Find current Doctrine database connection settings in symfony
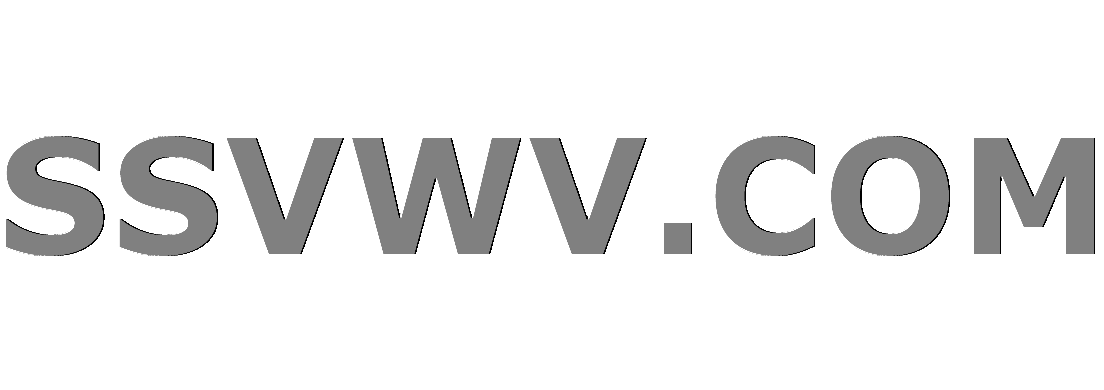
Multi tool use
Find current Doctrine database connection settings in symfony
I need to know the database name and database server name inside a symfony project. How can one access the current database connection settings programmatically in symfony (using Doctrine)?
5 Answers
5
for example:
foreach(Doctrine_Manager::getInstance()->getConnections() as $connection){
$conn = $connection->getOptions();
preg_match('/host=(.*);/', $conn['dsn'], $host);
var_dump($host);
}
Assuming you have the EntityManager as $this->em
$this->em
Get Doctrine Database Name from Symfony2:
$this->em->getConnection()->getDatabase();
Get Doctrine Host Name (Server Name) from Symfony2:
$this->em->getConnection()->getHost();
There are many other parameters you can access from the connection such as username
, port
and password
. See the connection class for more info
username
port
password
The dbname in the dsn for a specific conexion:
databases.yml
all:
conexion1:
class: sfDoctrineDatabase
param:
dsn: 'mysql:host=localhost;dbname=basegestion1'
username: miusuario
password: ********
conexion2:
class: sfDoctrineDatabase
param:
dsn: 'mysql:host=localhost;dbname=baseestadisticas1'
username: miusuario
password: ********
In the Action:
$mConexion1Options = Doctrine_Manager::getInstance()->getConnection('conexion1')->getOptions();
preg_match('/dbname=(.*)/', $mConexion1Options['dsn'], $mDbConexion1);
Then, the dbname is:
echo $mDbConexion1[1]; //basegestion1
Use this code:
$myConnection = Doctrine_Manager::getInstance()->getConnection('doctrine')->getOptions();
$dsnInfo = $this->parseDsn($myConnection['dsn']);
$settings = array();
$settings['dbUser'] = (string) $myConnection["username"];
$settings['dbPassword'] = (string) $myConnection["password"];
$settings['dbHost'] = (string) $dsnInfo["host"];
$settings['dbName'] = (string) $dsnInfo['dbname'];
private function parseDsn ($dsn)
{
$dsnArray = array();
$dsnArray['phptype'] = substr($dsn, 0, strpos($dsn, ':'));
preg_match('/dbname = (w+)/', $dsn, $dbname);
$dsnArray['dbname'] = $dbname[1];
preg_match('/host = (w+)/', $dsn, $host);
$dsnArray['host'] = $host[1];
return $dsnArray;
}
Try this one
$conn = Doctrine_Manager::getInstance()->getConnection('doctrine')->getOptions();
$dns_array = split(';', $conn['dsn']);
preg_match('/host=(.*);/', $dns_array, $dbhost);
preg_match('/dbname=(.*)/', $dns_array, $dbname);
$dbname = $dbname;
$dbhost = $dbhost;
$dbuser = $conn['username'];
$dbpass = $conn['password'];
Updated
As Function split() is deprecated
so here is recommended way.
Function split() is deprecated
$dns_array = explode(';', $conn['dsn']);
preg_match('/host=(.*)/', $dns_array[0], $dbhost);
preg_match('/dbname=(.*)/', $dns_array[2], $dbname);
$dbhost = $dbhost[1];
$dbname = $dbname[1];
$dbuser = $conn['username'];
$dbpass = $conn['password'];
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I think there is a much more elegant way, see stackoverflow.com/a/26505585/744975
– Andrew Atkinson
Oct 22 '14 at 10:40