How do I display more JSON items in HTML using WebKit?
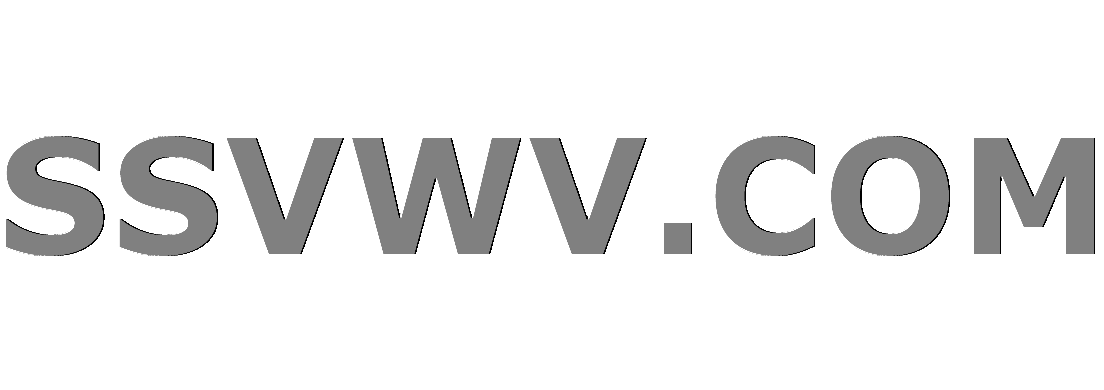
Multi tool use
How do I display more JSON items in HTML using WebKit?
I am building a news app using SwiftyJSON and I have been able to pull the data properly. I am also able to display the title and description in a tableView. However, when I go to the Detail View I want to be able to display the full article summary from the feed.
Here are the feed elements:
func parse(json: JSON) {
for article in json["articles"].arrayValue {
let title = article["title"].stringValue
let author = article["author"].stringValue
let date = article["publishedAt"].stringValue
let image = article["urlToImage"].stringValue
let description = article["description"].stringValue
let obj = ["title": title, "author": author, "date": date, "image": image, "description": description, ]
news.append(obj)
}
I am sending the data to the Detail View Controller as follows:
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let vc = DetailViewController()
vc.articleSummary = news[indexPath.row]
navigationController?.pushViewController(vc, animated: true)
}
Then on the Detail View Controller here is the code. The commented items are items that I would like to add to the display:
import UIKit
import WebKit
class DetailViewController: UIViewController {
var webView: WKWebView!
var articleSummary: [String: String]!
override func loadView() {
webView = WKWebView()
view = webView
}
override func viewDidLoad() {
super.viewDidLoad()
guard articleSummary != nil else { return }
if let description = articleSummary["description"] {
var html = "<html>"
html += "<head>"
html += "<meta name="viewport" content="width=device-width, initial-scale=1">"
html += "<style> body { font-size: 150%; } </style>"
html += "</head>"
html += "<body>"
// html += <h1>title</h1>
// html += <h4>author</h4>
// html += <p>date</p>
// html += <img src="image" alt="" />
html += description
html += "</body>"
html += "</html>"
webView.loadHTMLString(html, baseURL: nil)
}
}
}
what is the problem with your code? is it throwing an error when you uncomment those lines?
– Christian Abella
Jul 3 at 0:02
If I uncomment the code it show as the word not the value.
– fmz
Jul 3 at 0:11
1 Answer
1
You just need to escape the HTML string properly and your data will be displayed.
if let description = articleSummary["description"],
let title = articleSummary["title"],
let author = articleSummary["author"],
let image = articleSummary["image"],
let date = articleSummary["date"] {
var html = "<html>"
html += "<head>"
html += "<meta name="viewport" content="width=device-width, initial-scale=1">"
html += "<style> body { font-size: 150%; } </style>"
html += "</head>"
html += "<body>"
html += "<h1>(title)</h1>"
html += "<h4>(author)</h4>"
html += "<p>(date)</p>"
html += "<img src="(image)" alt="" />"
html += description
html += "</body>"
html += "</html>"
webView.loadHTMLString(html, baseURL: nil)
}
I tried that and I get errors for each item saying “Use of unresolved identifier ...”
– fmz
Jul 3 at 0:19
you need to add those variables in the if let statement. check my updated answer
– Christian Abella
Jul 3 at 0:36
Excellent! Thank you very much.
– fmz
Jul 3 at 0:39
no worries. happy to help.
– Christian Abella
Jul 3 at 0:44
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
how did you add your webView on your ViewController?
– Christian Abella
Jul 2 at 23:54