Is there an API for getting original Properties.Settings?
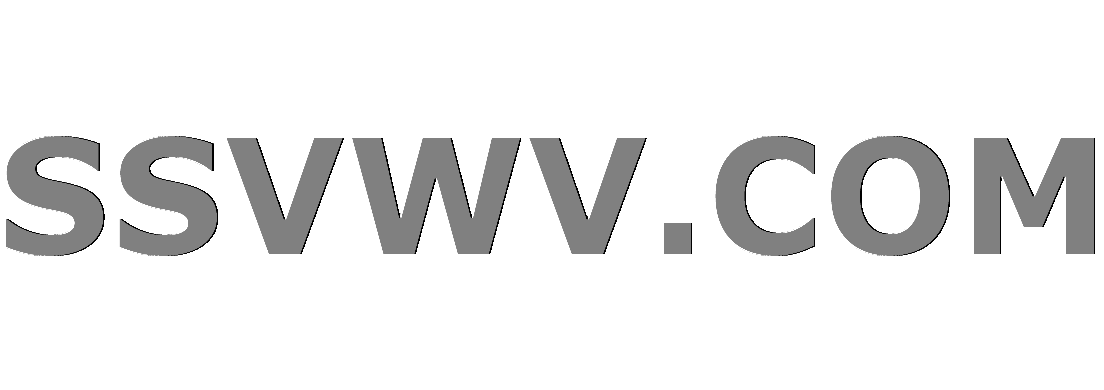
Multi tool use
Is there an API for getting original Properties.Settings?
When you access for example:
Properties.Settings.Default.myColor
you will get actually a current setting of the value of myColor
, not the original one which was set during the development of the program.
myColor
And I am looking exactly for it -- the values which were set originally as default. They can be seen again when the current settings are deleted.
Of course, I am looking for an API to get those values without deleting current settings.
@Sweeper, thank you, I know, but it would mean having two sources of those values. Not clean approach for my taste :-)
– astrowalker
Jul 3 at 7:59
2 Answers
2
You can find default value for a setting property by property name this way:
var value = (string)Properties.Settings.Default.Properties["propertyName"].DefaultValue;
But the returned value is string
representation of the property value, for example, if you look at Settings.Designer.cs
you will see the property is decorated with an attribute which stores the default value [DefaultSettingValueAttribute("128, 128, 255")]
. In this case the return value for above code will be "128, 128, 225"
.
string
Settings.Designer.cs
[DefaultSettingValueAttribute("128, 128, 255")]
"128, 128, 225"
To be able to get the default value in the original property type, I created the following extension method:
using System.ComponentModel;
using System.Configuration;
public static class SettingsExtensions
{
public static object GetDefaultValue(this ApplicationSettingsBase settings,
string propertyName)
{
var property = settings.Properties[propertyName];
var type = property.PropertyType;
var defaultValue = property.DefaultValue;
return TypeDescriptor.GetConverter(type).ConvertFrom(defaultValue);
}
}
Then as usage:
var myColor = (Color)Properties.Settings.Default.GetDefaultValue("myColor");
Thank you, but this does not work. I guess
DefaultValue
holds serialized value (so you cannot cast it, only deserialize it first and then cast) but I am not sure why DefaultValue
is object
then not a string
. And I noticed the default value is stored as attribute, thus I wrote my code using it.– astrowalker
Jul 3 at 12:08
DefaultValue
DefaultValue
object
string
Let me check. I guess it needs tweaking. I tried with string in fact ...
– Reza Aghaei
Jul 3 at 12:09
@astrowalker Yes, it returns string string stored value. I used an extension method to convert it back to the original property type.
– Reza Aghaei
Jul 3 at 12:23
Changed a bit. Make sure use the latest version.
– Reza Aghaei
Jul 3 at 12:27
I didn't find any existing API but I needed it so...
I made VS to create interface for entire Settings (in user part, not designer):
internal sealed partial class Settings : ISettings
this is only for easier using both settings (current and default)
Interface looks like this:
internal interface ISettings
{
bool AskForNewDescription { get; }
...
I created default settings class:
internal sealed class DefaultSettings : ISettings
{
public bool AskForNewDescription => GetDefault<bool>();
I didn't tested it for all possible cases, but it works for me:
private readonly ISettings source;
private string GetSerialized(string name)
{
var prop = this.source.GetType().GetProperty(name);
foreach (object attr in prop.GetCustomAttributes(true))
{
var val_attr = attr as DefaultSettingValueAttribute;
if (val_attr != null)
return val_attr.Value;
}
return null;
}
private T GetDefault<T>([CallerMemberName] string name = null)
{
string s = GetSerialized(name);
if (s == null)
return default(T);
TypeConverter tc = TypeDescriptor.GetConverter(typeof(T));
return (T)tc.ConvertFromString(s);
}
There is a built-in mechanism which allows you to get the property default value shared in the other answer.
– Reza Aghaei
Jul 3 at 12:02
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You can just create constants/readonly fields in your code.
– Sweeper
Jul 3 at 7:58