Populate a list view in asp.net winform by using stored procedure with parameters through a button click
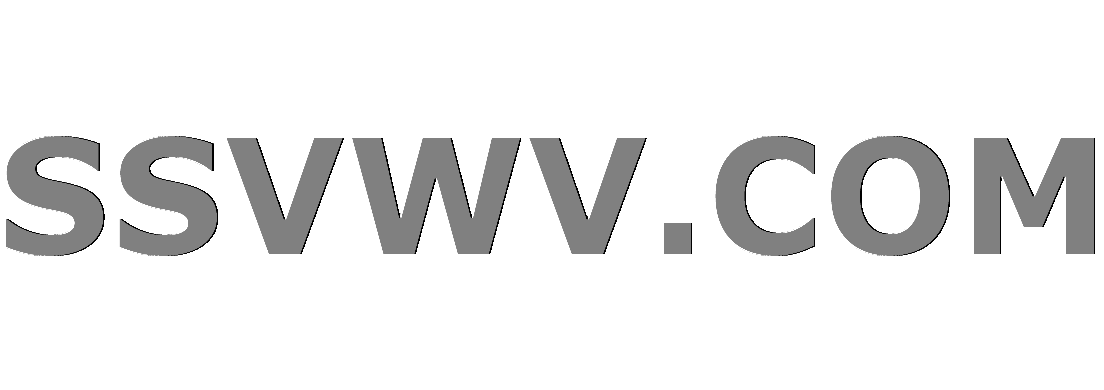
Multi tool use
Populate a list view in asp.net winform by using stored procedure with parameters through a button click
In my Winforms application, I have to do search by using three parameters. I retrieve from the database by using stored procedure. But an exception is thrown:
Procedure or function 'sp_searchProduct' expects parameter '@categoryId', which was not supplied
Kindly help me.
This is my code:
private void button2_Click(object sender, EventArgs e)
{
SqlConnection con = new SqlConnection(@"Data Source=DESKTOP-U1OP1S9SQLEXPRESS;Initial Catalog=PaintStores;Integrated Security=True");
SqlCommand command = new SqlCommand("sp_searchProduct", con);
command.Parameters.AddWithValue("@categoryId", comboBox1.SelectedValue.ToString());
command.Parameters.AddWithValue("@typeId", comboBox2.SelectedValue.ToString());
command.Parameters.AddWithValue("@productId", comboBox3.SelectedValue.ToString());
SqlDataAdapter da = new SqlDataAdapter(command);
DataTable dataTable = new DataTable();
da.Fill(dataTable);
for (int i = 0; i < dataTable.Rows.Count; i++)
{
DataRow drow = dataTable.Rows[i];
if (drow.RowState != DataRowState.Deleted)
{
ListViewItem lvi = new ListViewItem(drow["ProductId"].ToString());
lvi.SubItems.Add(drow["ProductName"].ToString());
lvi.SubItems.Add(drow["TypeName"].ToString());
lvi.SubItems.Add(drow["Quantity"].ToString());
lvi.SubItems.Add(drow["Price"].ToString());
lvi.SubItems.Add(drow["Stock"].ToString());
listView1.Items.Add(lvi);
}
}
con.Close();
}
This is my stored procedure:
ALTER PROCEDURE [dbo].[sp_searchProduct]
@categoryId INT,
@typeId INT,
@productId INT
AS
IF (@categoryId = 0)
BEGIN
SET @categoryId = NULL
END
IF (@typeId = 0)
BEGIN
SET @typeId = NULL
END
IF (@productId = 0)
BEGIN
SET @productId = NULL
END
BEGIN
SET NOCOUNT ON;
SELECT p.ProductId, p.ProductName, p.Quantity, p.Price, p.Stock
FROM tblProductNew p
INNER JOIN tblTypes t ON t.TypeId = p.TypeId
WHERE p.ProductId = ISNULL(@productId, p.ProductId)
AND p.TypeId = ISNULL(@typeId, p.TypeId)
AND t.MainCategoryId = ISNULL(@categoryId, t.MainCategoryId)
END
I included it in my question
– Tharani Gnanasegaram
May 27 '17 at 11:35
@Chirag I corrected the mistakes you have mentioned, and the exception is gone now. But it didn't give the answer to the query. It displays the whole set of data in the list. I debug the line "listView1.Items.Add(lvi);". It give the query list. But in the view the whole data is displayed. Kindly help me.
– Tharani Gnanasegaram
May 27 '17 at 12:53
Side note: you should not use the
sp_
prefix for your stored procedures. Microsoft has reserved that prefix for its own use (see Naming Stored Procedures), and you do run the risk of a name clash sometime in the future. It's also bad for your stored procedure performance. It's best to just simply avoid sp_
and use something else as a prefix - or no prefix at all!– marc_s
Jul 2 at 17:00
sp_
sp_
3 Answers
3
Make sure you add this to your code before calling da.Fill(dataTable);
da.Fill(dataTable);
command.CommandType = CommandType.StoredProcedure;
Your final code should look something like this..
SqlConnection con = new SqlConnection(@"Data Source=DESKTOP-U1OP1S9SQLEXPRESS;Initial Catalog=PaintStores;Integrated Security=True");
SqlCommand command = new SqlCommand("sp_searchProduct", con);
command.CommandType = CommandType.StoredProcedure;
command.Parameters.AddWithValue("@categoryId",comboBox1.SelectedValue.ToString());
It works. The exception is gone now. But it didn't give the answer to the query. It displays the whole set of data in the list.
– Tharani Gnanasegaram
May 27 '17 at 11:49
@Coderstech Must be something wrong with the values you pass or the logic in the stored procedure. I suggest you to debug and see what values are being passed to proc.
– 5a7335h
May 27 '17 at 11:52
I debug it. the line "listView1.Items.Add(lvi);" gives only the query results. But in the view all the data are displayed
– Tharani Gnanasegaram
May 27 '17 at 12:05
There are two mistakes. One is you should specify command type. Second is, SP expects categoryId as int and you're converting it into string.
SqlConnection con = new SqlConnection(@"Data Source=DESKTOP-U1OP1S9SQLEXPRESS;Initial Catalog=PaintStores;Integrated Security=True");
SqlCommand command = new SqlCommand("sp_searchProduct", con);
command.CommandType = CommandType.StoredProcedure;
command.Parameters.AddWithValue("@categoryId", comboBox1.SelectedValue);
command.Parameters.AddWithValue("@typeId", comboBox2.SelectedValue);
command.Parameters.AddWithValue("@productId", comboBox3.SelectedValue);
SqlDataAdapter da = new SqlDataAdapter(command);
DataTable dataTable = new DataTable();
da.Fill(dataTable);
I corrected them, and the exception is gone now. But it didn't give the answer to the query. It displays the whole set of data in the list.
– Tharani Gnanasegaram
May 27 '17 at 11:51
The mistake is I missed to clear the earlier results in the list view. I use the line listView1.Items.Clear();
before I insert the queried results to the list view. Now the error gone.
listView1.Items.Clear();
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
May I Have your SP?
– Chirag
May 27 '17 at 11:30