Xamarin.Forms TapGestureRecognizer not working on IOS
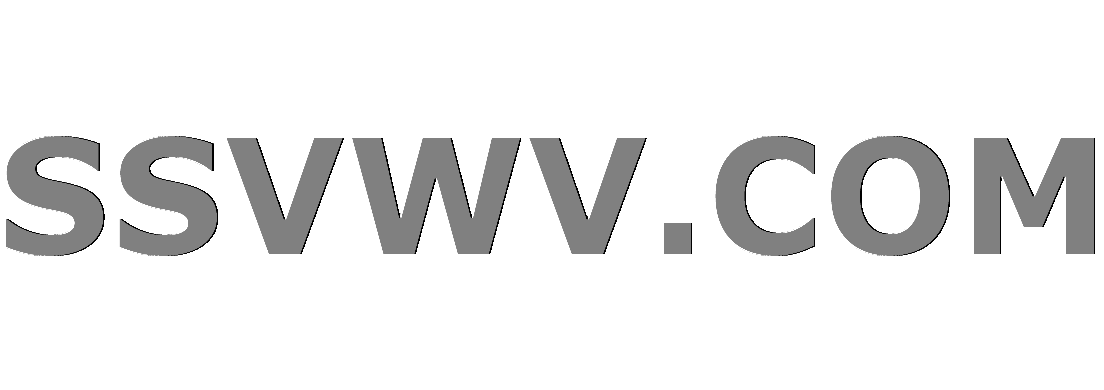
Multi tool use
Xamarin.Forms TapGestureRecognizer not working on IOS
I have a very simple Xamarin.Forms app. It's just for testing, and at the moment is just supposed to display messages when different boxes on the screen are tapped. The tap gesture isn't working though.
Here is my XAML:
<!-- Header -->
<Label Text="My Company"
TextColor="White"
FontSize="Large"
BackgroundColor="#a6192e"
HorizontalTextAlignment="Center" />
<!-- Body -->
<FlexLayout FlexLayout.Grow="1">
<!-- Content -->
<BoxView
BackgroundColor="#80225f"
FlexLayout.Grow="1" />
<Grid
BackgroundColor="#80225f"
Padding="20,50,50,20"
RowSpacing="20"
ColumnSpacing="20">
<Grid.RowDefinitions>
<RowDefinition Height="300"/>
<RowDefinition Height="300"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="280"/>
<ColumnDefinition Width="280"/>
<ColumnDefinition Width="280"/>
</Grid.ColumnDefinitions>
<BoxView
x:Name="App1Box"
BackgroundColor="Silver"
Grid.Row="0"
Grid.Column="0"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"/>
<BoxView
BackgroundColor="Gray"
Grid.Row="0"
Grid.Column="0"
HeightRequest="60"
VerticalOptions="Start"/>
<Label
Grid.Row="0"
Grid.Column="0"
HorizontalTextAlignment="Center"
TextColor="White"
FontSize="Large"
FontAttributes="Bold"
Margin="10">
App1</Label>
<BoxView
x:Name="App2Box"
BackgroundColor="Silver"
Grid.Row="0"
Grid.Column="1"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"/>
<BoxView
BackgroundColor="Gray"
Grid.Row="0"
Grid.Column="1"
HeightRequest="60"
VerticalOptions="Start"/>
<Label
Grid.Row="0"
Grid.Column="1"
HorizontalTextAlignment="Center"
TextColor="White"
FontSize="Large"
FontAttributes="Bold"
Margin="10">
App2</Label>
<BoxView
x:Name="App3Box"
BackgroundColor="Silver"
Grid.Row="0"
Grid.Column="2"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"/>
<BoxView
BackgroundColor="Gray"
Grid.Row="0"
Grid.Column="2"
HeightRequest="60"
VerticalOptions="Start"/>
<Label
Grid.Row="0"
Grid.Column="2"
HorizontalTextAlignment="Center"
TextColor="White"
FontSize="Large"
FontAttributes="Bold"
Margin="10">
App3</Label>
<Button Grid.Row="1"
Grid.Column="0"
Text="Test Tap"
Clicked="OnMealsTapped"
WidthRequest="100"
HeightRequest="100"
BackgroundColor="Lime"
TextColor="Red"/>
</Grid>
<!-- Navigation items-->
<BoxView FlexLayout.Basis="50"
FlexLayout.Order="-1"
Color="#80225f" />
<!-- Aside items -->
<BoxView FlexLayout.Basis="50"
Color="#80225f" />
</FlexLayout>
<!-- Footer -->
<Label Text="Test App"
FontSize="Large"
BackgroundColor="Gray"
HorizontalTextAlignment="Center" />
</FlexLayout>
</ContentPage.Content>
And here is my C#:
using System;
using System.Collections.Generic;
using Xamarin.Forms;
namespace TESTAPP
{
public partial class MyPage : ContentPage
{
public MyPage()
{
InitializeComponent();
var App1TapHandler = new TapGestureRecognizer();
var App2TapHandler = new TapGestureRecognizer();
var App3TapHandler = new TapGestureRecognizer();
App1TapHandler.NumberOfTapsRequired = 1;
App1TapHandler.Tapped += OnApp1Tapped;
App2TapHandler.NumberOfTapsRequired = 1;
App2TapHandler.Tapped += OnApp2Tapped;
App3TapHandler.NumberOfTapsRequired = 1;
App3TapHandler.Tapped += OnApp3Tapped;
App1Box.GestureRecognizers.Add(App1BoxTapHandler);
App2Box.GestureRecognizers.Add(App2BoxTapHandler);
App3Box.GestureRecognizers.Add(App3BoxTapHandler);
}
private void OnApp1Tapped(object sender, EventArgs e)
{
DisplayAlert("App1","Lets use App1","OK");
}
private void OnApp2Tapped(object sender, EventArgs e)
{
DisplayAlert("App2", "Lets use App2", "OK");
}
private void OnApp3Tapped(object sender, EventArgs e)
{
DisplayAlert("App3", "Lets use App3", "OK");
}
}
}
Button is there for testing. When tapping button I get the test alert message. Nothing happens when tapping any of the BoxViews.
Why is that not working?
2 Answers
2
Your problem isn't related to the TapGesture, but you have 2 Boxviews overlapping.
So you will have to add the InputTransparent
in the BoxViews that aren't receiving any input:
InputTransparent
<BoxView
x:Name="App1Box"
BackgroundColor="Silver"
Grid.Row="0"
Grid.Column="0"
HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"/>
<BoxView
InputTransparent="true" <!-- Here -->
BackgroundColor="Gray"
Grid.Row="0"
Grid.Column="0"
HeightRequest="60"
VerticalOptions="Start"/>
Repeat for the remaining.
Marking as answer - when you said "repeat for remaining" I didn't realise you meant remaining overlapping elements. Adding this to the Label as well solved the problem.
– Matt G
Jul 2 at 20:33
Try to invoke the code in the event handlers on the main thread.
Device.BeginInvokeOnMainThread(() =>
{
//Run the code here.
});
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This has definitely given me a point in the right direction...adding this didn't seem to fix it, I tried adding to either and then both BoxView. But when I remove the overlapping BoxView and Label, the tap works.
– Matt G
Jul 2 at 20:29