Firebase Database Not Loading in TableView
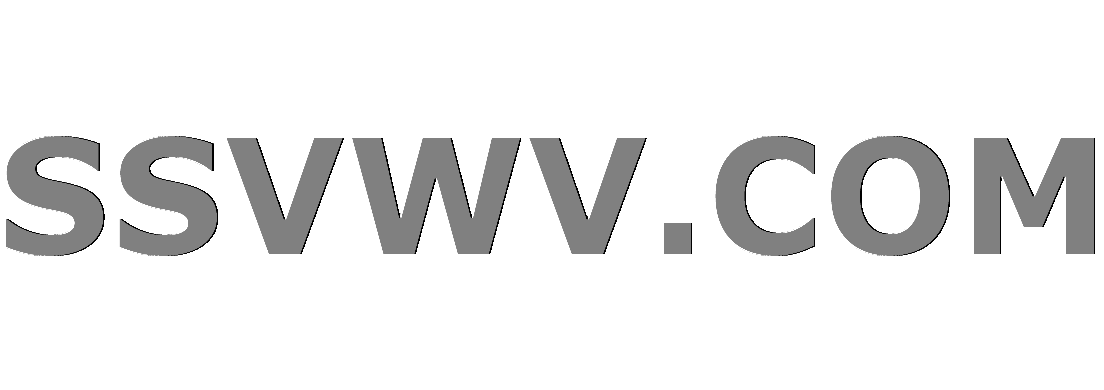
Multi tool use
Firebase Database Not Loading in TableView
For the iOS project that I am working on, I am using Firebase as my database. I have multiple pages each with their own reference, and they are working just fine. But when I created my most recent page, nothing is loaded when the app is running. The tableview is blank, but there are no error messages. Is there something wrong with the way that I am referencing the database?
Here is the code:
import UIKit
import Firebase
class wrestlingController: UIViewController, UITableViewDelegate, UITableViewDataSource {
var ref = DatabaseReference()
var wDate = [String]()
var wTime = [String]()
var wMatch = [String]()
var databaseHandle:DatabaseHandle = 0
var databaseHandle2:DatabaseHandle = 0
var databaseHandle3:DatabaseHandle = 0
@IBOutlet var wrestlingTable: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
ref = Database.database().reference()
databaseHandle = ref.child("Wrestle").child("wDates").observe(.childAdded) { (snapshot) in
let post = snapshot.value as? String
if let actualPost = post {
self.wDate.append(actualPost)
}
}
databaseHandle2 = ref.child("Wrestle").child("wTimes").observe(.childAdded) { (snapshot) in
let post2 = snapshot.value as? String
if let actualPost2 = post2{
self.wTime.append(actualPost2)
}
}
databaseHandle3 = ref.child("Wrestle").child("wMatches").observe(.childAdded) { (snapshot) in
let post3 = snapshot.value as? String
if let actualPost3 = post3 {
self.wMatch.append(actualPost3)
self.wrestlingTable.reloadData()
}
}
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return wMatch.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let wrestlingCell = tableView.dequeueReusableCell(withIdentifier: "wrestlingCell") as! wrestlingCell
wrestlingCell.wDate.text = wDate[indexPath.row]
wrestlingCell.wTime.text = wTime[indexPath.row]
wrestlingCell.wMatch.text = wMatch[indexPath.row]
return(wrestlingCell)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
reloadData
reloadData()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Firebase returns from a background thread and you should consider putting your
reloadData
inside the main thread for starters. And i assume you have debugged the response to check if there is actually any data being returned and since you are callingreloadData()
inside just one closure, its a race condition depending on wether other closures have returned their fetch by the time its called. Consider reloading inside every closure as a quick check for your problem.– Rikh
Jul 3 at 4:30