Catch on JS Promise being called but not calling other jest mocked object
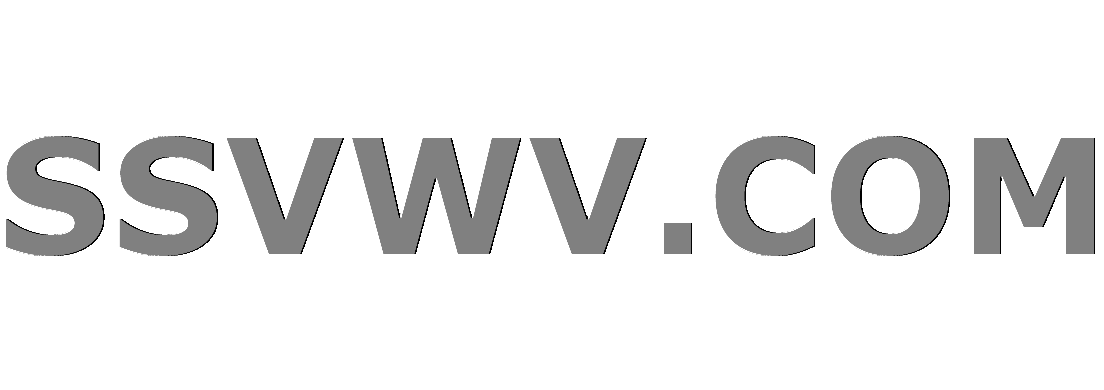
Multi tool use
Catch on JS Promise being called but not calling other jest mocked object
I have a simple js function that tries to connect to mongodb via a Promise and either succeeds of fails.
const MongodbService = () => {
mongoose.connect(mongodbUrl, options).then(() => {
logger.info('Mongodb Connected', { url: process.env.MONGODB_URL })
}).catch(err => {
logger.error('Mongodb: ${err}', { url: process.env.MONGODB_URL })
})
return mongoose
}
and I want to simply test it. I mock the logger:
import logger from '../../../config/winston'
jest.mock('../../../config/winston')
I have spec for testing success, which works as expected:
it('it should handle a connection success', async () => {
mongoose.connect = jest.fn(() => Promise.resolve())
await MongodbService()
expect(logger.info.mock.calls[0][0]).toEqual('Mongodb Connected')
expect(logger.info.mock.calls[0][1]).toEqual({ url: 'mongodb://mongodb/jb-dev' })
})
I have another which check for failure, and this is where I'm stuck:
it('it should handle a connection failure', async () => {
mongoose.connect = jest.fn(() => Promise.reject(new Error('boom')))
await MongodbService()
expect(logger.error.mock.calls[0][0]).toEqual('Mongodb Error: boom')
})
This is failing stating the logger has not been called, even though when I put a console.log in the function I can see it has correctly been called. If I add a setTimeout around the expect I can then see the logger being called, but it's been called twice. I think I'm missing something simple. Can anyone point me in the right direction?
1 Answer
1
Doh! So I was miss-understanding the information returned when I thought it was not working. Adding nextTick worked for me just fine:
it('it should handle a connection failure', async () => {
mongoose.connect = jest.fn(() => Promise.reject(new Error('bang')))
await MongodbService()
process.nextTick(() => {
expect(logger.error.mock.calls[0][0]).toEqual('Mongodb: Error: bang')
})
})
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.