How to handle with dropdown menu on Phptravels.net website using selenium
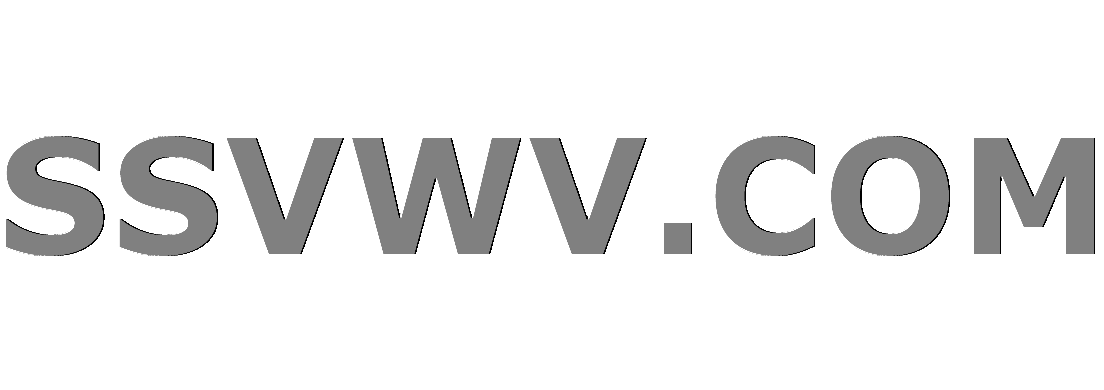
Multi tool use
How to handle with dropdown menu on Phptravels.net website using selenium
I have some big problem with handle with dropdown menu on https://www.phptravels.net/ website.
I wrote this test, but it doesn't work and I don't have idea how to do it correctly.
public class HomePage {
private Logger logger = LogManager.getRootLogger();
@FindBy(xpath = "//*[@id="li_myaccount"]/ul")
private WebElement dropDownMenu;
@FindBy(xpath = "//*[@id="li_myaccount"]/ul/li")
private WebElement dropDownMenuOptions;
public HomePage() {
PageFactory.initElements(DriverManager.getWebDriver(), this);
}
public void clickOnMyAccountDropMenuAndSelectOption(String option) {
WaitForElement.waitUntilElementIsVisible(dropDownMenu);
dropDownMenu.click();
List<WebElement> options = DriverManager.getWebDriver().findElements(By.xpath("//*[@id="li_myaccount"]/ul/li"));
for(WebElement o: options) {
if(o.getText().equals(option)) {
o.click();
return;
}
}
}
public void clickOnLoginLink() {
WaitForElement.waitUntilElementIsClickable(loginLink);
loginLink.click();
logger.info("Clicked on Login link");
}
}
My question is that how should I change clickOnMyAccountDropMenuAndSelectOption method to make test correct? Thanks for your help!
2 Answers
2
Try this:
public class HomePage {
private Logger logger = LogManager.getRootLogger();
@FindBy(xpath = "//nav//*[@id='li_myaccount']/a")
private WebElement dropDownMenu;
@FindBy(xpath = "//nav//*[@id='li_myaccount']/ul/li/a")
private WebElement dropDownMenuOptions;
public HomePage() {
PageFactory.initElements(DriverManager.getWebDriver(), this);
}
public void clickOnMyAccountDropMenuAndSelectOption(String option) {
WaitForElement.waitUntilElementIsVisible(dropDownMenu);
dropDownMenu.click();
Thread.sleep(2000); // wait 2 seconds until dropdown loads
List<WebElement> options = DriverManager.getWebDriver().findElements(By.xpath("//nav//*[@id='li_myaccount']/ul/li/a"));
for(WebElement o: options) {
if(o.getText().equals(option)) {
o.click();
return;
}
}
}
public void clickOnLoginLink() {
WaitForElement.waitUntilElementIsClickable(loginLink);
loginLink.click();
logger.info("Clicked on Login link");
}
}
Your xPaths
were not correct. I have fixed them.
xPaths
You need to change some of the locators :
It's always a good practice to use css selector over xpath.
For this :
@FindBy(xpath = "//*[@id="li_myaccount"]/ul")
private WebElement dropDownMenu;
Use this :
@FindBy(css= "li#li_myaccount>a[aria-expanded]")
private WebElement dropDownMenu;
For this :
@FindBy(xpath = "//*[@id="li_myaccount"]/ul/li")
private WebElement dropDownMenuOptions;
Use this :
@FindBy(css= "div#collapse ul.navbar-right li#li_myaccount li>a")
private WebElement dropDownMenuOptions;
And in this method use it like :
List<WebElement> options = DriverManager.getWebDriver().findElements(By.cssSelector("div#collapse ul.navbar-right li#li_myaccount li>a"));
for(WebElement o: options) {
if(o.getText().trim().contains("Login")) {
o.click();
}
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
can you describe what output you are getting? Where/what is the error; have you verified your FindBy's?
– Ywapom
Jul 2 at 20:11