Defining an API with swagger: GET call that uses JSON in parameters
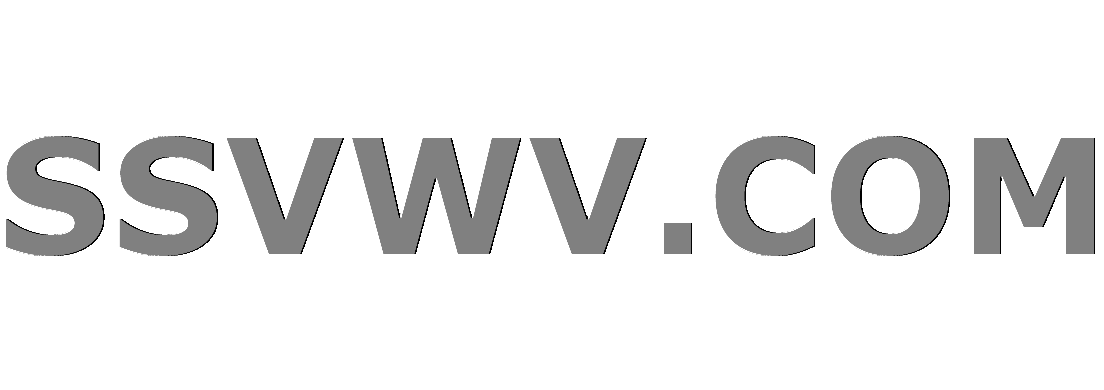
Multi tool use
Defining an API with swagger: GET call that uses JSON in parameters
I am trying to create a proper, REST API, and document it with Swagger (2.0).
So, I have an API call that is a query, ie, it makes no changes and doesn't create anything (idempotent and safe). But it requires passing in a complex JSON parameter (list of items, 2 or 3 sets of addresses, etc). So I'm doing a GET with a parameter thats URL encoded JSON. That seems like the proper way to do it.
I see so often API's like this where they do it as a POST for this reason, but that's an incorrect use of the POST verb.
I'm seeing lots of swagger API's that do this...
I can't figure out if there's a way to do a proper rest API with Swagger, using a JSON parameter. You can define the parameter as a string, of course, and pass your encoded JSON into it, but then the swagger tooling doesn't understand that there's a schema/definition for it.
Is swagger not able to properly document this kind of call?
2 Answers
2
I'm also working on defining form parameters which are URL-encoded JSON objects and facing the same situation as yours.
Thoroughly going through the Swagger specs will tell you that this is not supported yet, but something which is very much required for REST APIs.
Also, you can't define a schema for such an object.
OpenAPI 2.0 does not support objects in query strings, it only supports primitive values and arrays of primitives. The most you can do is define your parameter as type: string
, add an example
of a JSON value, and use description
to document the JSON object structure.
type: string
example
description
swagger: '2.0'
...
paths:
/something:
get:
parameters:
- in: query
name: params
required: true
description: A JSON object with the `id` and `name` properties
type: string
example: '{"id":4,"name":"foo"}'
JSON in query string can be described using OpenAPI 3.0. In OAS 3, query parameters can be primitives, arrays as well as objects, and you can specify how these parameters should be serialized – flattened into key=value
pairs, encoded as a JSON string, and so on.
key=value
For query parameters that contain a JSON string, use the content
keyword to define a schema
for the JSON data:
content
schema
openapi: 3.0.1
...
paths:
/something:
get:
parameters:
- in: query
name: params
required: true
# Parameter is an object that should be serialized as JSON
content:
application/json:
schema:
type: object
properties:
id:
type: integer
name:
type: string
This corresponds to the following GET request (before URL encoding):
GET /something?params={"id:4,"name":"foo"}
or after URL encoding:
GET /something?params=%7B%22id%3A4%2C%22name%22%3A%22foo%22%7D
Note for Swagger UI users: As of July 2018 Swagger UI does not support parameter that use content
, see issue 4442.
content
If you need "try it out" support, the workaround is to define the parameter as just type: string
and add an example
of the JSON data. You lose the ability to describe the JSON schema for the query string, but "try it out" will work.
type: string
example
parameters:
- in: query
name: params
required: true
schema:
type: string # <-------
example: '{"id":4,"name":"foo"}' # <-------
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.