Python Falcon microservice that uses microservice?
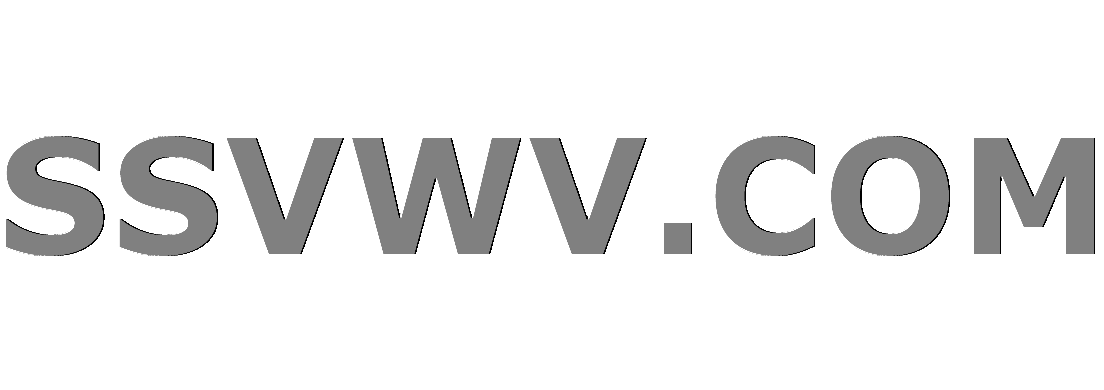
Multi tool use
Python Falcon microservice that uses microservice?
I'm just getting started with Falcon and I'm trying to do something that I think should be very simple and basic. I simply want one of my services to send a request to another one.
Let me make it even more simple with a tiny code example. Here are two microservices. Service #1 stores a number, and service #2 increments a stored number.
class NumberResource:
def on_post(self, req, resp):
self.value = req.media.get('value')
resp.media = {'value': self.value}
def on_get(self, req, resp):
resp.media = {'value': self.value}
class NextNumberResource:
def on_get(self, req, resp):
sibling = {}
# TODO sibling = get("/number")
resp.media = {'value': sibling.value+1}
api = falcon.API()
api.add_route('/number', NumberResource())
api.add_route('/next', NextNumberResource())
Now I can store the number 17:
curl http://localhost:8000/number -d '{"value":17}'
And I can retrieve the stored value:
curl http://localhost:8000/number
{"value": 17}
I want to fill in the TODO line so that I can retrieve the calculated value:
curl http://localhost:8000/next
{"value": 18}
What's the best way to do that?
1 Answer
1
The best way to do this would be via OOP pattern in Python. An example could be like this (Albeit, it needs more defensive programming + serialization/de-serialization)
class SomeLogicHandler():
myValue #Important to have myValue as class variable and not as instance variable.
def __init__(self):
super(SomeLogicHandler, self).__init()
self.updateMyValue = self._updateMyValue
self.getMyValue = self._getMyValue
def _updateMyValue(self, value):
self.myValue+= value
def _getMyValue(self):
return {value: self.myValue}
class ApiRouteHandler(SomeLogicHandler):
def __init__(self):
super(ApiRouteHandler, self).__init__()
def on_post(self, req, resp):
#post implementation.
self.updateMyValue(req.media.get('value'))
class ApiRouteHandler2(SomeLogicHandler):
def __init__(self):
super(ApiRouteHandler2, self).__init__()
def on_get(self, req, resp):
#get implementation.
resp.body = self.getMyValue()
SomeLogicHandler
Thanks for the update. I usually decouple all logic handlers into a separate package and import them within API route handlers. I missed mentioning that in my response above. I shall update it. Thanks again.
– Pruthvi Kumar
Jul 3 at 2:35
This is still not real code.
– Stephen Rauch
Jul 3 at 2:57
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You are using
SomeLogicHandler
before it is defined. Does this actually work for you?– Stephen Rauch
Jul 3 at 2:20