How to access child component values from the parent in react native?
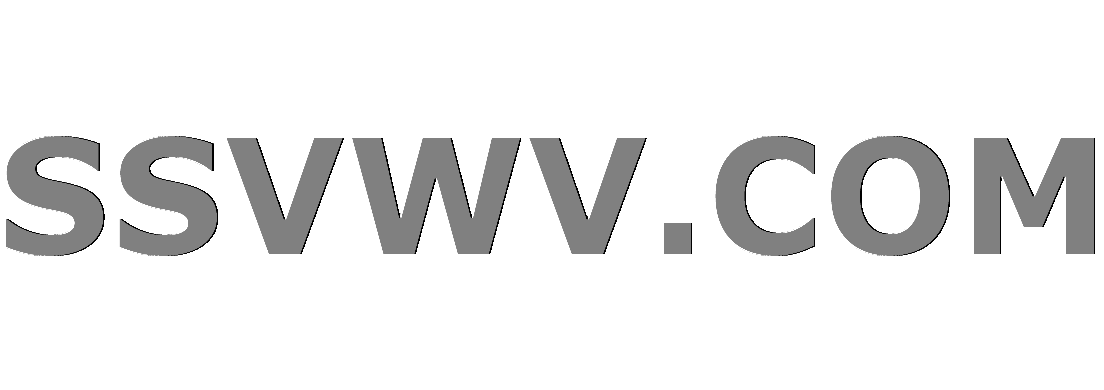
Multi tool use
How to access child component values from the parent in react native?
I have a login screen with following structure:
import Logo from '../components/Logo'
import Form from '../components/Form';
export default class Login extends React. Component {
<View style={styles.container} >
<Logo/>
<Form type="login"/>
<View style={styles.signUpTextCont}>
...
</View>
</View>
and here is my Form component:
export default class Form extends React.Component {
constructor (props){
super(props)
this.state = {
username : '',
password : ''
}
}
handleChangeUsername = (text) => {
this.setState({ username: text })
}
handleChangePassword = (text) => {
this.setState({ password: text })
}
render() {
return (
<View style={styles.container} >
<TextInput
ref={(input) => { this.username = input }}
onChangeText = {this.handleChangeUsername}
value = {this.state.username}
/>
<TextInput
ref={(input) => { this.password = input }}
onChangeText = {this.handleChangePassword}
value = {this.state.password}
/>
<TouchableOpacity style={styles.button}>
<Text style={styles.buttonText}>{this.props.type}</Text>
</TouchableOpacity>
</View>
);
}
}
now I would like to have a checkLogin() method in Login screen (parent).
How can I access username and password values to check them in the Login screen?
I will be grateful if someone could help.
2 Answers
2
Try using ref
keyword for accessing the child values.
ref
<View style={styles.container} >
<Logo/>
<Form type="login"
ref={'login'}/>
<View style={styles.signUpTextCont}>
...
</View>
</View>
To Acess Child Component Values in parent:
onClick = () =>{
//you can access properties on child component by following style:
let userName = this.refs['login'].state.username;
let password = this.refs['login'].state.password;
}
you can use callback to send username and password to parent like this sample code:
Form:
handleChangeUsername = (text) => {
this.setState({ username: text })
this.props.userChange(text)
}
handleChangePassword = (text) => {
this.setState({ password: text })
this.props.passChange(text)
}
login:
add two state named user and pass and:
setUser = (text) => {
this.setState({user:text})
}
setPass = (text) => {
this.setState({pass:text})
}
checkLogin = () => {
// check user and pass state...
}
<Form
type="login"
userChange = {(text) => { this.setUser(text) } }
passChange = {(text) => { this.setPass(text) } }
/>
and now, user and pass is in state in login and you can check it.
I hope this can help you
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
on your parent component set a prop like loginDetails={this.login} and in child component onChange do this.props.loginDetails(username,password); and you will get the data in login()...this is a way..
– Aravind S
Jul 3 at 7:36