Sleeping in jest tests (using async await) does not work
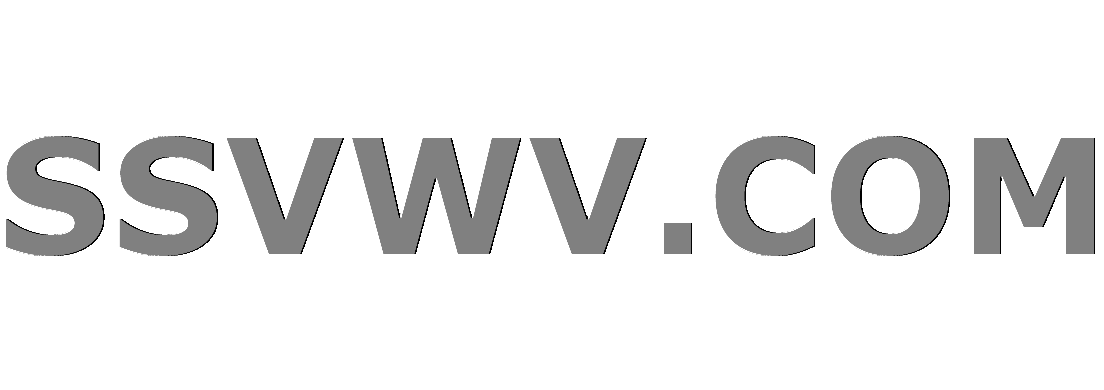
Multi tool use
Sleeping in jest tests (using async await) does not work
I need to pause the test for a certain time in Jest. Since I am running integration tests I don't want to use mock timers. My first approach was to do this:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
describe('User Actions', () => {
it('should refresh tokens', () => {
(async () => {
console.log('Start');
await sleep(10000);
console.log('End');
})();
})
});
It did not work. Jest simply get stuck on the sleep function indefinitely! My second approach was to do this:
describe('User Actions', () => {
it('should refresh tokens', () => {
(async () => {
console.log('Start');
setTimeout(() => {
console.log('End');
}, 10000);
})();
})
});
Since I am using Puppeteer, my third option was to use waitFor function from Puppeteer. But that does not work either:
describe('User Actions', () => {
it('should refresh tokens', () => {
(async () => {
console.log('Start');
await page.waitFor(10000);
console.log('End');
})();
})
});
The --detectOpenHandles
argument does not show any open handles. What is going on? Why does not Jest not wait?
--detectOpenHandles
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.