Adding new comboboxes Java
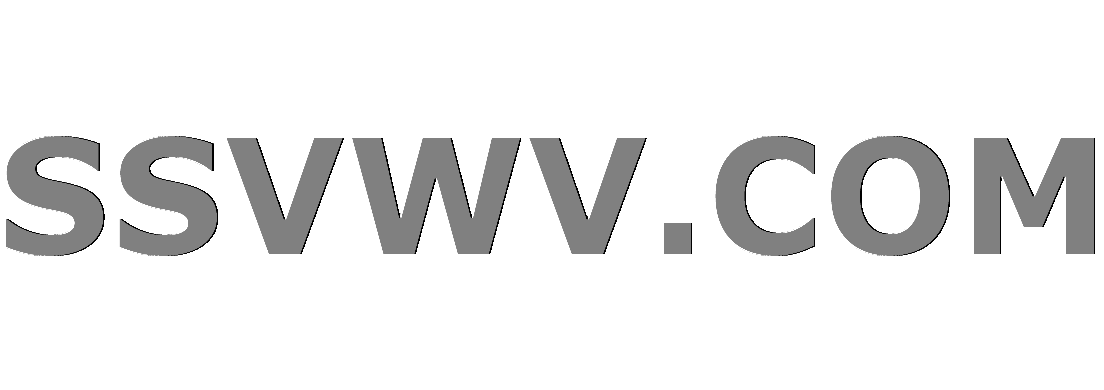
Multi tool use
Adding new comboboxes Java
I have a problem with Swing interface on java. Explaination: I have a Combobox with 1, 2, 3, 4, 5 items. When an exact item is selected I need to create some more comboboxes the number of which depends on the selected item. So, if number 5 is selected, 5 more comboboxes must appear in the frame. I used ActionListener but it did not work properly. However, the same code but outside Actionlistener works well. What a problem can it be?
public class FrameClass extends JFrame {
JPanel panel;
JComboBox box;
String s = {"1", "2", "3", "4", "5"};
String s1 = {"0", "1", "2", "3", "4", "5"};
public FrameClass() {
panel = new JPanel();
box = new JComboBox(s);
JComboBox adults = new JComboBox(s);
JComboBox children = new JComboBox(s1);
panel.add(box, BorderLayout.CENTER);
box.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
for(int i = 0; i <= box.getSelectedIndex(); i++) {
panel.add(adults, BorderLayout.WEST);
panel.add(children, BorderLayout.WEST);
}
}
});
add(panel);
}
}
public class MainClass {
public static void main(String args) {
JFrame frame = new FrameClass();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setExtendedState(Frame.MAXIMIZED_BOTH);
frame.getContentPane().setBackground(Color.WHITE);
frame.setVisible(true);
}
}
add a call to
panel.revalidate();
after adding the components– Michael
Jul 2 at 12:36
panel.revalidate();
1 Answer
1
The problem that you don't inform the layout manager about new elements in your panel.
Here is the correct variant of your action listener:
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.WindowConstants;
public class FrameClass extends JFrame {
JPanel panel;
JComboBox<String> box;
String s = {"1", "2", "3", "4", "5"};
String s1 = {"0", "1", "2", "3", "4", "5"};
public FrameClass() {
panel = new JPanel();
box = new JComboBox(s);
JComboBox adults = new JComboBox[5];
JComboBox children = new JComboBox[5];
for (int i = 0; i < 5; i++) {
adults[i] = new JComboBox<>(s);
children[i] = new JComboBox<>(s1);
}
panel.add(box, BorderLayout.CENTER);
JPanel nested = new JPanel();
add(nested, BorderLayout.EAST);
box.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
nested.removeAll();
nested.setLayout(new GridLayout(box.getSelectedIndex() + 1, 2));
for (int i = 0; i <= box.getSelectedIndex(); i++) {
nested.add(adults[i]);
nested.add(children[i]);
}
getContentPane().revalidate();
getContentPane().repaint();
pack();
}
});
add(panel);
}
public static void main(String args) {
JFrame frame = new FrameClass();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.pack();
frame.getContentPane().setBackground(Color.WHITE);
frame.setLocationRelativeTo(null); // center the window
frame.setVisible(true);
}
}
Thanks a lot. It works but new comboboxes appear only once even when the selected index is 4
– Yuriy_Puhlik
Jul 2 at 12:42
@Yuriy_Puhlik You cannot add one combo box twice. You need an array of combo boxes to add.
– Sergiy Medvynskyy
Jul 2 at 12:47
@Yuriy_Puhlik also you probably need another layout manager
– Sergiy Medvynskyy
Jul 2 at 12:51
@Yuriy_Puhlik I've changed my example. It adds now the different number of combo boxes, depending on the current selection in the main combo box.
– Sergiy Medvynskyy
Jul 2 at 13:06
Thank you very much. It works properly!
– Yuriy_Puhlik
Jul 2 at 13:30
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
All the same with SwingUtilities.invokeLater
– Yuriy_Puhlik
Jul 2 at 12:35