Laravel - using CacheManager as a dependency
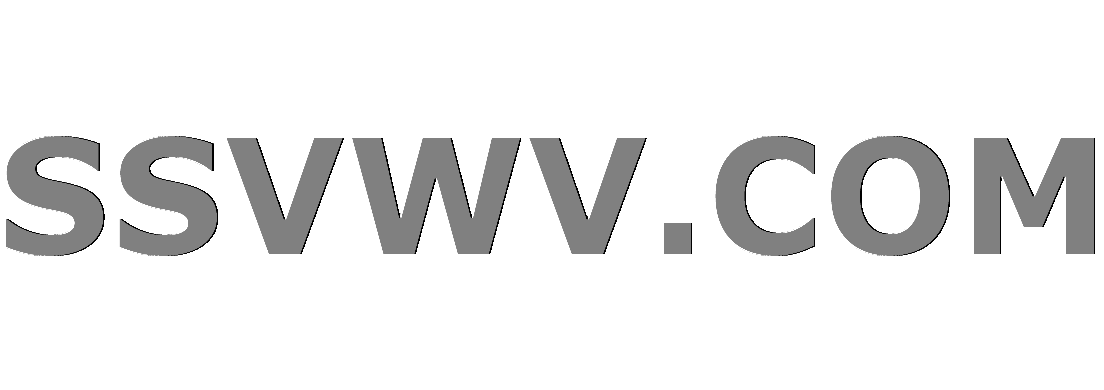
Multi tool use
Laravel - using CacheManager as a dependency
I am trying to use CacheManager
class as a dependency in my service:
CacheManager
<?php
declare(strict_types=1);
namespace App;
use GuzzleHttpClient;
use IlluminateCacheCacheManager;
class MatrixWebService implements WebServiceInterface
{
public function __construct(Client $client, CacheManager $cache)
{
$this->client = $client;
$this->cache = $cache;
}
}
Because there can be multiple implementations of WebserviceInterface
I need to define it in the AppServiceProvider
accordingly:
WebserviceInterface
AppServiceProvider
$this->app->bind(WebServiceInterface::class, function () {
return new MatrixWebService(
new Client(),
$this->app->get(CacheManager::class)
);
});
The problem is when I am trying to use my service, Laravel is not able to resolve CacheManager
class (there is some substitution for cache
instead):
CacheManager
cache
[2018-07-02 22:45:26] local.ERROR: Class cache does not exist {"exception":"[object] (ReflectionException(code: -1): Class cache does not exist at /var/www/html/vendor/laravel/framework/src/Illuminate/Container/Container.php:769)
[stacktrace]
#0 /var/www/html/vendor/laravel/framework/src/Illuminate/Container/Container.php(769): ReflectionClass->__construct('cache')
#1 /var/www/html/vendor/laravel/framework/src/Illuminate/Container/Container.php(648): IlluminateContainerContainer->build('cache')
#2 /var/www/html/vendor/laravel/framework/src/Illuminate/Container/Container.php(610): IlluminateContainerContainer->resolve('cache')
#3 /var/www/html/app/Providers/AppServiceProvider.php(28): IlluminateContainerContainer->get('Illuminate\Cach...')
...
Any ideas on how to approach this correctly?
1 Answer
1
When your application has multiple implementations of an interface, contextual binding is useful:
namespace App;
use GuzzleHttpClient;
use CacheRepository;
class MatrixWebService implements WebServiceInterface
{
public function __construct(Client $client, Repository $cache)
{
$this->client = $client;
$this->cache = $cache;
}
}
// AppServiceProvider.php
public function register()
{
$this->app->bind(WebServiceInterface::class, MatrixWebServiceInterface::class);
// bind MatrixWebService
$this->app->when(MatrixWebService::class)
->needs(Repository::class)
->give(function () {
return app()->makeWith(CacheManager::class, [
'app' => $this->app
]);
});
// bind some other implementation of WebServiceInterface
$this->app->when(FooService::class)
->needs(Repository::class)
->give(function () {
return new SomeOtherCacheImplementation();
});
}
use IlluminateContractsConfigRepository;
CacheRepository
$this->app->bind(WebServiceInterface::class, MatrixWebServiceInterface::class);
MatrixWebService
Done. Did the contextual binding work for you?
– DigitalDrifter
Jul 4 at 2:20
Currently just using it for different environments. But will definitely use it in the future. Thanks!
– falnyr
Jul 4 at 3:09
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Can you please change
use IlluminateContractsConfigRepository;
toCacheRepository
and add$this->app->bind(WebServiceInterface::class, MatrixWebServiceInterface::class);
beforeMatrixWebService
definition? I will accept the answer then.– falnyr
Jul 4 at 0:30