Expand UITableViewCell UIImage
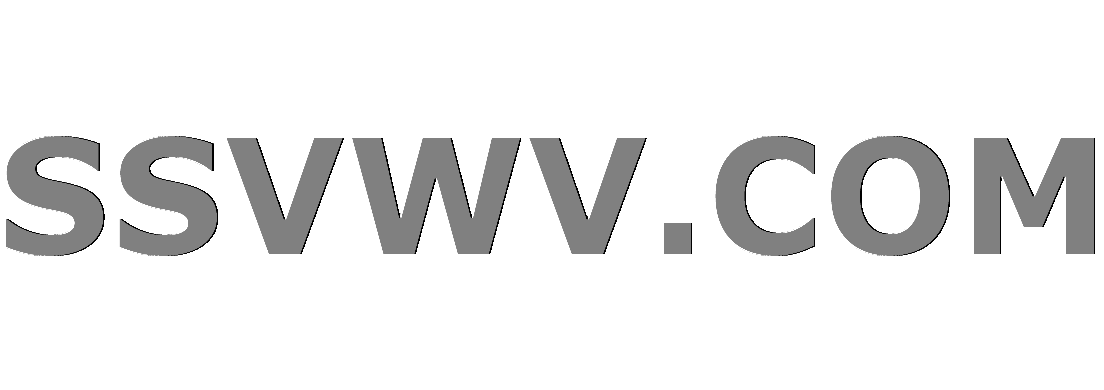
Multi tool use
Expand UITableViewCell UIImage
I have a UITableViewCell
with two images, my goal is to expand these images upon a long press by the user. In the best case scenario the image would cover the entire screen with a small 'x' or something to close.
UITableViewCell
I have the following function I'm using within the custom UITableViewCell
, but the image only expands the size of the cell. I can't figure out how to expand the image over the entire tableview/navBar/tabbar of the superview.
UITableViewCell
@objc func answerOneLongPress(_ sender: UILongPressGestureRecognizer) {
let imageView = sender.view as! UIImageView
let newImageView = UIImageView(image: imageView.image)
let screenSize = UIScreen.main.bounds
let screenWidth = screenSize.width
let screenHeight = screenSize.height
newImageView.frame = CGRect(x: 0, y: 0, width: screenWidth, height: screenHeight)
newImageView.backgroundColor = .black
newImageView.contentMode = .scaleAspectFit
self.addSubview(newImageView)
}
Please let me know if you need more information. I feel like this should be happening in the UITableViewController
as opposed to the cell, but haven't been able to get it working that way.
UITableViewController
can you add a gif which shows whats going wrong?
– Akshaysingh Yaduvanshi
Jul 3 at 6:20
@RahulGUsai care to give some example or more information?
– Casey West
Jul 3 at 6:22
like this github.com/jeantimex/ios-swift-collapsible-table-section
– Devil Decoder
Jul 3 at 6:28
What is
self
in this context to which you add a subview? You will need to add this subview to a view controller's view or event to a window UIApplication.shared.keyWindow
. If this is a cell then your image view is being clipped and is even positioned incorrectly.– Matic Oblak
Jul 3 at 6:28
self
UIApplication.shared.keyWindow
1 Answer
1
You should not add your view to cell but to a view controller or to a key window. That depends on your needs. what happens in your case is your image view is added on a cell and is being clipped and also it is not positioned correctly.
I would use some kind of object that handles presenting of this image. Let the code speak for itself:
class ImageOverlayController {
private var startFrame: CGRect
private var backgroundView: UIView
private var imageView: UIImageView
private init(startFrame: CGRect, backgroundView: UIView, imageView: UIImageView) {
self.startFrame = startFrame
self.backgroundView = backgroundView
self.imageView = imageView
}
private convenience init() { self.init(startFrame: .zero, backgroundView: UIView(), imageView: UIImageView()) }
static func showPopupImage(inController viewController: UIViewController? = nil, fromImageView imageView: UIImageView) -> ImageOverlayController {
guard let targetView = viewController?.view ?? UIApplication.shared.keyWindow else { return ImageOverlayController() } // This should never happen
let startFrame = imageView.convert(imageView.bounds, to: targetView)
let backgroundView: UIView = {
let view = UIView(frame: targetView.bounds)
view.backgroundColor = UIColor.black.withAlphaComponent(0.0)
return view
}()
let newImageView: UIImageView = {
let view = UIImageView(frame: startFrame)
view.image = imageView.image
return view
}()
let controller = ImageOverlayController(startFrame: startFrame, backgroundView: backgroundView, imageView: imageView)
backgroundView.addSubview(newImageView)
targetView.addSubview(backgroundView)
UIView.animate(withDuration: 0.3) {
backgroundView.backgroundColor = UIColor.black.withAlphaComponent(0.5)
newImageView.frame = targetView.bounds
}
return controller
}
func dimiss(completion: (() -> Void)? = nil) {
UIView.animate(withDuration: 0.3, animations: {
self.imageView.frame = self.startFrame
self.backgroundView.backgroundColor = self.backgroundView.backgroundColor?.withAlphaComponent(0.0)
}) { _ in
self.backgroundView.removeFromSuperview()
completion?()
}
}
}
As you say a button must still be added which may then call dismiss on the view.
Note: The code I provided was not really tested but just quickly put together. Let me know if there are any issues so I modify it.
Thank you so incredibly much. I will give it a test. Sorry for the noob question, but would you also be able to provide a little guidance on how to utilize this class from a UITableViewController?
– Casey West
Jul 3 at 7:04
@CaseyWest What do you mean? This is a static method and can be used with anything. You can call it in a cell or anywhere simply
ImageOverlayController. showPopupImage(fromImageView: myImageView)
So what is the difference in UITableViewController
?– Matic Oblak
Jul 3 at 7:09
ImageOverlayController. showPopupImage(fromImageView: myImageView)
UITableViewController
it is working great to show the image. The only issue I've found is if you press and hold (keep holding) it will infinitely try to show the image, probably caused by something in my cell. The expanding animation happens until you release the button. Working on adding the exit button currently. Thanks!!
– Casey West
Jul 3 at 7:26
@CaseyWest A long press gesture is the same as pan gesture but needs to be held down to trigger. What you are missing is checking the gesture recognizer state. In your case it needs to be
.begin
to trigger the image showing. What happens in your case is that if you keep moving your finger the method will keep triggering for state .change
and when you let go it also triggers for .ended
. So all you need is an if
statement there.– Matic Oblak
Jul 3 at 7:49
.begin
.change
.ended
if
Okay I added an if statement and it isn't spamming anymore. I am having trouble with the dismissal. Is it possible for you to include an appended version of my function in the original question, which utilizes your class? That would be very helpful, and sorry I'm fairly new at this.
– Casey West
Jul 3 at 19:57
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
you can use collapsible tableview on longpress just reload particular sections
– Devil Decoder
Jul 3 at 6:15