Proper syntax for Laravel Eloquent insert/update
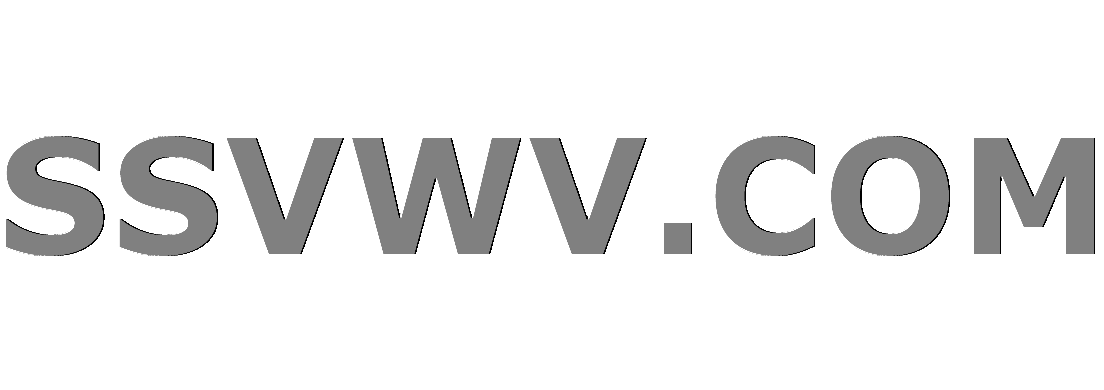
Multi tool use
Proper syntax for Laravel Eloquent insert/update
I have seemingly simple problem that I still can't solve after days of browsing around.
Say I have Debitur model.
class Debitur extends Model
{
protected $table = 'debiturs';
protected $fillable = [
'name', 'address',
];
public function debiturWife(){
return $this->hasOne('AppDebiturWife');
}
}
And I have a DebiturWife model.
class DebiturWife extends Model
{
protected $table = 'debitur_wifes';
protected $fillable = [
'nama', 'address', 'photo',
];
public function debitur(){
return $this->belongsTo('AppDebitur');
}
}
I can get Debitur and DebiturWife data in the DebiturController like this:
return Debitur::with('debiturWife')->find($debitur_id);
Now I want to create a new Debitur and DebiturWife, how do I do that?
Something like
$debitur = new Debitur;
$debitur->name = $request->name;
$debitur_wife = new Debitur.DebiturWife; //obviously doesn't work
Debitur ( id | name | address ). DebiturWife (id | debitur_id | name | address | photo ).
– Rizki Hadiaturrasyid
Jul 3 at 4:25
3 Answers
3
$debitur = new Debitur;
$debitur->name = $request->name;
$debitur->save();
$debitur_wife = new DebiturWife([
'name' => 'Foo'
]);
$debitur->debiturWife()->save($debitur_wife);
Documentation
What if later I want to update DebiturWife based on Debitur's id?
$debitur = Debitur::with('debiturWife')->find($id);
$debitur->debiturWife->name = 'new name';
$debitur->debiturWife->address = 'new address';
$debitur->save();
Reason for downvote?
– linktoahref
Jul 3 at 5:12
What if later I want to update DebiturWife based on Debitur's id? I tried this but doesn't work $debitur = Debitur::with('debiturWife')->find($id); $debiturWife = debiturWife::where('debitur_id',$id)->update($request->all()); $debitur->debiturWife()->save($debiturWife);
– Rizki Hadiaturrasyid
Jul 3 at 6:42
The documentation is confusing, I have read it multiple times.
– Rizki Hadiaturrasyid
Jul 3 at 6:43
@RizkiHadiaturrasyid Check out the updated answer
– linktoahref
Jul 3 at 7:08
Thank you very much!
– Rizki Hadiaturrasyid
Jul 3 at 8:24
You could do this way:
$debitur = new Debitur;
$debitur->name = $request->name;
$debitur->save();
$debitur->debiturWife()->create([
'name' => $request->name,
'address' => $request->address,
'photo' => $request->photo
]);
Another way of doing this would be —
$debiturWife = new DebiturWife();
$debiturWife->name = $request->name;
$debiturWife->address = $request->address;
$debiturWife->photo = $request->photo;
$debitur->debiturWife()->save($debiturWife);
The debitur_id
will be automatically set. The only difference between the create
& save
method is that the create
method accepts a plain PHP array whereas the save
method accepts an instance of DebitureWife
debitur_id
create
save
create
save
DebitureWife
See the documentation for better understanding.
One way is to use create
:
create
$debitur = Debitur::create([
'name' => $request->name
]);
$debiturWife = DebiturWife::create([
'name' => $request->wife_name,
'debitur_id' => $debitur->getKey()
]);
// chained together
Debitur::create([
'name' => $request->name
])->debiturWife()->save(new DebiturWife([
'name' => $request->wife_name
]));
If using mass assignment on the DebiturWife model, you will need to include debitur_id
in the $fillable
array:
debitur_id
$fillable
protected $fillable = [
'nama', 'address', 'photo', 'debitur_id'
];
Or, using the Query Builder:
$id = DB::table('debiturs')->insertGetId([
'name' => $request->name
]);
DB::table('debitur_wifes')->insert([
'name' => $request->wife_name,
'debitur_id' => $id
]);
Updating a related model:
Debitur::find($id)->debiturWife()->update(['name' => 'felicia']);
method DebiturWife::create() still undefined?
– Rizki Hadiaturrasyid
Jul 3 at 4:40
It shouldn't be undefined. It's defined on the query builder class: laravel.com/api/5.6/Illuminate/Database/Eloquent/…
– DigitalDrifter
Jul 3 at 4:46
oh I forgot to add the use AppDebiturWife because I put the controller inside API folder.
– Rizki Hadiaturrasyid
Jul 3 at 4:50
So it tries to find it inside the API folder instead, which isn't found.
– Rizki Hadiaturrasyid
Jul 3 at 4:50
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What are the schemas for Debitur and DebiturWife database tables?
– Adre Astrian
Jul 3 at 4:22