Importing Java Date Class
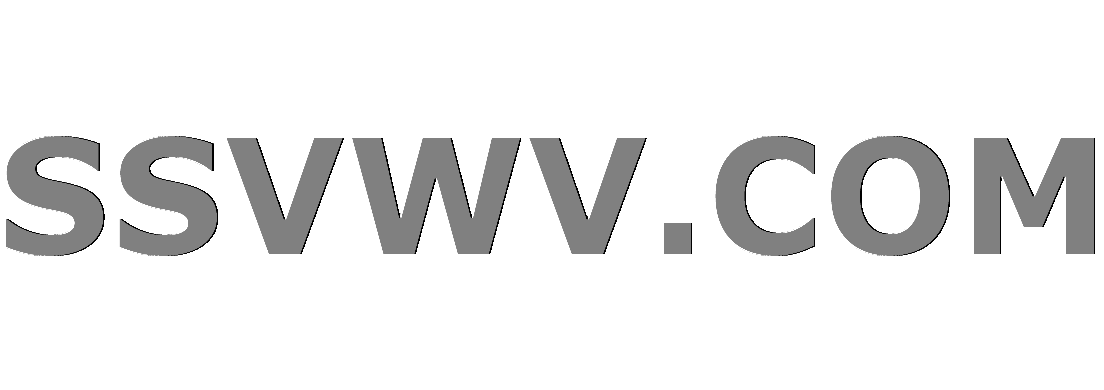
Multi tool use
Importing Java Date Class
I had to edit original question, because I think many authors misunderstand it.
I have the most basic problem. Try to get the system date. My code is this;
import java.util.Date;
public class DateDemo {
public static void main(String args) {
// Instantiate a Date object
Date date = new Date();
// display time and date using toString()
System.out.println(date.toString());
}
}
It gives me the error: Syntax error on token "import", assert expected
The solution should be very basic but I couldn't find it.
Thanks for your help.
Google 'java package statement'
– bphilipnyc
Jul 3 '16 at 18:11
Read the Oracle Java Tutorial.
– Basil Bourque
Jul 3 '16 at 18:25
I'm voting to close this question as off-topic because the author has not yet learned the most minimum basics of "Hello World" Java programming (classes and
main
method).– Basil Bourque
Jul 3 '16 at 18:27
main
By the way, the modern way to do this date-time work is:
java.time.Instant.now().toString()
– Basil Bourque
Sep 2 '17 at 18:26
java.time.Instant.now().toString()
3 Answers
3
You try to do something impossible. You should create a class and in a method in this class, you should make these operations. In the example below, you make these operations in a main method in your class. Main method is where the program starts.
In addition to these points, if your class is in a package, you should define it at the very beginning of the class. For example, your package is called as myPackage
, you should add it to your code like that below;
myPackage
For example, you creates a class Foo
in a package myPackage
.
Foo
myPackage
package myPackage;
import java.util.Date;
public class Foo {
public static void main(String args) {
// You create a new date object and assign it to the sysdate
Date sysdate = new Date();
// You are now displaying your time and date using toString()
System.out.println(sysdate.toString());
}
}
Thanks for your help I see that my code was literaly stupid but still when I try your code it gives me the same error. Since I am a begginner I couldn't get what is wrong with it.
– kutayatesoglu
Jul 3 '16 at 18:25
we all made the same mistakes. It is not stupid at all. Sen canını sıkma ;) Can you please copy the whole program here ?
– Ad Infinitum
Jul 3 '16 at 18:27
The whole program is that, as I said, I am a begginner. I am only learning the basics of java. As some authors mentioned before I am only learning the fundamentals of java.
– kutayatesoglu
Jul 3 '16 at 18:38
I have copy the code above and it runs on my local machine. You may write the whole error. With that, I could help better.
– Ad Infinitum
Jul 3 '16 at 18:43
I have editted my answer. Please pay attention to the package info above.
– Ad Infinitum
Jul 3 '16 at 18:47
The instructions in Java can't be outside classes. So your instructions
Date sysdate = new Date();
System.out.println(sysdate);
Should be in a method (For example, in the main
), and the method must be declared in a class. As far as I know, import
and package
instructions are the only ones which have to be before the class definition.
main
import
package
Note also that the file where the class is written must have the same name of the class, for instance, the example DateDemo
must be save in a file called DateDemo.java
.
DateDemo
DateDemo.java
Thanks, I changed my code according to what you wrote but still I had the same problem. The code above fits all what you have mentioned. The file is named DateDemo.java and the instructions are in a method which declared in a class. But the error says that it expect "assert" which I dont know what it is.
– kutayatesoglu
Jul 3 '16 at 18:49
The other Answers are correct about you not including proper class structure with main method.
Never use Date
Date
Also, never use the Date
class. It is terribly designed, and quite confusing and troublesome. Now legacy, supplanted by the java.time.Instant
class.
Date
java.time.Instant
java.time
To represent a moment in UTC, use Instant
. That class uses a resolution as fine as nanoseconds. The current moment may be captured with only milliseconds or microseconds depending on the limitations of your host computer clock hardware, your host operating system, and your Java Virtual Machine implementation.
Instant
Example code.
package com.basilbourque.example.telltimeapp ;
import java.time.Instant ;
public class TellTime {
public static void main( String args )
{
Instant instant = Instant.now() ; // Capture the current moment in UTC.
String output = instant.toString() ; // Generate text in a `String` object representing the value of the `Instant` object in standard ISO 8601 format.
System.out.println( output ) ; // Dump text to the console.
}
}
Lets get more realistic. Launch an instance of our app in the main
method. Then invoke a method on that app object to get the ball rolling.
main
Our app knows how to do two things:
America/Montreal
Our main
method asks for the first of those two.
main
package com.basilbourque.example.telltimeapp ;
import java.time.Instant ;
public class TellTime {
public static void main( String args ) // The `main` method is *not* object-oriented, just a hack, the way we solve the chicken-or-the-egg dilemma] of how to launch an OOP app.
{
TellTime app = new TellTime() ; // Create an instance of our app, an object that represents the entire running app.
app.tellCurrentMomentInUtc() ; // Ask our app object to run some code.
}
public void tellCurrentMomentInUtc()
{
Instant instant = Instant.now() ; // Capture the current moment in UTC.
String output = instant.toString() ; // Generate text in a `String` object representing the value of the `Instant` object in standard ISO 8601 format.
System.out.println( output ) ; // Dump text to the console. 2018-07-02T20:17:49.929677Z
}
public void tellCurrentMomentInMontreal()
{
Instant instant = Instant.now() ; // Capture the current moment in UTC.
ZoneId z = ZoneId.of( "America/Montreal" ) ; // A time zone is a history of the past, present, and future changes to the offset-from-UTC used by the people of a particular region.
ZonedDateTime zdt = instant.atZone( z ) ; // Adjust from UTC to Québec time. Same moment, same point on the timeline, different wall-clock time.
DateTimeFormatter f = DateTimeFormatter.ofLocalizedDateTime( FormatStyle.FULL ).withLocale( Locale.CANADA_FRENCH ) ; // Be aware that time zone and locale have *nothing* to do with one another, completely orthogonal issues.
String output = zdt.format( f ) ; // Generate text in a localized format.
System.out.println( output ) ; // Dump text to the console. Example: lundi 2 juillet 2018 à 16:16:59 Eastern Daylight Time
}
}
Tutorials
Study the Java Tutorials by Oracle before posting to Stack Overflow.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
java.util.Date
Calendar
SimpleDateFormat
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
java.sql.*
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
Interval
YearWeek
YearQuarter
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Please pay more attention to the fundamentals of Java before you start doing those things.
– Jerrybibo
Jul 3 '16 at 18:10