protractor ExpectedConditions wait for DOM attribute to contain
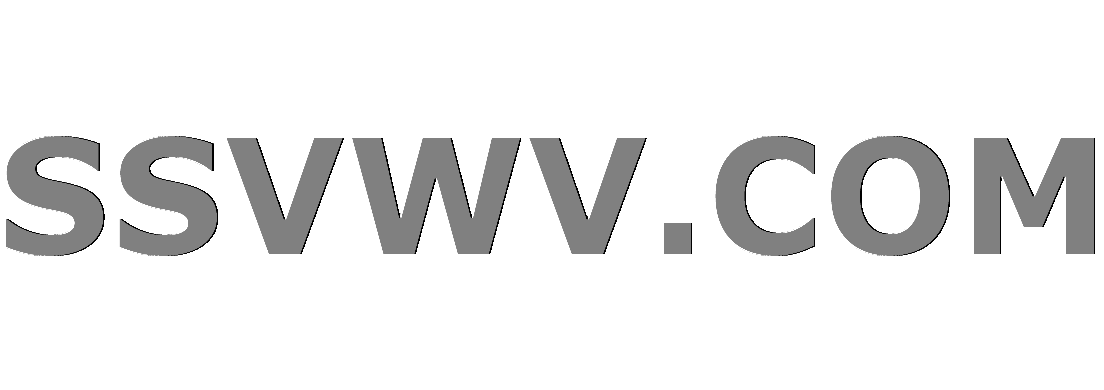
Multi tool use
protractor ExpectedConditions wait for DOM attribute to contain
I have the following DOM element:
input type="checkbox" class="ng-untouched ng-pristine ng-valid" ng-reflect-model="false"
In Protractor- I need to wait for the attribute ng-reflect-model
to equal true before I perform my assertions. Is there a browser.wait ExpectedCondition
to do this? If so, what is it?
ng-reflect-model
browser.wait ExpectedCondition
I'm looking for something like Selenium's attributeContains
attributeContains
3 Answers
3
There is no predefined expected condition available in protractor to verify attribute change for an element. But you can create your own wait condition like below,
browser.wait(function(){
return element(by.css("input[type='checkbox']"))
.getAttribute("ng-reflect-model")
.then(function(ngReflectModel){
return ngReflectModel == "false";
})
},5000);
How does this look if I want to pass in parameters rather than have static values?
timer = 5000;
static waitForAttributeToContain(item: ElementFinder, attribute: string, value: string) {
browser.wait(() => {
return item.getAttribute(attribute).then(
function(attributeCheck) {
return attributeCheck === value;
});
}, timer);
}
You can create a helper function which can be used in other places too, for waiting for a specific condition:
export async function forCondition(condition: () => boolean | PromiseLike<boolean>, timeout = 20000): Promise<boolean> {
try {
return await browser.wait(condition, timeout);
} catch (err) {
return false;
}
}
In code you can then do something like:
// other helper function
async function isValueSet(): Promise<boolean> {
let input = element(by.model('input');
let value = await input.getAttribute('ng-reflect-model');
// cast to boolean operator.
return !!value;
}
And then in the test:
it('should wait for value to be true', async function() {
await forCondition(function () { return isValueSet(); }, 30000);
// expect()...
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.