Use type defined in C library in C++ program with namespace like scheme
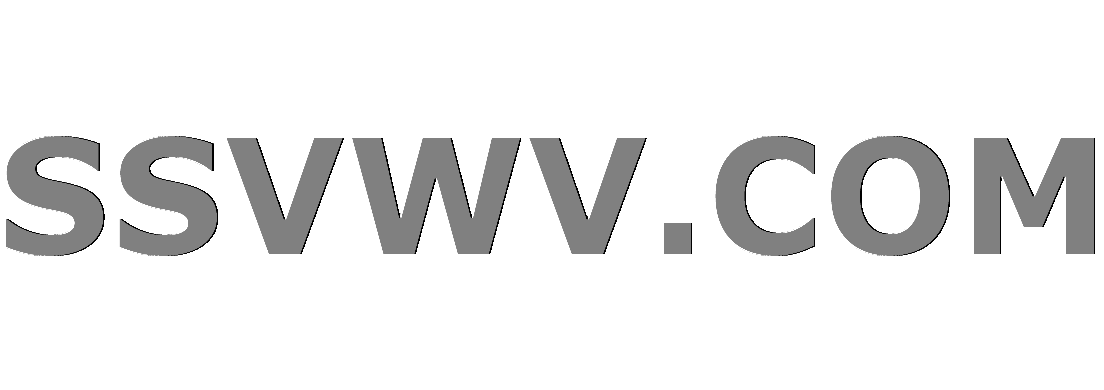
Multi tool use
Use type defined in C library in C++ program with namespace like scheme
I have written a C library which defines some functions and types like the following:
typedef struct {
int i;
} A;
void func1(void);
int func2(A* a);
I now want to use these functions and create variables of type A
in a C++ program. Unfortunately C does not have namespaces but I would still like to emulate some similar structure to avoid name collisions in the C++ program. However I don't like the option of prepending every function and struct with a prefix:
A
typedef struct {
int i;
} library_A;
void library_func1(void);
int library_func2(A* a);
I found this nice way to emulate a namespace scheme by defining a struct which has some pointers to the functions:
/*interface.h*/
struct library {
void (*func1)(void);
void (*func2)(A*);
};
extern const struct library Library;
/* end interface.h */
/* interface.c */
#include "interface.h"
void func1(void)
{
...
}
void func2(A* a)
{
...
}
const struct library Library = {
.func1 = func1,
.func2 = func2,
};
/* end interface.c */
/* C++ program */
#include "interface.h"
int main(void)
{
Library.method1();
A a;
a.i = 5;
Library.method2(&a);
return 0;
}
/* end C++ program */
Is it possible to also include the definition of type A
in Library
or a different way such that a type Library.A
is defined in the C++ program and I can write the C++ program as
A
Library
Library.A
#include "interface.h"
int main(void)
{
Library.method1();
Library.A a;
a.i = 5;
Library.method2(&a);
return 0;
}
1 Answer
1
To my knowledge, it's not possible to do this.
However I don't like the option of prepending every function and struct with a prefix
That is the proper way of handling this situation, though. Think about it this way: namespaces work as prefixes too, the difference is that instead of underscore _
you use double colons ::
.
_
::
Your approach of using a Library
global is surprising from a user's perspective. As a user my first question would be:
Library
Is there a technical reason why I shouldn't use the library functions directly?
If there isn't a reason besides you, the developer, simply not liking how library_XYZ
looks, then my next thought would be:
library_XYZ
Pointless weirdness. The library developer doesn't take this seriously or is inexperienced.
And another thing, it will be extra work for you to maintain the library helper struct
whenever you add, remove or rename stuff from the library. It will eventually become a burden to update that global.
struct
Library.func
library_func
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
There is also another problem: Address of
Library.func
cannot be used to initialize function pointer with static storage duration by library user, butlibrary_func
can be. This limits how library can be used.– user694733
Jul 4 at 11:56