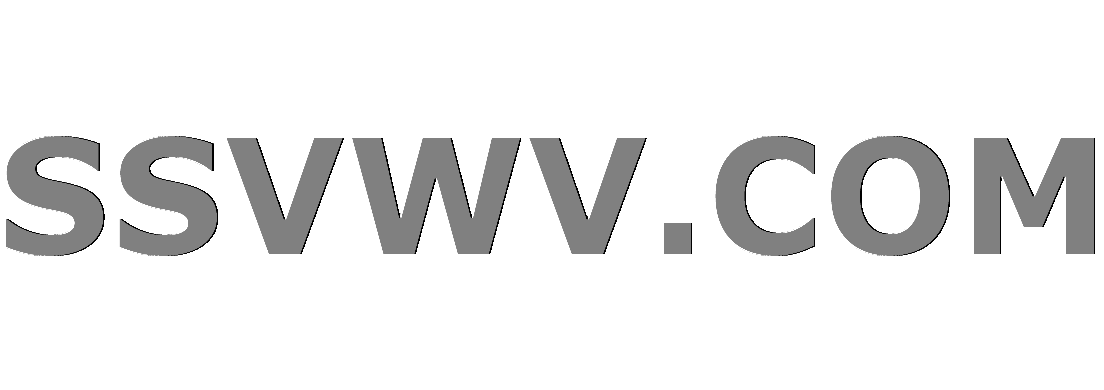
Multi tool use
Calling a function inside render function - ReactJS?
Ok so I am making a "buzzfeed" quiz generator and I am having an issue with a piece of my form. So for each question made there are two different parts, a "Question" and the "Answers". There will always be 4 answers but I want to keep track of which Answers are checked later so I would like to give each answer a unique id "question" + questionNum + "Answer" + answerNum
or "question" + questionNum + "Answer" + i
So I made this answersGenerator
function that is supposed to give me 4 unique answer choice fill ins for the user to input answers for that specific questions (right now it says something about terms and conditions - ignore that I ad just left it like that for now because I was following a tutorial). Here is all my code:
"question" + questionNum + "Answer" + answerNum
"question" + questionNum + "Answer" + i
answersGenerator
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import logo from './logo.svg';
import './App.css';
var questionNum = 1;
var answerNum = 1;
class App extends Component {
constructor(props) {
super(props);
this.state = {
quizTitle: "",
author: "",
question: "",
terms: false,
test: "",
};
this.handleChange = this.handleChange.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
}
handleChange(event) {
const target = event.target;
const value = target.type === "checkbox" ? target.checked : target.value;
const name = target.name;
this.setState({
[name]: value
});
}
handleSubmit(event) {
event.preventDefault();
console.log(this.state);
}
render() {
return (

Welcome to React
To get started, edit src/App.js
and save to reload.
Give your quiz a title.
</div>
Who's the Author?
</div>
Question {questionNum}
</div>
Answers
{this.answersGenerator()}
</div>
</div>
</div>
</div>
Add Question
</div>
</form>
</div>
Quiz Title: {this.state.quizTitle}
Author: {this.state.author}
Question: {this.state.question}
Terms and Condition: {this.state.terms}
Do you test?: {this.state.test}
</div>
</div>
</div>
);
}
}
function answersGenerator () {
console.log("hello this is answer generator");
var div = document.createElement('div');
div.className = 'answers' + {questionNum};
for (var i = 1; i < 5; i++){
div.innerHTML =
'
';
document.getElementsByClassName('answersDiv')[0].appendChild(div)
}
}
function addQuestion () {
questionNum++;
console.log(questionNum + "that is the question number");
var div = document.createElement('div');
div.className = "questions" + questionNum;
div.innerHTML =
'
Question '+{questionNum}+'
Answers
Check the box(es) for the correct answer(s).
';
document.getElementsByClassName('questions')[0].appendChild(div)
}
export default App;
There's a few things to just ignore in here, i just wasn't sure how much info you needed. So the issue is that I get an error when I call my answersGenerator
function in my render
function. Here's the error Uncaught TypeError: this.answersGenerator is not a function
Here's the small piece where that is
answersGenerator
render
Uncaught TypeError: this.answersGenerator is not a function
Answers
{this.answersGenerator()}
</div>
</div>
I appreciate the help, if you have any other tips for me other than this issue I am having I am all ears. I am very new at this, being js and React.
UPDATE: I have edited the answersDiv
to be just this (I relized it was being called inside a checkbox)
answersDiv
Answers
{answersGenerator()}
I am still getting the same errors though.
What's the error?
– Colin
Jul 2 at 20:21
Sorry about that, I added the error
– dorkycam
Jul 2 at 20:26
2 Answers
2
You're receiving the error because the answersGenerator()
isn't a method of your class App
, so, you can't refer to it using this
. If you want to do on that way, move you function to inside the component, like this:
answersGenerator()
App
this
class App extends Component {
// your component definition etc..
answersGenerator = () => {
// your function implementation
}
// the rest of your component
}
TIP:
If you dont want to have to always bind the function, like:
this.handleChange = this.handleChange.bind(this);
Declare your method like:
handleChange = (event) => { // the implementation }
Instead of
handleChange(event) { // the implementation }
The () => {}
, known as arrow function
, does the bind for your.
() => {}
arrow function
EDIT
You're developing with React, that supports creating HTML
inside your code. So, you can create an array of answers, then, return a div
with the answers mapped inside. The map
will take each answer
and render inside the div
. Try to define your method as below:
HTML
div
map
answer
div
answersGenerator => () {
console.log("hello this is answer generator");
var answers =
for (var i = 1; i < 5; i++){
answers.push(
);
}
return {answers.map(answer => answer)}
}
Ok so I took the answersGenerator
function off the bottom there and added it to the App
thing before the render
function like this: (next Comment)
– dorkycam
Jul 2 at 20:38
answersGenerator
App
render
answersGenerator = () => { // the stuff the function does }
– dorkycam
Jul 2 at 20:39
answersGenerator = () => { // the stuff the function does }
And then called it in the render function like this {this.answersGenerator()}
but I still get this error Uncaught TypeError: Cannot read property 'appendChild' of undefined
– dorkycam
Jul 2 at 20:40
{this.answersGenerator()}
Uncaught TypeError: Cannot read property 'appendChild' of undefined
That's because document.getElementsByClassName
doesn't work inside ReactJS. Take a look at this question
– Matheus Reis
Jul 2 at 20:43
document.getElementsByClassName
Ah, ok. I've looked over the question linked but I'm not familiar with how ReactDOM.findDOMNode(component)
works
– dorkycam
Jul 2 at 20:52
ReactDOM.findDOMNode(component)
At first glance it looks like you haven't defined your answersGenerator()
function within your App
class.
answersGenerator()
App
Thus, this.answersGenerator()
doesn't exist.
this.answersGenerator()
Try just using answersGenerator()
without the this
.
answersGenerator()
this
I tried it without the "this" and this is the error I'm getting now Uncaught TypeError: Cannot read property 'appendChild' of undefined
– dorkycam
Jul 2 at 20:28
Uncaught TypeError: Cannot read property 'appendChild' of undefined
Yep, you've a number of errors in your code.
– Colin
Jul 2 at 20:31
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
wL8V,RyCUE4JvM3FIXDfiDyrhtTp6JXxMgMJN45,aX Ohypg,oJ9vN,r,oWv
Please, add the error to your question
– Matheus Reis
Jul 2 at 20:19