Remove text after answer has been made with jQuery/JavaScript
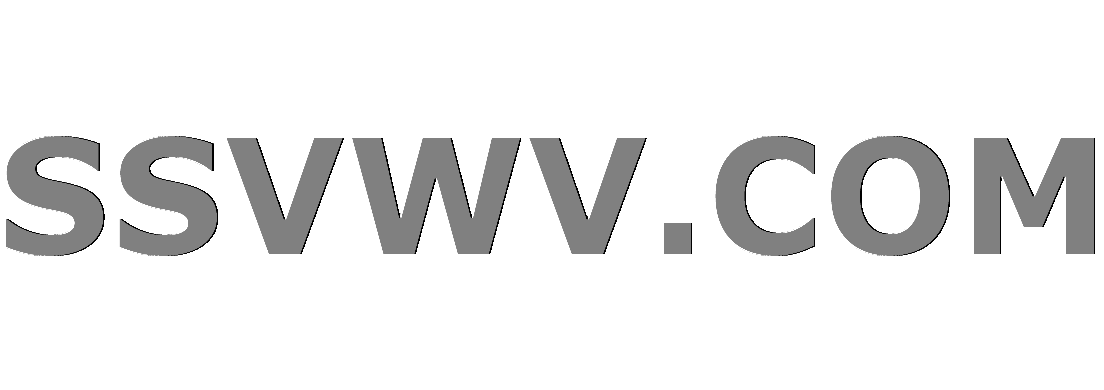
Multi tool use
Remove text after answer has been made with jQuery/JavaScript
I've run into an issue with my logic I think. What should happen is, the user will enter an answer to the presented question. Once the question has been submitted, the current text (first question) disappears, and the next question in the array appears in place of the first question.
Currently the questions continue to pile on to one another without removing the previous question. Every time I try and add more code to remove the element, it gives me an error.
Please help! I am slowly adding to this codes functionality but I am stuck now on this add/remove text thing.
$(function() {
$("#submit").on("click", askQuestion)
var questions = [{
question: "Is the price higher or lower than $40.00?",
answer: "higher"
},
{
question: "Is the price higher or lower than $100.00?",
answer: "higher"
},
{
question: "Is the price higher or lower than $50.00?",
answer: "lower"
}
];
function askQuestion() {
if (answer && document.querySelector("#user-answer").value == answer) {
document.querySelector("#correct").style.display = "block";
document.querySelector("#sorry").style.display = "none";
answer = randomQuestion()
} else {
document.querySelector("#correct").style.display = "none";
document.querySelector("#sorry").style.display = "block";
}
}
function randomQuestion() {
var question = questions[Math.floor(Math.random() * questions.length)];
document.querySelector("#user-answer").value = "";
var element = document.createElement("div");
element.innerHTML = question.question;
$("#question").append(element.firstChild);
return question.answer;
}
var answer = randomQuestion();
});
<html>
<head>
<meta charset="UTF-8">
<title>Game time</title>
</head>
<body>
<h1>Question and Answer</h1>
<label for="text">Answer:</label>
<input id="user-answer" type="text" value="">
<button id="submit" type="submit">Submit</button>
<p id="sorry" style="display: none">Sorry...</p>
<p id="correct" style="display: none">You got it!</p>
https://code.jquery.com/jquery-3.3.1.min.js
http://main.js
</body>
</html>
stackoverflow.com/questions/1675215/…
– fstep
Jul 2 at 19:53
2 Answers
2
You're simply using append
when you should be changing the question, not appending to it.
append
You can use:
$("#question").html(element.firstChild);
$("#question").html(element.firstChild);
instead of $("#question").append(element.firstChild);
$("#question").append(element.firstChild);
$(function() {
$("#submit").on("click", askQuestion)
var questions = [{
question: "Is the price higher or lower than $40.00?",
answer: "higher"
},
{
question: "Is the price higher or lower than $100.00?",
answer: "higher"
},
{
question: "Is the price higher or lower than $50.00?",
answer: "lower"
}
];
function askQuestion() {
if (answer && document.querySelector("#user-answer").value == answer) {
document.querySelector("#correct").style.display = "block";
document.querySelector("#sorry").style.display = "none";
answer = randomQuestion()
} else {
document.querySelector("#correct").style.display = "none";
document.querySelector("#sorry").style.display = "block";
}
}
function randomQuestion() {
var question = questions[Math.floor(Math.random() * questions.length)];
document.querySelector("#user-answer").value = "";
var element = document.createElement("div");
element.innerHTML = question.question;
$("#question").html(element.firstChild);
return question.answer;
}
var answer = randomQuestion();
});
<html>
<head>
<meta charset="UTF-8">
<title>Game time</title>
</head>
<body>
<h1>Question and Answer</h1>
<label for="text">Answer:</label>
<input id="user-answer" type="text" value="">
<button id="submit" type="submit">Submit</button>
<p id="sorry" style="display: none">Sorry...</p>
<p id="correct" style="display: none">You got it!</p>
https://code.jquery.com/jquery-3.3.1.min.js
http://main.js
</body>
</html>
You're a life saver. I almost cried literally. I've been doing this for a few days on my down time at work and I've been stuck for longer than I'd like to admit. I can't believe this was the only issue.
– Nick0989
Jul 2 at 19:58
@Nick0989 no worries! glad I could help, we've all been there.
– zfrisch
Jul 2 at 19:59
You are using the append method. If you want to completly override the text, you could use the .text (jQuery) method or .innerText (Vanilla js)
That won't work, you're interpreting a node.
html
works, text
and innerText
don't because they turn it into a string "[object Object]"– zfrisch
Jul 2 at 19:57
html
text
innerText
You get a node if you use a selector that returns a group of elements. If you use document.getElementById or document.querySelector you will get that specific element, and the the .innerText methods will be available. About the jQuery .text method I tried and worked fine. $('#question').text('a'); Maybe I just didn't explain right.
– Joao Paulo
Jul 2 at 21:50
Sure, I get it, but that's outside the scope of the answer you provided. The code
$("#question").append(element.firstChild);
is what needs to be changed in the above code, but your answer doesn't say anything about changing the input, only what method is being used. For example if you change the code to $("#question").text(element.firstChild);
it will display "[object Object]" because element.firstChild
is an object, not text.– zfrisch
Jul 2 at 21:58
$("#question").append(element.firstChild);
$("#question").text(element.firstChild);
element.firstChild
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
what is the error?
– mjw
Jul 2 at 19:50