How to reload or re-render the entire page using AngularJS
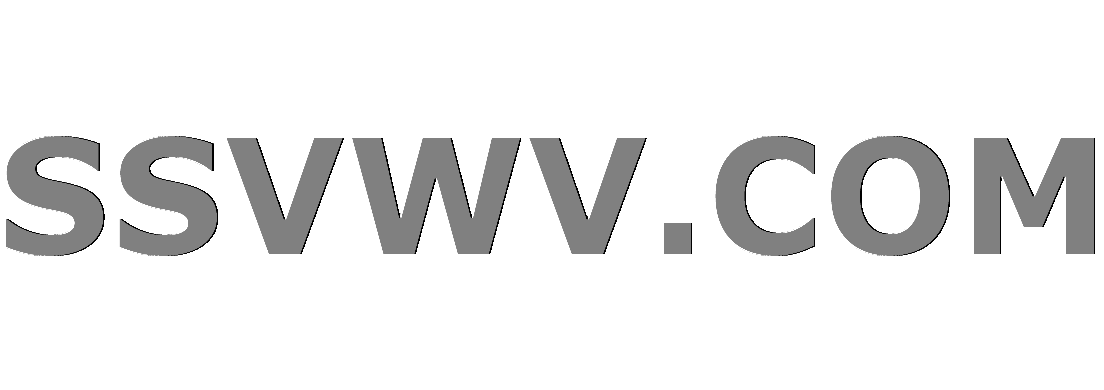
Multi tool use
How to reload or re-render the entire page using AngularJS
After rendering the entire page based on several user contexts and having made several $http
requests, I want the user to be able to switch contexts and re-render everything again (resending all $http
requests, etc). If I just redirect the user somewhere else, things work properly:
$http
$http
$scope.on_impersonate_success = function(response) {
//$window.location.reload(); // This cancels any current request
$location.path('/'); // This works as expected, if path != current_path
};
$scope.impersonate = function(username) {
return auth.impersonate(username)
.then($scope.on_impersonate_success, $scope.on_auth_failed);
};
If I use $window.location.reload()
, then some of the $http
requests on auth.impersonate(username)
that are waiting for a response get cancelled, so I can't use that. Also, the hack $location.path($location.path())
doesn't work either (nothing happens).
$window.location.reload()
$http
auth.impersonate(username)
$location.path($location.path())
Is there another way to re-render the page without manually issuing all requests again?
Possible duplicate of AngularJs: Reload page
– Alena Kastsiukavets
Aug 3 '17 at 12:57
11 Answers
11
For the record, to force angular to re-render the current page, you can use:
$route.reload();
According to AngularJS documentation:
Causes $route service to reload the current route even if $location hasn't changed.
As a result of that, ngView creates new scope, reinstantiates the controller.
Thanks for the comment, for some reason I seem to get slightly different behavior when I use
$route.reload()
compared to a normal refresh. For example a tab is set to show initially on refresh, but when the reload method seems to reload the page without setting the tab, not sure what the problem is.– Doug Molineux
May 28 '14 at 15:36
$route.reload()
Does this run filters/services as well? Getting undesired persistent state. Edit: Still upvoted for answering the question correctly & this is super helpful. Thank you
– grant
Nov 4 '14 at 20:15
Remember to inject
$route
to the controller for this to work.– Neel
Jun 3 '15 at 18:37
$route
@alphapilgrim The ngRoute module is no longer part of the core
angular.js file
. If you are continuing to use $routeProvider
then you will now need to include angular-route.js
in your HTML:– Alvaro Joao
Feb 4 '16 at 16:02
angular.js file
$routeProvider
angular-route.js
$state.reload() if you using stateProvider
– Lalit Rao
Apr 3 '17 at 11:06
$route.reload()
will reinitialise the controllers but not the services. If you want to reset the whole state of your application you can use:
$route.reload()
$window.location.reload();
This is a standard DOM method which you can access injecting the $window service.
If you want to be sure to reload the page from the server, for example when you are using Django or another web framework and you want a fresh server side render, pass true
as a parameter to reload
, as explained in the docs. Since that requires interaction with the server, it will be slower so do it only if necessary
true
reload
The above applies to Angular 1. I am not using Angular 2, looks like the services are different there, there is Router
, Location
, and the DOCUMENT
. I did not test different behaviors there
Router
Location
DOCUMENT
It is worth noting that one shouldn't be storing state in the services anyway to require services to be "restarted".
– andersonvom
Oct 22 '14 at 13:44
Who says that? Where is one supposed to store cached data?
– danza
Oct 23 '14 at 9:52
I have stumbled upon lots and lots of bugs due to data being stored in services, making them stateful rather than stateless. Unless we're talking about a caching service (in which case, there would be an interface to flush it), I would keep data out of services and in the controllers. For instance, one could keep data in localStorage, and use a service to access it. This would free the service from keeping data directly in it.
– andersonvom
Oct 23 '14 at 14:07
@danza Where is one supposed to store cached data? ... a model object? Howver Angular has done a terrible job of naming their MVCS constituents. I keep injectable model objects via the module.value API. Then the various APIs I defined on an ng service that alter the model object work more like commands.
– jusopi
Nov 14 '14 at 14:39
Sure, that will work, but it is not good practice. See the documentation about $window, the reason for using it is explained in the beginning: While window is globally available in JavaScript, it causes testability problems, because it is a global variable. In angular we always refer to it through the $window service, so it may be overridden, removed or mocked for testing
– danza
Sep 1 '15 at 8:23
For reloading the page for a given route path :-
$location.path('/path1/path2');
$route.reload();
Don't know why but the .reload() method seems not working here. It doesn't realod anything.
– mircobabini
Jul 12 '14 at 11:09
If you are using angular ui-router this will be the best solution.
$scope.myLoadingFunction = function() {
$state.reload();
};
Well maybe you forgot to add "$route" when declaring the dependencies of your Controller:
app.controller('NameCtrl', ['$scope','$route', function($scope,$route) {
// $route.reload(); Then this should work fine.
}]);
Just to reload everything , use window.location.reload(); with angularjs
Check out working example
HTML
Reset
angularJS
var myApp = angular.module('myApp',);
function MyCtrl($scope) {
$scope.reloadPage = function(){window.location.reload();}
}
http://jsfiddle.net/HB7LU/22324/
Easiest solution I figured was,
add '/' to the route that want to be reloaded every time when coming back.
eg:
instead of the following
$routeProvider
.when('/About', {
templateUrl: 'about.html',
controller: 'AboutCtrl'
})
use,
$routeProvider
.when('/About/', { //notice the '/' at the end
templateUrl: 'about.html',
controller: 'AboutCtrl'
})
what does this mean ? not working for angular2 ?
– Pardeep Jain
Feb 24 '16 at 10:15
@PardeepJain This worked for me. That is why this is an answer. Did you downvote my answer?
– Diyoda_
Feb 27 '16 at 0:17
no not at all, your answer is not so bad to downvote. amazing i don't now why same is not working for me in angular2.
– Pardeep Jain
Feb 27 '16 at 10:24
Perfect solution. Thks!
– simon
Apr 27 '16 at 12:52
This works even if your original path is the root of the application -
/
:O My route now looks like this: //
– MadPhysicist
Oct 10 '16 at 20:12
/
//
If you want to refresh the controller while refreshing any services you are using, you can use this solution:
i.e.
app.controller('myCtrl',['$scope','MyService','$state', function($scope,MyService,$state) {
//At the point where you want to refresh the controller including MyServices
$state.reload();
//OR:
$state.go($state.current, {}, {reload: true});
}
This will refresh the controller and the HTML as well you can call it Refresh or Re-Render.
this is for ui router not for angular.
– dgzornoza
Jul 4 '16 at 10:22
I got this working code for removing cache and reloading the page
View
<a class="btn" ng-click="reload()">
<i class="icon-reload"></i>
</a>
Controller
Injectors: $scope,$state,$stateParams,$templateCache
$scope.reload = function() { // To Reload anypage
$templateCache.removeAll();
$state.transitionTo($state.current, $stateParams, { reload: true, inherit: true, notify: true });
};
Use the following code without intimate reload notification to the user. It will render the page
var currentPageTemplate = $route.current.templateUrl;
$templateCache.remove(currentPageTemplate);
$window.location.reload();
I've had a hard fight with this problem for months, and the only solution that worked for me is this:
var landingUrl = "http://www.ytopic.es"; //URL complete
$window.location.href = landingUrl;
This is hardly different than doing
$window.location.reload()
which the OP specifically mentions does not work properly in his case.– cpburnz
Mar 10 '15 at 17:53
$window.location.reload()
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
As Alvaro Joao says below, you need to use angular-route.js in order to get this to work. bennadel.com/blog/…
– Yvonne Aburrow
Sep 15 '16 at 14:14