Error while writing into pipe in c++
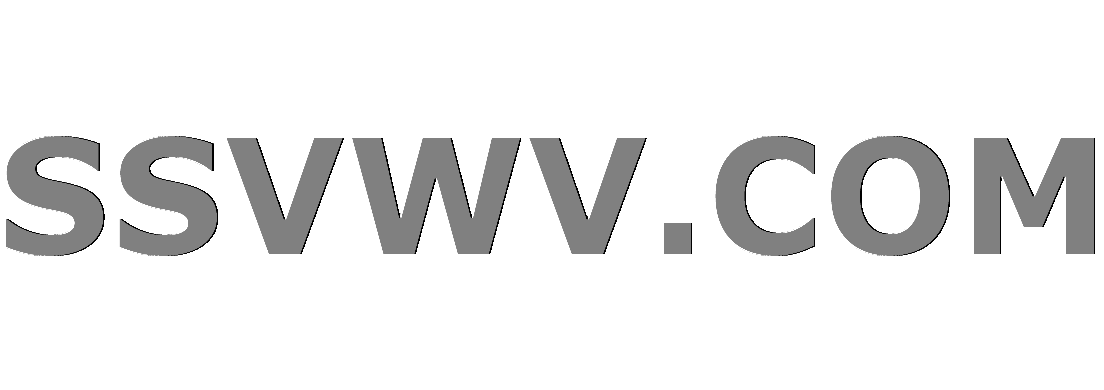
Multi tool use
Error while writing into pipe in c++
I'm trying to implement basic named pipe implementation.
In my client code if I call fun()
only one time then my program runs completely fine.
But when I call fun()
more than one time then it fails alternatively(e.g. if I call fun()
3 times, first call will be successful, second call will fail and third call runs fine.
When the call fails in GetLastError()
I get 231
as output on my screen.
If I use Sleep()
after every fun()
call in client then my program runs fine for every fun()
call.
If I use Sleep()
in my server program then it reads from from buffer only one time and all the calls (after the first call) to fun()
fail in client side.
fun()
fun()
fun()
GetLastError()
231
Sleep()
fun()
fun()
Sleep()
fun()
Server Code:
#include<stdio.h>
#include<windows.h>
#include<iostream>
#include<stdlib.h>
using namespace std;
int main(void)
{
HANDLE hPipe;
char buffer[1024];
DWORD dwRead;
hPipe = CreateNamedPipe(TEXT("\.pipePipe"),
PIPE_ACCESS_INBOUND | FILE_FLAG_FIRST_PIPE_INSTANCE,
PIPE_TYPE_BYTE | PIPE_READMODE_BYTE | PIPE_WAIT, // FILE_FLAG_FIRST_PIPE_INSTANCE is not needed but forces CreateNamedPipe(..) to fail if the pipe already exists...
1,
1024 * 16,
1024 * 16,
INFINITE,
NULL);
while (hPipe != INVALID_HANDLE_VALUE)
{
switch (ConnectNamedPipe(hPipe, NULL) ? NOERROR : GetLastError())
{
case NOERROR:
case ERROR_PIPE_CONNECTED: // STATUS_PIPE_CONNECTED
case ERROR_NO_DATA: // STATUS_PIPE_CLOSING
while (ReadFile(hPipe, buffer, sizeof(buffer)-1, &dwRead, NULL))
{
// do something with data in buffer
//Sleep(2000);
buffer[dwRead] = '';
printf("%s", buffer);
}
DisconnectNamedPipe(hPipe);
break;
}
//CloseHandle(hPipe);
}
return 0;
}
Client Code:
#include<stdio.h>
#include<windows.h>
#include<iostream>
#include<stdlib.h>
using namespace std;
void fun()
{
HANDLE hPipe;
DWORD dwWritten;
hPipe = CreateFile(TEXT("\.pipePipe"),
GENERIC_WRITE,
0,
NULL,
OPEN_EXISTING,
0,
NULL);
if (hPipe != INVALID_HANDLE_VALUE)
{
WriteFile(hPipe,
"Hello Pipen",
12, // = length of string + ''
&dwWritten,
NULL);
CloseHandle(hPipe);
}
else
{
cout<<"****";
cout<<GetLastError();
cout<<"****";
}
}
int main(void)
{
fun(); //Success
//Sleep(2000);
fun(); //failure
//Sleep(2000);
fun(); //Success
//Sleep(2000);
fun(); //failure
//Sleep(2000);
fun(); //Success
return (0);
}
What does the debugger say?
– JHBonarius
Jul 3 at 6:43
why you drop
WaitNamedPipe
from here ?– RbMm
Jul 3 at 6:49
WaitNamedPipe
Try to set parameter
nMaxInstances
of CreateNamedPipe()
to PIPE_UNLIMITED_INSTANCES
.– zett42
Jul 3 at 17:53
nMaxInstances
CreateNamedPipe()
PIPE_UNLIMITED_INSTANCES
Also your
while (hPipe != INVALID_HANDLE_VALUE)
should be if (hPipe != INVALID_HANDLE_VALUE)
as hPipe
never changes inside of the loop. You will never break out of the loop.– zett42
Jul 3 at 17:57
while (hPipe != INVALID_HANDLE_VALUE)
if (hPipe != INVALID_HANDLE_VALUE)
hPipe
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Possible duplicate of Named Pipe CreateFile() returns INVALID_HANDLE_VALUE, and GetLastError() returns ERROR_PIPE_BUSY
– Sami Kuhmonen
Jul 3 at 6:39