Node.js: get path from the request
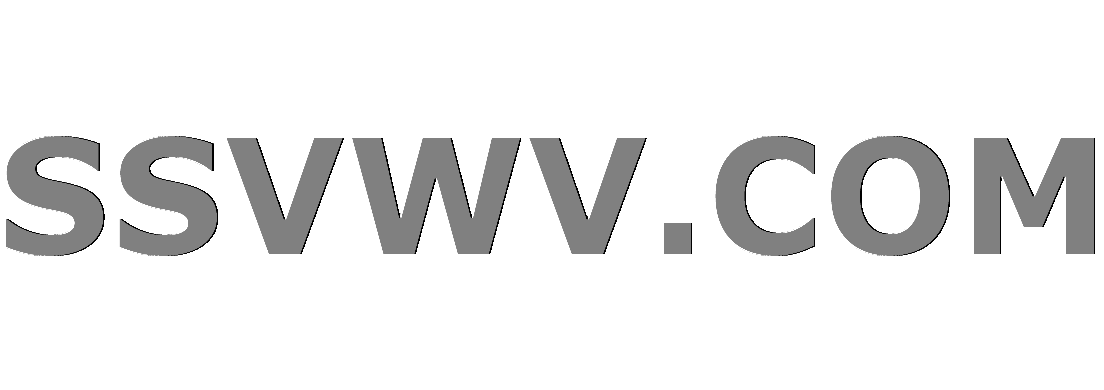
Multi tool use
Node.js: get path from the request
I have a service called "localhost:3000/returnStat" that should take a file path as parameter. For example '/BackupFolder/toto/tata/titi/myfile.txt'.
How can I test this service on my browser?
How can I format this request using Express for instance?
exports.returnStat = function(req, res) {
var fs = require('fs');
var neededstats = ;
var p = __dirname + '/' + req.params.filepath;
fs.stat(p, function(err, stats) {
if (err) {
throw err;
}
neededstats.push(stats.mtime);
neededstats.push(stats.size);
res.send(neededstats);
});
};
and with the browser, just for quick tests
– Nabil Djarallah
Sep 21 '13 at 11:04
6 Answers
6
var http = require('http');
var url = require('url');
var fs = require('fs');
var neededstats = ;
http.createServer(function(req, res) {
if (req.url == '/index.html' || req.url == '/') {
fs.readFile('./index.html', function(err, data) {
res.end(data);
});
} else {
var p = __dirname + '/' + req.params.filepath;
fs.stat(p, function(err, stats) {
if (err) {
throw err;
}
neededstats.push(stats.mtime);
neededstats.push(stats.size);
res.send(neededstats);
});
}
}).listen(8080, '0.0.0.0');
console.log('Server running.');
I have not tested your code but other things works
If you want to get the path info from request url
var url_parts = url.parse(req.url);
console.log(url_parts);
console.log(url_parts.pathname);
1.If you are getting the URL parameters still not able to read the file just correct your file path in my example. If you place index.html in same directory as server code it would work...
2.if you have big folder structure that you want to host using node then I would advise you to use some framework like expressjs
If you want raw solution to file path
var http = require("http");
var url = require("url");
function start() {
function onRequest(request, response) {
var pathname = url.parse(request.url).pathname;
console.log("Request for " + pathname + " received.");
response.writeHead(200, {"Content-Type": "text/plain"});
response.write("Hello World");
response.end();
}
http.createServer(onRequest).listen(8888);
console.log("Server has started.");
}
exports.start = start;
source : http://www.nodebeginner.org/
My code works, but how can I retrive the file path (req parameter such as '/BackupFolder/toto/tata/titi/myfile.txt')
– Nabil Djarallah
Sep 21 '13 at 11:02
simply call req.url
. that should do the work. you'll get something like /something?bla=foo
req.url
/something?bla=foo
The path in your example is
/something
.– Patrick Fisher
May 14 '14 at 4:37
/something
use
req.url.match('^[^?]*')[0]
to only show the path– epegzz
May 30 '15 at 15:07
req.url.match('^[^?]*')[0]
Based on @epegzz suggestion for the regex.
( url ) => {
return url.match('^[^?]*')[0].split('/').slice(1)
}
returns an array with paths.
req.protocol + '://' + req.get('host') + req.originalUrl
or
req.protocol + '://' + req.headers.host + req.originalUrl
// I like this one as it survives from proxy server, getting the original host name
req.protocol + '://' + req.headers.host + req.originalUrl
You can use this in app.js
file .
app.js
var apiurl = express.Router();
apiurl.use(function(req, res, next) {
var fullUrl = req.protocol + '://' + req.get('host') + req.originalUrl;
next();
});
app.use('/', apiurl);
Combining solutions above when using express request:
let url=url.parse(req.originalUrl);
let page = url.parse(uri).path?url.parse(uri).path.match('^[^?]*')[0].split('/').slice(1)[0] : '';
this will handle all cases like
localhost/page
localhost:3000/page/
/page?item_id=1
localhost:3000/
localhost/
etc. Some examples:
> urls
[ 'http://localhost/page',
'http://localhost:3000/page/',
'http://localhost/page?item_id=1',
'http://localhost/',
'http://localhost:3000/',
'http://localhost/',
'http://localhost:3000/page#item_id=2',
'http://localhost:3000/page?item_id=2#3',
'http://localhost',
'http://localhost:3000' ]
> urls.map(uri => url.parse(uri).path?url.parse(uri).path.match('^[^?]*')[0].split('/').slice(1)[0] : '' )
[ 'page', 'page', 'page', '', '', '', 'page', 'page', '', '' ]
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Yep create a REST call see this article erichonorez.wordpress.com/2013/02/10/…
– lastboy
Sep 21 '13 at 10:28