D3js Multi line graph convert from using CSV file
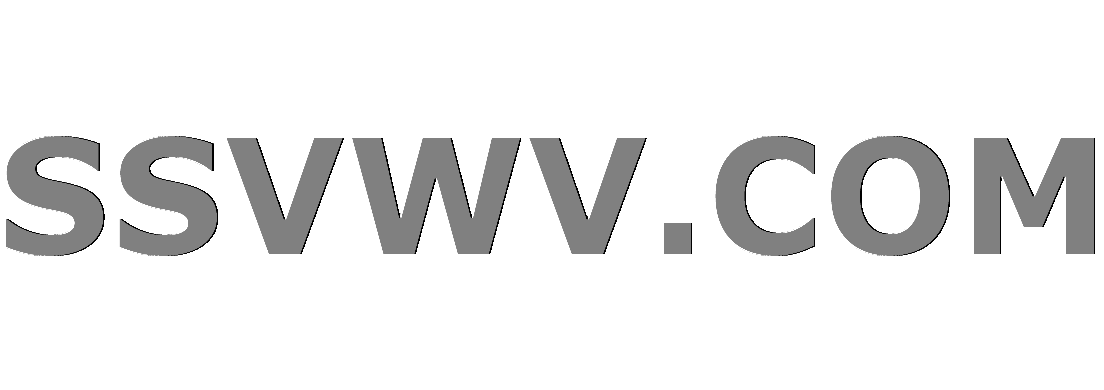
Multi tool use
D3js Multi line graph convert from using CSV file
I am looking to make a multi line graph from this example.
Instead of using data from a CSV file I'm building an array of values from the database:
$token_prices = sw::shared()->prices->getForTokenID($token_id);
$token_prices_array = array();
foreach ($token_prices as $token_price) {
$token_prices_array = [
"date" => $token_price['date'],
"close" => $token_price['close']
];
}
$second_token_prices = sw::shared()->prices->getForTokenID(3);
$second_token_prices_array = array();
foreach ($second_token_prices as $second_token_price) {
$second_token_prices_array = [
"date" => $second_token_price['date'],
"close" => $second_token_price['close']
];
}
$all = array_merge($second_token_prices_array, $token_prices_array);
foreach ($all as $datapoint) {
$result[$datapoint['date']] = $datapoint['close'];
}
Data output:
{"15-Jun-18":["8.4","0.14559"],"16-Jun-18":["8.36","0.147207"],"17-Jun-18":["8.42","0.13422"],"18-Jun-18":["8.71","0.146177"],"19-Jun-18":["8.62","0.138188"],"20-Jun-18":["8.45","0.128201"],
My issue is with plugging the data from the database in:
var tokendata = <?php echo json_encode($result) ?>;
data = tokendata;
data.forEach(function(d) {
d.date = parseTime(d.date);
d.close = +d.close;
d.open = +d.open;
});
I get an issue here "data.forEach is not a function"...
How can I fix this to use the data from the database?
Here is the Fiddle
1 Answer
1
It looks like you are embedding the results of the query php page as a JSON string -- if you want to iterate over that data as an array, you will have to parse it back into a Javascript object first.
I'm assuming that the first code snippet is running on a different server, and so the $result
array is not directly available to your javascript code -- is this why you are trying to set a variable to the encoded return value? If so, it's not the best way to pull data into your page's script, but this may work for you:
$result
var data = JSON.parse('<?php echo json_encode($result)?>');
or even:
var data = eval(<?php echo json_encode($result)?>);
Both methods assume that your result is returned as a valid json string, since there is no error checking or try/catch logic. I honestly don't know what the output of the json_encode()
method looks like, so if you still can't get it working, please update your post with an example of the returned string.
json_encode()
Looks like you don't need the double quotes around the php output string...
– SteveR
Jul 2 at 19:01
Unfortunately, I get a different error now: Unexpected token o in JSON at position 1 at JSON.parse. I've updated the original post to show how the array is structured/output
– joeshmoe
Jul 2 at 19:57
Thanks for the update -- much easier to see the data now. Since your output json is using double quotes for keys and string values, I would try using single quotes around the
<?php ...?>
substitution (as I've done in the updated example). That may still fail if you have any single quotes inside the data -- in which case it looks like you will need to use the eval(...)
function.– SteveR
Jul 2 at 20:02
<?php ...?>
eval(...)
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Hi thanks for your input - I get this error: Uncaught SyntaxError: missing ) after argument list ..... heres output: var data = JSON.parse("{"15-Jun-18":["8.4","0.14559"],"16-Jun-18":["8.36","0.147207"]}");
– joeshmoe
Jul 2 at 18:50