Spring Boot - OAuth with Roles and Authorities
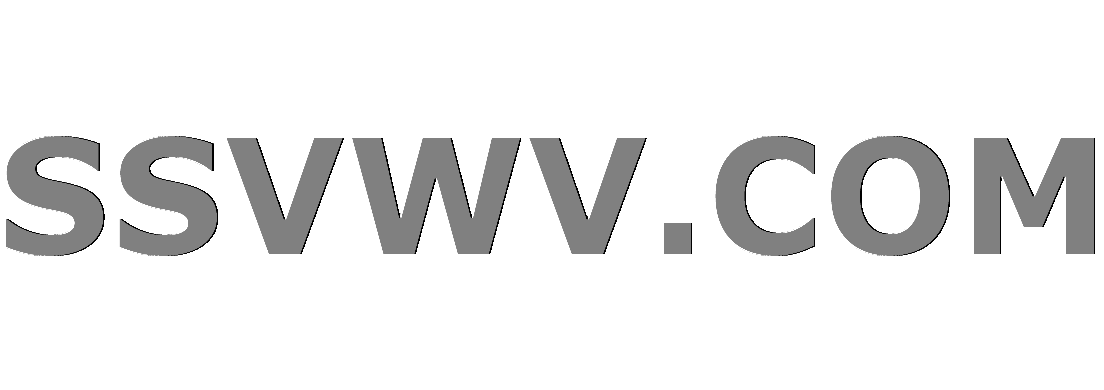
Multi tool use
Spring Boot - OAuth with Roles and Authorities
I am new to Spring Boot, and I am trying to configure OAuth 2.0. I was able to access the API using the access token, but am getting 403 when i am trying access JSP pages.
AuthorizationServerConfig.java
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Bean
public JwtAccessTokenConverter accessTokenConverter() {
JwtAccessTokenConverter converter = new JwtAccessTokenConverter();
converter.setSigningKey("QWERTY");
return converter;
}
@Bean
public TokenStore tokenStore() {
return new JwtTokenStore(accessTokenConverter());
}
@Override
public void configure(ClientDetailsServiceConfigurer configurer) throws Exception {
configurer.inMemory().withClient(Utils.CLIEN_ID).secret(Utils.CLIENT_SECRET).authorities("ADMIN", "AGENT")
.authorizedGrantTypes(Utils.GRANT_TYPE_PASSWORD, Utils.AUTHORIZATION_CODE, Utils.REFRESH_TOKEN,
Utils.IMPLICIT)
.scopes(Utils.SCOPE_READ, Utils.SCOPE_WRITE, Utils.TRUST)
.accessTokenValiditySeconds(Utils.ACCESS_TOKEN_VALIDITY_SECONDS)
.refreshTokenValiditySeconds(Utils.FREFRESH_TOKEN_VALIDITY_SECONDS);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenStore(tokenStore()).authenticationManager(authenticationManager)
.accessTokenConverter(accessTokenConverter());
}
}
WebSecurityConfig.java
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Resource(name = "userService")
private UserDetailsService userDetailsService;
@Override
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Autowired
public void globalUserDetails(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(encoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable().anonymous().disable().authorizeRequests()
.antMatchers("/auth/**").hasRole("ADMIN");
}
@Bean
public BCryptPasswordEncoder encoder() {
return new BCryptPasswordEncoder();
}
@Bean
public FilterRegistrationBean corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("*");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
FilterRegistrationBean bean = new FilterRegistrationBean(new CorsFilter(source));
bean.setOrder(0);
return bean;
}
}
UserDetailServiceImplementation.java
@Service(value = "userService")
public class UserDetailServiceImplementation implements UserDetailsService {
@Autowired
private UserRepository userRepository;
public UserDetailServiceImplementation() {
super();
}
@Override
public UserDetails loadUserByUsername(String sLoginID) throws UsernameNotFoundException {
// TODO Auto-generated method stub
User user = userRepository.findByLoginID(sLoginID);
if (user == null) {
throw new UsernameNotFoundException(sLoginID);
}
return new org.springframework.security.core.userdetails.User(sLoginID, user.getsPassword(),
getAuthorities(user.getiUserTypeID()));
}
public Collection<? extends GrantedAuthority> getAuthorities(Integer role) {
List<GrantedAuthority> authList = getGrantedAuthorities(getRoles(role));
return authList;
}
public List<String> getRoles(Integer role) {
List<String> roles = new ArrayList<String>();
if (role.intValue() == 1) {
roles.add("ROLE_AGENT");
roles.add("ROLE_ADMIN");
} else if (role.intValue() == 2) {
roles.add("ROLE_MANAGER");
}
return roles;
}
public static List<GrantedAuthority> getGrantedAuthorities(List<String> roles) {
List<GrantedAuthority> authorities = new ArrayList<GrantedAuthority>();
for (String role : roles) {
authorities.add(new SimpleGrantedAuthority(role));
}
return authorities;
}
}
ResourceServerConfig.java
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
private static final String RESOURCE_ID = "resource_id";
@Override
public void configure(ResourceServerSecurityConfigurer resources) {
resources.resourceId(RESOURCE_ID).stateless(false);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.anonymous().disable().authorizeRequests()
.antMatchers("/auth/admin/**").access("hasRole('ROLE_ADMIN')").and().exceptionHandling()
.accessDeniedHandler(new OAuth2AccessDeniedHandler());
}
}
When I try to access oauth/token in Postman And RestTemplate, I was able to retrieve the access_token and other parameters. Controller part of my application has RestTemplate, that retrieve the data from the API, based on the API when we re-direct to to any page, it say forbidden because of the hasRole function. Whether I need to implement separate security configuration for JSP pages?
@Ashish451 I haven't used that, instead of that i have added properties in application.properties file
– Jay Vignesh
Jul 3 at 4:39
What properties you have added in application.properties
– Ashish451
Jul 3 at 4:43
If this is a new application, using a different template system (I prefer Thymeleaf) is preferable to JSP because of serious limitations in the JSP architecture.
– chrylis
Jul 3 at 4:54
@Ashish451 spring.mvc.view.prefix=/WEB-INF/jsp/ spring.mvc.view.suffix=.jsp
– Jay Vignesh
Jul 3 at 5:06
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What about your view Resolver code...
– Ashish451
Jul 3 at 4:30