Validate input with regular expression for universal alphabets in javascript
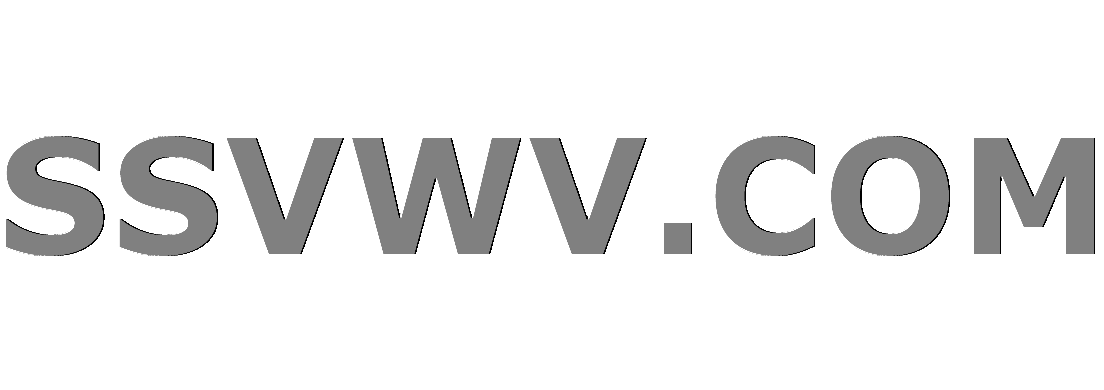
Multi tool use
Validate input with regular expression for universal alphabets in javascript
I have a form validator by AntonLapshin where I'm trying to validate a non-empty input field which can only take alphabets, space, - and '. The alphabets can be a-z, A-Z and europian letters æÆøØåÅöÖéÉèÈüÜ, etc. See this for more details.
Here is what I am doing:
method : function(input) {
return input.value !== ''
&& input.value === /^[a-zA-Z'- u00c0-u017e]+$/
}
Here, it should match: Åløæ-Bond Mc'Cool
Åløæ-Bond Mc'Cool
But fail: 123-Bond Mc'C@o!
123-Bond Mc'C@o!
When I run ^[a-zA-Z'- u00c0-u017e]+$
in regex tester, It works absolutely fine, but in my script, it is not validating and throws an invalid input error.
^[a-zA-Z'- u00c0-u017e]+$
What am I doing wrong?
What error is coming from your script?
– Aman Chhabra
Jul 3 at 5:30
There's no syntax error in my script. It's just the regex pattern not working.
– CodeMonk
Jul 3 at 5:44
3 Answers
3
I prefer to use RegExp
. You also need to do return pattern.test(input)
RegExp
return pattern.test(input)
This will work :)
var test1 = "Åløæ-Bond Mc'Cool";
var test2 = "123-Bond Mc'C@o!";
var pattern = new RegExp(/^[a-zA-Z'- u00c0-u017e]+$/);
function regextest(input) {
return input !== '' && pattern.test(input)
}
console.log(regextest(test1))
console.log(regextest(test2))
Using
RegExp
is not necessary, but pattern.test
is ;)– Zim
Jul 3 at 5:44
RegExp
pattern.test
Really? @Zim Wow, I never realized that :) Learn something new every day ;) updated
– Sheshank S.
Jul 3 at 5:45
Thanks it worked out well
– CodeMonk
Jul 3 at 5:58
modify your function to test with regex
var pattern = /^[a-zA-Z'- u00c0-u017e]+$/
var method = function(input) {
return input !== '' &&
pattern.test(input)
}
//your sample strings
console.log(method("Åløæ-Bond Mc'Cool"))
console.log(method("123 - Bond Mc 'C@o!"))
That doesn't work?
– Sheshank S.
Jul 3 at 5:37
Unfortunately, it did not work.
– CodeMonk
Jul 3 at 5:48
Don't know how there are 2 upvotes on this answer.. it doesn't work for me @CodeMonk
– Sheshank S.
Jul 3 at 6:07
I figured out a simpler solution for my question:
method : function(input) {
return input.value !== '' && /^[a-zA-Z'- u00c0-u017e]+$/.test(input.value)
}
The problem was, after the &&
operator, I was checking for the input value (that’s wrong in regex check) instead of Boolean value.
&&
This solution works perfectly and creates no confusion.
pattern.test
is the standard way, it doesn't create any confusion..– Sheshank S.
Jul 5 at 15:06
pattern.test
You’re right. I’m only referring to the readability referring to my own question
– CodeMonk
Jul 5 at 17:08
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Would be helpful to have the error from your script
– Sheshank S.
Jul 3 at 5:30