JSON manipulations in Spring boot & Angular
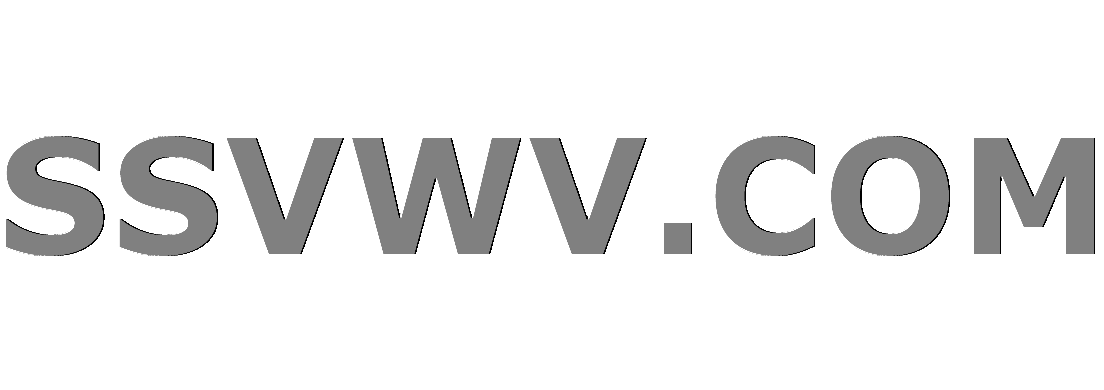
Multi tool use
JSON manipulations in Spring boot & Angular
I'm using Spring Bootand querying a MySQL db, using the following code
@GetMapping("/dashboard")
public String Date() {
Connection conn = null;
List<Map<String, Object>> listOfDates = null;
try{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Connecting to database...To retrive DATE");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
String countQuery= "SELECT migrations.mignum, migration_states.statemigrations.projectleader, migrations.productiondate,"
+ " migrations.installationtiers, migrations.targetplatform, migrations.apprelated, migrations.appversion FROM migrations, migration_states WHERE migrations.productiondate='2018-07-07";
QueryRunner queryRunner = new QueryRunner();
listOfDates = queryRunner.query(conn, countQuery, new MapListHandler());
conn.close();
}
catch (SQLException se) {
se.printStackTrace();
}catch(Exception e){
//Handle errors for Class.forName
e.printStackTrace();
}finally {
DbUtils.closeQuietly(conn);
}
return new Gson().toJson(listOfDates);
}
which will return me a JSon object like below
[
{"state":"Approval in Staging","mignum":146384,"projectleader":"James Rice","productiondate":"Jul 7, 2018","installationtiers":"Linux Web WL10","targetplatform":"Production","apprelated":"Content Only","appversion":""},
{"state":"Approval by QA in Staging","mignum":146451,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"UPS Pickup Point Attribute Injector","appversion":"18.7.1"},
{"state":"Approval by QA in Staging","mignum":146453,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"UPS Pickup Point DB Web Services","appversion":"18.7.1"},
{"state":"Migration to Mahwah","mignum":146485,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Account Invoice Authorization","appversion":"18.07.01"},
{"state":"Migration to Mahwah","mignum":146487,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL10","targetplatform":"Production","apprelated":"My Choice Enrollment Component","appversion":"18.07.03"},
{"state":"Migration to Mahwah","mignum":146489,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"My Choice Enrollment WebApp","appversion":"18.07.01"},
{"state":"Migration to Mahwah","mignum":146492,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL81","targetplatform":"Production","apprelated":"LASSO","appversion":"UTA_18.07.03"},{"state":"Approval by QA in Staging","mignum":146495,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux Web WL10","targetplatform":"Production","apprelated":"LASSO","appversion":"18.07.03"},{"state":"Approval by QA in Staging","mignum":146496,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux Web WL10","targetplatform":"Production","apprelated":"LASSO","appversion":"18.07.03"},{"state":"Approval by QA in Staging","mignum":146497,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Precommissioning Authorization ","appversion":"18.07.09"},{"state":"Approval by QA in Staging","mignum":146498,"projectleader":"Keith Lucas","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Precommissioning Authorization ","appversion":"18.07.06"},{"state":"Approval by Dev Staging","mignum":146547,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Account Validation Component ","appversion":""},{"state":"Migration to Mahwah","mignum":146549,"projectleader":"Amardeep Grewal","productiondate":"Jul 7, 2018","installationtiers":"Linux Web WL10","targetplatform":"Production","apprelated":"URL Alias","appversion":""},{"state":"Approval by QA in Staging","mignum":146565,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Quantum View SubServer","appversion":"9.3.0"},
{"state":"Approval by Dev Staging","mignum":146566,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Web Email Preference App","appversion":"v3.8.19"},
{"state":"Approval by QA in Staging","mignum":146569,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"View Bill","appversion":"4.0.2"},
{"state":"Migration to Mahwah","mignum":146578,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Claims History Component","appversion":"NA"},
{"state":"Approval by QA in Staging","mignum":146579,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Address Search Component ","appversion":"2.1.0"},
{"state":"Approval by Dev Staging","mignum":146581,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"DCOWS","appversion":" 8.03.01"},
....
]
Can anyone let me know,how to perform the following manipulations in Spring side or Angular side
Find the count of each state so that I get Json array as follows
[
{"state":"Approval by Dev Staging", "count": 12},
{"state":"Approval by QA in Staging", "count": 12},
...
]
Get the data present for each state Eg:
if state = "Approval by Dev Staging"
get its details as json object to display in front-end Angular
if state = "Approval by QA in Staging"
get its details as json object to display in front-end Angular
Eg:
[{
"name":"Approval by QA in Staging",
"value":[
{"mignum":146547,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Account Validation Component ","appversion":"xxx"},
{"mignum":146547,"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Account Validation Component ","appversion":"xxx"},
....
}]
},
{
"name":"Migrations to Staging",
"value":[{
{"mignum":146547,
"projectleader":"Eric Lok","productiondate":"Jul 7, 2018","installationtiers":"Linux BEA WL12","targetplatform":"Production","apprelated":"Account Validation Component ","appversion":"xxx"} ....
}]
}]
.... etc
It will be coming from query to MYSQL "SELECT migrations.mignum, migration_states.state AS Current_State FROM migrations, migration_states WHERE ((migrations.productiondate=(SELECT valval FROM global_configs WHERE valname='erday')))"
– user9414660
Jul 2 at 17:06
2 Answers
2
For the second question, I was able to acheive it in Angular side using the following code
in dashboard.service.ts by calling as below
getDateDashboard() {
return this._http.get(this.baseUrl + '/dashboard', this.options).pipe(map((response: Response) => response.json()));
}
in *dashboard.component.ts* by making a call to above service as follows
for (let index = 0; index < chartdataa.length; index++) {
if(chartdataa[index].state=="Approval by Dev. in Mahwah"){
this.displayDevMawah.data = chartdataa.filter(function(data:any){ return data.state == "Approval by Dev. in Mahwah";});
this.displayDevMawah.count = this.displayDevMawah.data.length;
console.log("this.displayDevMawah.count-->", this.displayDevMawah.count);
}
if(chartdataa[index].state=="Approval by Dev. in Windward"){
this.displayDevWindward.data = chartdataa.filter(function(data:any){ return data.state == "Approval by Dev. in Windward";});
this.displayDevWindward.count = this.displayDevWindward.data.length;
console.log("this.displayDevWindward.count-->", this.displayDevWindward.count);
}
...
}
Now I need help to get json data for my first question from the above result, which is
Getting the json data with state and count for each state in Angular side/ Spring side as follows
[
{"state":"Completed","count":240},
{"state":"Pending Approval by Development in Windward","count":2},
{"state":"Pending Approval by QA in Windward","count":1},
{"state":"Pending Migration to Mahwah","count":1},
{"state":"Pending Migration to Production","count":3},
...
]
public String url(@RequestBody String jsonData) {
String messageId="0";
try {
ObjectMapper objectMapper = new ObjectMapper();
Message ms = new Message(); //write your class name that uses database entities
Message message = objectMapper.readValue(jsonData, Message.class);
if (message != null) {
String text= message.getMessage();
messageRepository.save(ms);//enter reposistory that use in controller
ms.getMessageId().toString();
}
} catch (Exception ex) {
String errorMsg = ex.getMessage();
if(errorMsg!="")
return messageId + " donot save Error ";
}
return messageId;
}
Got the json object only
@GetMapping("/dashboard") public String Date() { Connection conn = null; List<Map<String, Object>> listOfDates = null; String countQuery= "SELECT migrations.mignum, migration_states.state FROM migrations, migration_states WHERE migrations.productiondate='2018-07-07"; QueryRunner queryRunner = new QueryRunner(); listOfDates = queryRunner.query(conn, countQuery, new MapListHandler()); conn.close(); return new Gson().toJson(listOfDates); }
want 2 display 1. Count by each state 2. Get all data w.r.t state– user9414660
Jul 2 at 18:35
@GetMapping("/dashboard") public String Date() { Connection conn = null; List<Map<String, Object>> listOfDates = null; String countQuery= "SELECT migrations.mignum, migration_states.state FROM migrations, migration_states WHERE migrations.productiondate='2018-07-07"; QueryRunner queryRunner = new QueryRunner(); listOfDates = queryRunner.query(conn, countQuery, new MapListHandler()); conn.close(); return new Gson().toJson(listOfDates); }
Please format the code properly and describe what tge code is doing. And check
if(errorMsg!="")
– Marged
Jul 2 at 19:36
if(errorMsg!="")
@Marged I added the code to the question for clarity
– user9414660
Jul 2 at 19:58
Thanks this is only spring side code. in angularJS or javascript you can call it with loop for multiple inserts.
– M Faiz
Jul 4 at 7:18
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
where do you get the json object from? is it coming through some http api, or is it in a file, or a literal string? database?
– eis
Jul 2 at 15:12