VHDL initalize signed of variable length to maximum value
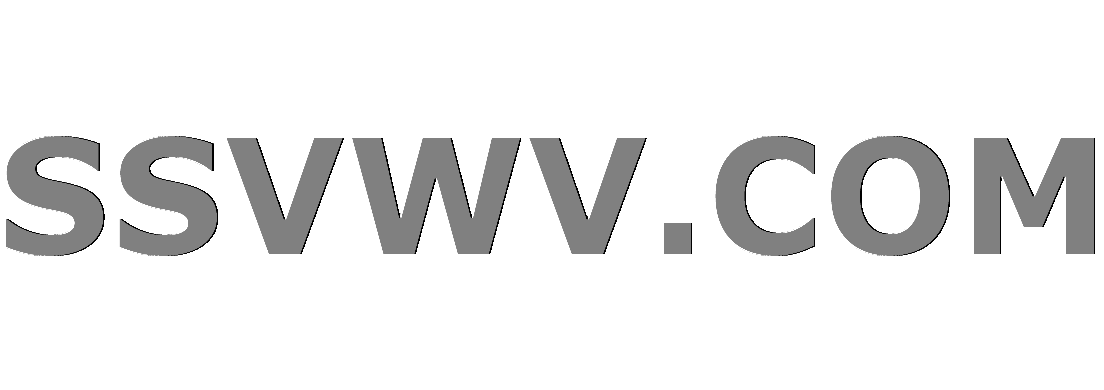
Multi tool use
VHDL initalize signed of variable length to maximum value
I've got the following function that returns the value variable when it can be represented with the length given as a parameter. If value is out of limits, it should return the maximum possible value or the maximum negative value depending on the sign of value.
function truncate (
value : in signed;
length : in integer)
return signed is
constant max_positive_value : signed(length-1 downto 0) := ((length-1) => '0', others => '1');
constant max_negative_value : signed(length-1 downto 0) := ((length-1) => '1', others => '0');
variable return_value : signed(length-1 downto 0) := (others => '0');
begin
if (value >= max_positive_value) then
return_value := max_positive_value;
elsif (value <= max_negative_value) then
return_value := max_negative_value;
else
return_value := resize(value, length);
end if;
return return_value;
end;
The problem is the initialization of the max_positive_value
and max_negative_value
. GHDL complains about not static choice exclude others choice
.
How can I initalize the limit values if the length is variable?
I'm using VHDL 93.
max_positive_value
max_negative_value
not static choice exclude others choice
3 Answers
3
Fortunately, since your odd-man-out is on the left, you can do this:
constant max_positive_value : signed(length-1 downto 0) := ('0', others => '1');
constant max_negative_value : signed(length-1 downto 0) := ('1', others => '0');
https://www.edaplayground.com/x/64S7
The LRM says
Apart from a final element association with the single choice others,
the rest (if any) of the element associations of an array aggregate
shall be either all positional or all named. A named association of an
array aggregate is allowed to have a choice that is not locally
static, or likewise a choice that is a null range, only if the
aggregate includes a single element association and this element
association has a single choice. An others choice is locally static if
the applicable index constraint is locally static.
which is as clear as mud.
Non-locally static OTHERS choice is allowed only if it is the only choice of the only association.
Before posting my answer, I tried it on two other simulators and it just so happens that I found two that were both happy. I've just tried another and it has the same opinion as Modelsim. I must be down to the startling clarity of the LRM.
– Matthew Taylor
Jul 3 at 9:17
The EDA playground code the function call parameter for length is a locally static expression (
V5 := truncate(V, 5);
). The constant declarations in the function declarative part are elaborated at run time following elaboration of the parameter list. Note that this is a Modelsim warning unless using -pendanticerrors (see verror for the particular not-provided message number). -IEEE Std 10761993 7.3.3 Function calls "Evaluation of a function call includes evaluation of the actual parameter expressions specified in the call...".– user1155120
Jul 5 at 0:33
V5 := truncate(V, 5);
2.1 Subprogram declarations, 2.1.1 Formal parameters, 2.1.1.1 Constant and variable parameters, "For a nonforeign subprogram having a parameter of a scalar type or an access type, the parameter is passed by copy." How that copying is done isn't defined while 7.3.3 says the actual parameter expression is evaluated.
– user1155120
Jul 5 at 0:41
s/others/(length-2 downto 0)/ but Katu's answer is less hacky
– Brian Drummond
Jul 6 at 15:09
I found another way to do it that Modelsim accepts without a warning:
constant max_positive_value : signed(length-1 downto 0) := to_signed(2**(length-1)-1, length);
constant max_negative_value : signed(length-1 downto 0) := to_signed(-2**(length-1), length);
The other way to do it is to declare a constant locally to work around the good old "not locally static" VHDL issues:
constant C_LEN : integer := length
constant max_negative_value : signed(length-1 downto 0) := ((C_LEN-1) => '1', others => '0');
This doesn't avoid the warning message compiling with Quartus vcom
– Katu
Jul 10 at 15:16
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This fixed the error in GHDL but modelsim vcom still shows a warning
Non-locally static OTHERS choice is allowed only if it is the only choice of the only association.
– Katu
Jul 3 at 8:55