Python Function to Fractionize digits of many tuples contained within a list
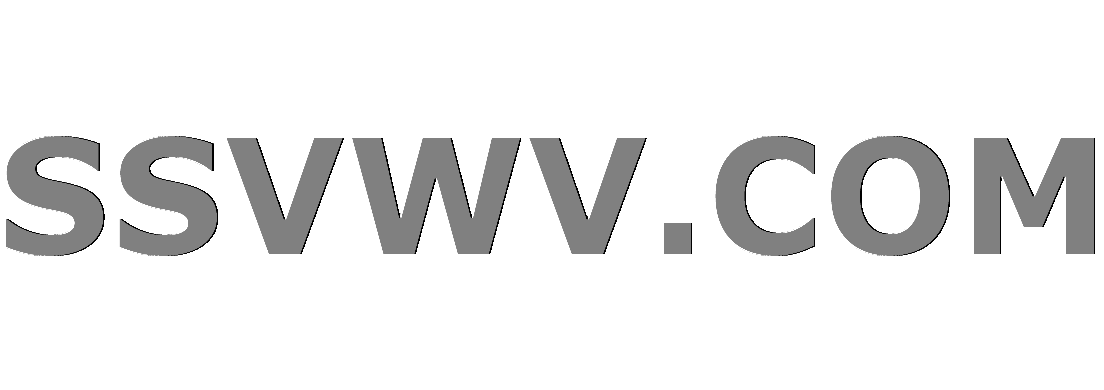
Multi tool use
Python Function to Fractionize digits of many tuples contained within a list
> AA = [('JAMES', 124), ('KATE', 78), ('MARIE', 62), ('NGUYEN', 59)]
>
> BB = [('JACK', 133), ('JILL', 68), ('SARAH', 67), ('MARGARITA', 66),
> ('NATE', 66), ('DANI', 55)]
>
> CC =[('MARYLOU', 155), ('PETER', 98), ('ALEXA', 57), ('MARK', 54),
> ('KAREN', 34)]
I have a lot of lists (AA, BB and CC) containing tuples containing a string and corresponding digit.
For each list (such as AA, BB and CC), I want the function to take the highest digit (124 in the list AA) in these tuples as 1, and output the remaining digits of all other tuples in the same list as fraction of the highest digit among the tuples (78/124=0.63). Fractions should be 2 decimal points.
Target Output
AA = [('JAMES', 1), ('KATE', 0.63), ('MARIE', 0.5), ('NGUYEN', 0.48)]
BB = [('JACK', 1), ('JILL', 0.51), ('SARAH', 0.5), ('MARGARITA', 0.5), ('NATE', 0.5), ('DANI', 0.41)]
CC =[('MARYLOU', 1), ('PETER', 0.63), ('ALEXA', 0.37), ('MARK', 0.35), ('KAREN', 0.22)]
Please suggest tips to solve this problem.
So far, I came up with the ranking of tuples inside each list.
def rank(L): #to sort tuples inside the List by the value inside the tuples
L = L.sort(reverse = True, key = lambda x : x[1])
its not homework, I am doing genomic data analysis, can't wrap my head around defining functions for objects inside objects.
– Nguyen
Jul 3 at 4:39
If you don't have any code to show us where you are stuck, check the answer below and tell us what parts you get stuck on. Break the one-liner in to step-by-step parts and put it into a function.
– davedwards
Jul 3 at 4:52
1 Answer
1
Here are some tips:
If you cant get it, this should help you in step 2 and 3:
return map(lambda x : (x[0],)+(round(float(x[1])/list1[0][1],2),),list1)
return map(lambda x : (x[0],)+(round(float(x[1])/list1[0][1],2),),list1)
AA = [('JAMES', 124), ('KATE', 78), ('MARIE', 62), ('NGUYEN', 59)]
def rank(L):
L.sort(reverse = True, key = lambda x : x[1])
return L
def normalize(L):
L = rank(L)
newlist = #The new list
for i in L: #Iterate through the sorted list
newtuple = (i[0],) #Copy the name as is
newtuple += (round(float(i[1])/L[0][1],2),) #Divide by the largest value and round to 2 decimal places
newlist.append(newtuple) #Add it to the new list
return newlist
AA = normalize(AA)
print AA
#[('JAMES', 1.0), ('KATE', 0.63), ('MARIE', 0.5), ('NGUYEN', 0.48)]
hey Rohit, are you from NTU Singapore?
– Nguyen
Jul 3 at 5:51
I added the sorting function. How can I do the step 2: looping through each list? @RohithS98
– Nguyen
Jul 3 at 6:07
No I am not from NTU. Since you are using a list of tuples, you will need to create a new list. Go through the elements of the first list with for loop and copy the name as it is while changing the second value. You can also use list comprehension like in my answer.
– RohithS98
Jul 3 at 6:13
You lost me. can you show example similar question asked on before similar to "Go through the elements of the first list with for loop and copy the name as it is while changing the second value. " @RohithS98
– Nguyen
Jul 3 at 6:22
@Nguyen I added a program which explains each steps in the comments
– RohithS98
Jul 3 at 6:42
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This sounds like homework. Have you started any code that attempts a solution?
– davedwards
Jul 3 at 4:07