Lua SWIG Basics
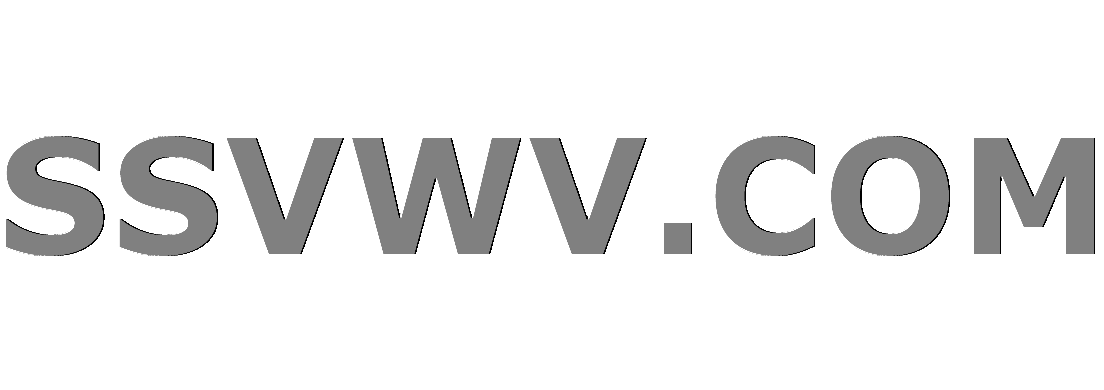
Multi tool use
Lua SWIG Basics
Im trying to implement basic vector class manipulation using the instructions provided at:
http://swig.org/Doc1.3/SWIG.html#SWIG_adding_member_functions
And I have the following .i:
%module mymodule
%{
typedef struct
{
float x,y,z;
} Vector3f;
%}
typedef struct
{
float x,y,z;
} Vector3f;
%extend Vector3f {
Vector3f(float x, float y, float z) {
Vector3f *v;
v = (Vector3f *) malloc(sizeof(Vector3f));
v->x = x;
v->y = y;
v->z = z;
return v;
}
~Vector3f() {
free($self);
}
void print() {
printf("Vector [%f, %f, %f]n", $self->x,$self->y,$self->z);
}
};
Now my problem is if I call the following code in Lua:
print(mymodule)
local v = Vector(3,4,0)
v.print()
--By the way is there an equivalent in Lua?
--del v
I got the follow output:
table: 0x000001F9356B1920
attempt to call global 'Vector' (a nil value)
Obviously the module is loaded properly as I first print the table address
but I cannot create a Vector... I also tried calling the module method like mymodule:Vector(1,2,3)
still generate an error. What am I missing here?
mymodule:Vector(1,2,3)
All I want is to generate a new Vector
and have the GC destroying it
using the ~Vector3f() method. What should I modify to make this mechanism
working?
Vector
1 Answer
1
SWIG will automatically generate a __gc
metamethod from the destructor. In principle your class doesn't even need a custom destructor, the default one will do just fine.
__gc
Furthermore SWIG does not need to know about all the implementation details of the functions, the signatures are totally sufficient for generating wrapper code. That is why I have moved the Vector3f
struct into the literal C++ section (which could also be in a header file) and only repeated the signatures.
Vector3f
Rather than having a print
member function, why not add capabilities to use Vector3f
with Lua's print()
function? Just write a __tostring
function which returns a string representation of your object.
print
Vector3f
print()
__tostring
test.i
test.i
%module mymodule
%{
#include <iostream>
struct Vector3f {
float x,y,z;
Vector3f(float x, float y, float z) : x(x), y(y), z(z) {
std::cout << "Constructing vectorn";
}
~Vector3f() {
std::cout << "Destroying vectorn";
}
};
%}
%include <std_string.i>
struct Vector3f
{
Vector3f(float x, float y, float z);
~Vector3f();
};
%extend Vector3f {
std::string __tostring() {
return std::string{"Vector ["}
+ std::to_string($self->x) + ", "
+ std::to_string($self->y) + ", "
+ std::to_string($self->z) + "]";
}
};
test.lua
test.lua
local mymodule = require("mymodule")
local v = mymodule.Vector3f(3,4,0)
print(v)
Example workflow to compile and run:
$ swig -lua -c++ test.i
$ clang++ -Wall -Wextra -Wpedantic -I/usr/include/lua5.2/ -fPIC -shared test_wrap.cxx -o mymodule.so -llua5.2
$ lua test.lua
Constructing vector
Vector [3.000000, 4.000000, 0.000000]
Destroying vector
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.