How do I perform an insecure URLSession query in iOS
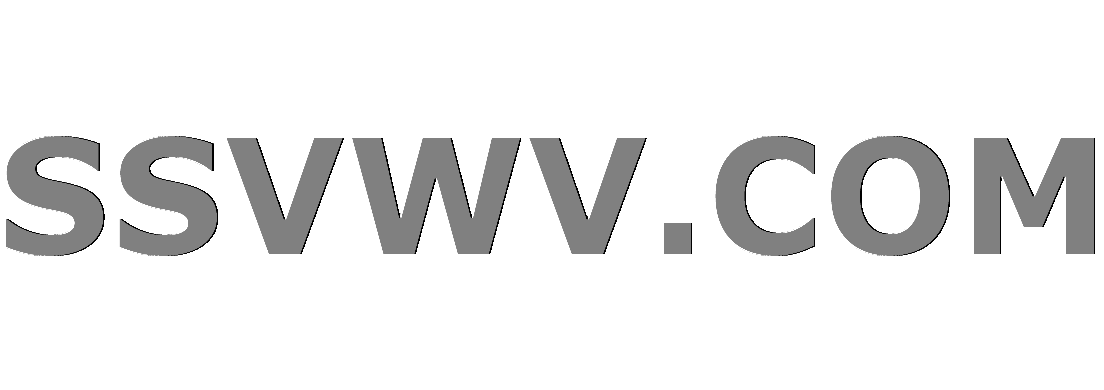
Multi tool use
How do I perform an insecure URLSession query in iOS
I'm trying to perform a query to a website that I run. However, currently, this website's certificate is invalid (for a valid reason for now).
I'm trying to query it with this code:
private static func performQuery(_ urlString: String) {
guard let url = URL(string: urlString) else {
return
}
print(url)
URLSession.shared.dataTask(with: url) {
(data, response, error) in
if error != nil {
print(error!.localizedDescription)
}
guard let data = data else {
return
}
do {
let productDetails = try JSONDecoder().decode([ProductDetails].self, from: data)
DispatchQueue.main.async {
print(productDetails)
}
} catch let jsonError {
print(jsonError)
}
}.resume()
}
However, I get:
NSURLSession/NSURLConnection HTTP load failed (kCFStreamErrorDomainSSL, -9813)
The certificate for this server is invalid. You might be connecting to a server that is pretending to be “mydomain.com” which could put your confidential information at risk.
How can I make an insecure URLSession query (equivalent to -k in CURL)?
I've tried setting these:
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
<key>NSExceptionDomains</key>
<dict>
<key>mydomain.com</key>
<dict>
<key>NSExceptionAllowsInsecureHTTPLoads</key>
<true/>
<key>NSIncludesSubdomains</key>
<true/>
<key>NSTemporaryExceptionRequiresForwardSecrecy</key>
<false/>
<key>NSThirdPartyExceptionAllowsInsecureHTTPLoads</key>
<true/>
</dict>
</dict>
</dict>
And yes I don't intend to release this to the App Store with insecure access, but I need to get the code tested and right now we can't get a valid certificate, so this is purely for development purposes.
2 Answers
2
First, set the delegate of the session to your class which conforms to URLSessionDelegate
like :
URLSessionDelegate
let session = URLSession(configuration: .default, delegate: self, delegateQueue: OperationQueue.main)
Add the implementation of didReceiveChallenge
method in your class which conforms to URLSessionDelegate
protocol
didReceiveChallenge
URLSessionDelegate
public func urlSession(_ session: URLSession, didReceive challenge: URLAuthenticationChallenge, completionHandler: @escaping (URLSession.AuthChallengeDisposition, URLCredential?) -> Void) {
completionHandler(.useCredential, URLCredential(trust: challenge.protectionSpace.serverTrust!))
}
This will allow insecure connection by trusting the server.
WARNING : DO NOT use this code in production apps, this is a potential security risk.
@Wayneio create a URLSession object with default session configuration and set it's delegate to your class. Use
init(configuration:delegate:delegateQueue:)
– Shaggy D
Jul 3 at 13:00
init(configuration:delegate:delegateQueue:)
@Wayneio edited my answer
– Shaggy D
Jul 3 at 13:05
Okay cool. So then to use it, I try let _ = session.shared.dataTask(with: request as URLRequest) {... but it say session cannot be used on type 'Util' (Util is my class name)
– Wayneio
Jul 3 at 13:07
Generally all your request code should be in a singleton class which has a session. For example, the class can be named as NetworkManager and it will have an instance of URLSession. You shouldn't perform requests from static methods of Utils.
– Shaggy D
Jul 3 at 13:11
Try these exceptions:
<key>NSExceptionMinimumTLSVersion</key>
<string>TLSv1.0</string>
<key>NSExceptionRequiresForwardSecrecy</key>
<false/>
<key>NSExceptionAllowsInsecureHTTPLoads</key>
<true/>
<key>NSIncludesSubdomains</key>
<true/>
Thanks, this returns the same error
– Wayneio
Jul 2 at 12:25
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
URLSession.shared.delegate = self doesn't work because delegate is get only
– Wayneio
Jul 3 at 12:52