What does “Expected BEGIN_ARRAY but was BEGIN_OBJECT” means?
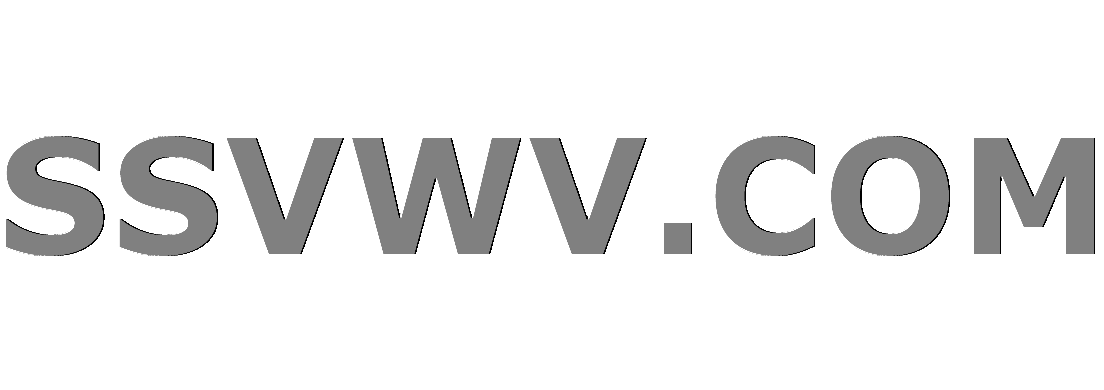
Multi tool use
What does “Expected BEGIN_ARRAY but was BEGIN_OBJECT” means?
I'm facing this very basic issue as I'm parsing according to response. Can some trace where I'm doing some thing wrong. I'm using GSON adapter with retrofit 2.
[
{
"date": "2018-07-17",
"trip": [
{
"id": 381,
"trip_address": "Dubai, United Arab Emirates",
"trip_created_by": "auth0|5a7dafcf5c679b17810dbd88",
"trip_arrival_datetime": "17-07-2018 21:03",
"trip_departure_datetime": null,
"trip_country": null,
"trip_city": null,
"trip_state": null,
"trip_location_longitude": "67.00113640",
"trip_location_latitude": "24.86073430",
"trip_status": "pending",
"created_at": "2018-06-24 12:03:20",
"updated_at": "2018-06-24 12:03:20"
}
]
}
]
My model classes:
Retrofit interface:
@GET("meetup-by-date/{user_id}/{date}")
Single<List<MeetupResponse>> getMeetups(@Path("user_id") String userId, @Path("date") String date);`
Response model:
public class MeetupResponse {
@SerializedName("date")
private String date;
@SerializedName("meetup")
private List<MeetUp> meetups;
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public List<MeetUp> getTrips() {
return meetups;
}
public void setTrips(List<MeetUp> trips) {
this.meetups = trips;
}
}
{ }
[ ]
2 Answers
2
You have a malformed JSON response somewhere.
If you post the full log trace along with the retrofit log of the response body, I could point out where specifically is the problem.
The issue is that you are using wrong key meetup
. change meetup
to trip
.i.e, change @SerializedName("meetup")
to @SerializedName("trip")
meetup
meetup
trip
@SerializedName("meetup")
@SerializedName("trip")
You may use the following pojo classes
public class MeetupResponse {
@SerializedName("date")
@Expose
private String date;
@SerializedName("trip")
@Expose
private List<Trip> trip = null;
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public List<Trip> getTrip() {
return trip;
}
public void setTrip(List<Trip> trip) {
this.trip = trip;
}
}
and this
public class Trip {
@SerializedName("id")
@Expose
private Integer id;
@SerializedName("trip_address")
@Expose
private String tripAddress;
@SerializedName("trip_created_by")
@Expose
private String tripCreatedBy;
@SerializedName("trip_arrival_datetime")
@Expose
private String tripArrivalDatetime;
@SerializedName("trip_departure_datetime")
@Expose
private Object tripDepartureDatetime;
@SerializedName("trip_country")
@Expose
private Object tripCountry;
@SerializedName("trip_city")
@Expose
private Object tripCity;
@SerializedName("trip_state")
@Expose
private Object tripState;
@SerializedName("trip_location_longitude")
@Expose
private String tripLocationLongitude;
@SerializedName("trip_location_latitude")
@Expose
private String tripLocationLatitude;
@SerializedName("trip_status")
@Expose
private String tripStatus;
@SerializedName("created_at")
@Expose
private String createdAt;
@SerializedName("updated_at")
@Expose
private String updatedAt;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getTripAddress() {
return tripAddress;
}
public void setTripAddress(String tripAddress) {
this.tripAddress = tripAddress;
}
public String getTripCreatedBy() {
return tripCreatedBy;
}
public void setTripCreatedBy(String tripCreatedBy) {
this.tripCreatedBy = tripCreatedBy;
}
public String getTripArrivalDatetime() {
return tripArrivalDatetime;
}
public void setTripArrivalDatetime(String tripArrivalDatetime) {
this.tripArrivalDatetime = tripArrivalDatetime;
}
public Object getTripDepartureDatetime() {
return tripDepartureDatetime;
}
public void setTripDepartureDatetime(Object tripDepartureDatetime) {
this.tripDepartureDatetime = tripDepartureDatetime;
}
public Object getTripCountry() {
return tripCountry;
}
public void setTripCountry(Object tripCountry) {
this.tripCountry = tripCountry;
}
public Object getTripCity() {
return tripCity;
}
public void setTripCity(Object tripCity) {
this.tripCity = tripCity;
}
public Object getTripState() {
return tripState;
}
public void setTripState(Object tripState) {
this.tripState = tripState;
}
public String getTripLocationLongitude() {
return tripLocationLongitude;
}
public void setTripLocationLongitude(String tripLocationLongitude) {
this.tripLocationLongitude = tripLocationLongitude;
}
public String getTripLocationLatitude() {
return tripLocationLatitude;
}
public void setTripLocationLatitude(String tripLocationLatitude) {
this.tripLocationLatitude = tripLocationLatitude;
}
public String getTripStatus() {
return tripStatus;
}
public void setTripStatus(String tripStatus) {
this.tripStatus = tripStatus;
}
public String getCreatedAt() {
return createdAt;
}
public void setCreatedAt(String createdAt) {
this.createdAt = createdAt;
}
public String getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
}
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
BEGIN_OBJECT means it found
{ }
before it found[ ]
It means that your response is trying to parse an array but the level failing is actually an object– inner_class7
Jul 2 at 20:48