Canvas background image clears when drawing over it
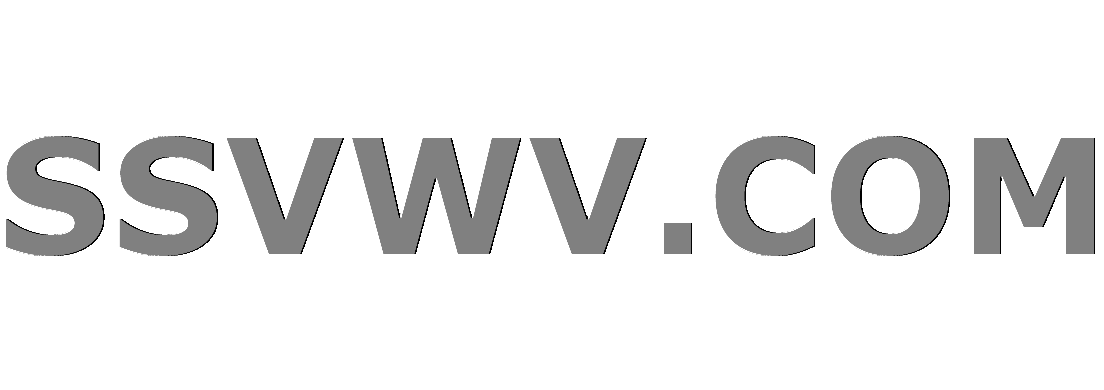
Multi tool use
Canvas background image clears when drawing over it
The application I'm attempting to write has a user upload an image to a canvas and then draw lines over it. So far I have the uploading to the canvas down as well as the drawing of the lines, except that whenever I draw a line on the canvas, the uploaded image disappears. Below you'll see the html and javascript code I currently have for the app. The various elements were obtained from various tutorials so i'm assuming there is some incompatibility that they are overwriting each other.
HTML
<input type='file' id="fileUpload">
<canvas id="c" width = 750 height= 400 style="border:1px solid #ccc"></canvas>
Javascript
// begin file upload block
function el(id) { return document.getElementById(id); }
var canvas = el("c");
var context = canvas.getContext("2d");
function readImage() {
if (this.files && this.files[0]) {
var FR = new FileReader();
FR.onload = function (e) {
var img = new Image();
img.onload = function () {
context.drawImage(img, 0, 0,img.width,img.height,0,0,750,400);
};
img.src = e.target.result;
};
FR.readAsDataURL(this.files[0]);
}
}
el("fileUpload").addEventListener("change", readImage, false);
//end file upload block
//begin line drawing block
var canvas = new fabric.Canvas('c', { selection: false });
var line, isDown;
canvas.on('mouse:down', function(o){
isDown = true;
var pointer = canvas.getPointer(o.e);
var points = [ pointer.x, pointer.y, pointer.x, pointer.y ];
line = new fabric.Line(points, {
strokeWidth: 5,
fill: 'red',
stroke: 'red',
originX: 'center',
originY: 'center'
});
canvas.add(line);
});
canvas.on('mouse:move', function(o){
if (!isDown) return;
var pointer = canvas.getPointer(o.e);
line.set({ x2: pointer.x, y2: pointer.y });
canvas.renderAll();
});
canvas.on('mouse:up', function(o){
isDown = false;
});
//end line drawing block
1 Answer
1
Use fabric.Image.fromURL
to load image and then add to canvas using canvas.add()
.
fabric.Image.fromURL
canvas.add()
DEMO
//begin line drawing block
var canvas = new fabric.Canvas('c', {
selection: false
});
var line, isDown;
canvas.on('mouse:down', function(o) {
isDown = true;
var pointer = canvas.getPointer(o.e);
var points = [pointer.x, pointer.y, pointer.x, pointer.y];
line = new fabric.Line(points, {
strokeWidth: 5,
fill: 'red',
stroke: 'red',
originX: 'center',
originY: 'center'
});
canvas.add(line);
});
canvas.on('mouse:move', function(o) {
if (!isDown) return;
var pointer = canvas.getPointer(o.e);
line.set({
x2: pointer.x,
y2: pointer.y
});
canvas.renderAll();
});
canvas.on('mouse:up', function(o) {
isDown = false;
});
//end line drawing block
// begin file upload block
function el(id) {
return document.getElementById(id);
}
function readImage() {
if (this.files && this.files[0]) {
var FR = new FileReader();
FR.onload = function(e) {
fabric.Image.fromURL(e.target.result, function(img) {
img.set({
left: 0,
top: 0,
evented: false
});
img.scaleToWidth(canvas.width);
img.setCoords();
canvas.add(img);
})
};
FR.readAsDataURL(this.files[0]);
}
}
el("fileUpload").addEventListener("change", readImage, false);
//end file upload block
https://rawgit.com/kangax/fabric.js/master/dist/fabric.js
<input type='file' id="fileUpload">
<canvas id="c" width = 750 height= 400 style="border:1px solid #ccc"></canvas>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.