Flutter: ListView.builder create columns
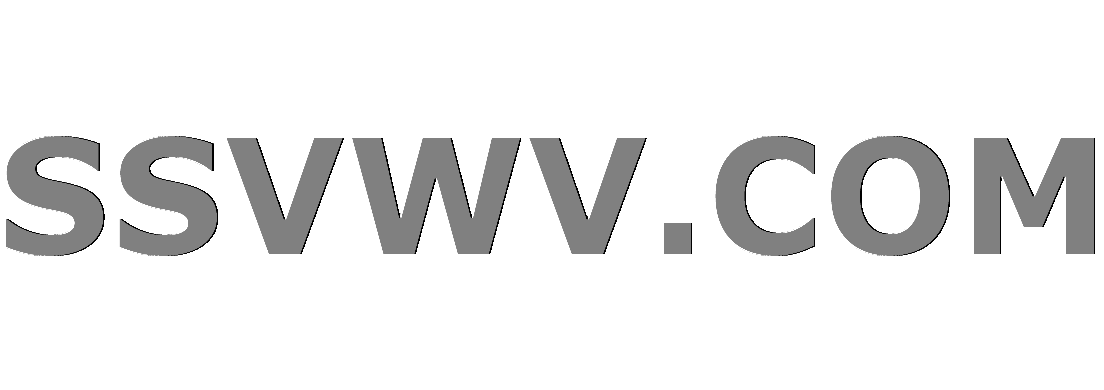
Multi tool use
Flutter: ListView.builder create columns
I am developing a flutter-app for some counting games. I am trying to get it working, that one ListView.builder is displaying two items in one column and the other two items in another column. But i do not know how to do that. Any suggestions?
Here is my code:
import 'dart:math';
import 'package:flutter/material.dart';
class Teams extends StatefulWidget{
final String spieler1;
final String spieler2;
final String spieler3;
final String spieler4;
Teams({Key key, this.spieler1, this.spieler2, this.spieler3, this.spieler4}) : super(key : key);
@override
State<StatefulWidget> createState() => new TeamsState();
}
class TeamsState extends State<Teams>{
@override
Widget build(BuildContext context) {
var namen = [widget.spieler1, widget.spieler2, widget.spieler3, widget.spieler4];
List<String> erstellt = shuffle(namen);
return new Scaffold(
appBar: new AppBar(
actions: <Widget>[
IconButton(
icon: Icon(Icons.shuffle),
onPressed: (){setState(() {});}
),
],
),
body: new ListView.builder(
itemCount: namen.length,
itemBuilder: (BuildContext context, int index){
return new ListTile(
title: new Text(erstellt[index]), // <-- content is loading here
);
}
),
);
}
List shuffle(List items) {
var random = new Random();
// Go through all elements.
for (var i = items.length - 1; i > 0; i--) {
// Pick a pseudorandom number according to the list length
var n = random.nextInt(i + 1);
var temp = items[i];
items[i] = items[n];
items[n] = temp;
}
return items;
}
}
1 Answer
1
I think what you are describing is whats known as a GridView in flutter. To achieve two columns this is an example of what you can do:
GridView.count(
// crossAxisCount is the number of columns
crossAxisCount: 2,
// This creates two columns with two items in each column
children: List.generate(2, (index) {
return Center(
child: Text(
'Item $index',
style: Theme.of(context).textTheme.headline,
),
);
}),
);
You can read more about it on Flutter's Website: https://flutter.io/cookbook/lists/grid-lists/
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Could you put a screenshot about what are you trying to do?
– diegoveloper
Jul 3 at 1:58