Python - Selenium: How to click on the element with text as “Year” within the webpage
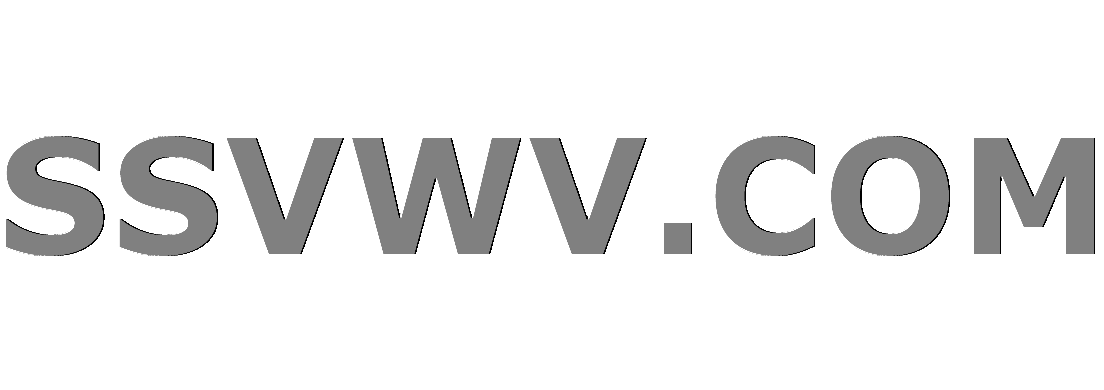
Multi tool use
Python - Selenium: How to click on the element with text as “Year” within the webpage
from bs4 import BeautifulSoup as soup
from openpyxl import load_workbook
from openpyxl.styles import PatternFill, Font
from selenium import webdriver
from selenium.webdriver.common.by import By
import datetime
import os
import time
import re
browser = webdriver.Chrome(executable_path=r"C:Program Files(x86)GoogleChromeApplicationchromedriver.exe")
my_url = 'https://www.eex.com/en/market-data/power/futures/phelix-at-futures#!/2017/07/24'
browser.get(my_url)
button = browser.find_elements_by_class_name('ng-scope')[-1]
browser.execute_script("arguments[0].click();", button)
It does not give me any error but it is not actually clicking. It should change from month to year, since it is the last element, but it stays there.
'Year' button, using inspect seems to be the last 'ng-scope' element
– Davide Ravera
Jul 3 at 8:19
3 Answers
3
As per the url you have provided to click on the element with text as Year you have to induce WebDriverWait for the element to be clickable as follows:
CSS_SELECTOR
:
CSS_SELECTOR
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.CSS_SELECTOR, "ul.tabs.filter_wrap.clearfix li.ng-scope:nth-child(3)>a"))).click()
XPATH
:
XPATH
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//ul[@class='tabs filter_wrap clearfix']//li[@class='ng-scope']/a[contains(.,'Year')]"))).click()
It's working fine, thank you
– Davide Ravera
Jul 3 at 8:34
Try this:
from bs4 import BeautifulSoup as soup
from openpyxl import load_workbook
from openpyxl.styles import PatternFill, Font
from selenium import webdriver
from selenium.webdriver.common.by import By
import datetime
import os
import time
import re
browser = webdriver.Chrome(executable_path=r"C:Program Files(x86)GoogleChromeApplicationchromedriver.exe")
my_url = 'https://www.eex.com/en/market-data/power/futures/phelix-at-futures#!/2017/07/24'
browser.get(my_url)
# wait until button will be present
WebDriverWait(driver, 120).until(EC.presence_of_element_located((By.XPATH, "//*[@id='content']/div/div/ul/li[3]/a")))
# find the button and click on it
button = driver.find_element_by_xpath("//*[@id='content']/div/div/ul/li[3]/a")
button.click()
You can peel the same apple in different ways. However, in this very case the better approach would be to use .find_element_by_link_text()
which is less likely to break. Give it a shot:
.find_element_by_link_text()
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
url = 'https://www.eex.com/en/market-data/power/futures/phelix-at-futures#!/2017/07/24'
driver = webdriver.Chrome()
driver.get(url)
wait = WebDriverWait(driver, 30)
wait.until(EC.presence_of_element_located((By.LINK_TEXT, "Year"))).click()
driver.quit()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Which button are you trying to click?
– DebanjanB
Jul 3 at 8:15