Return type in asynchronous function using mongoose produces error
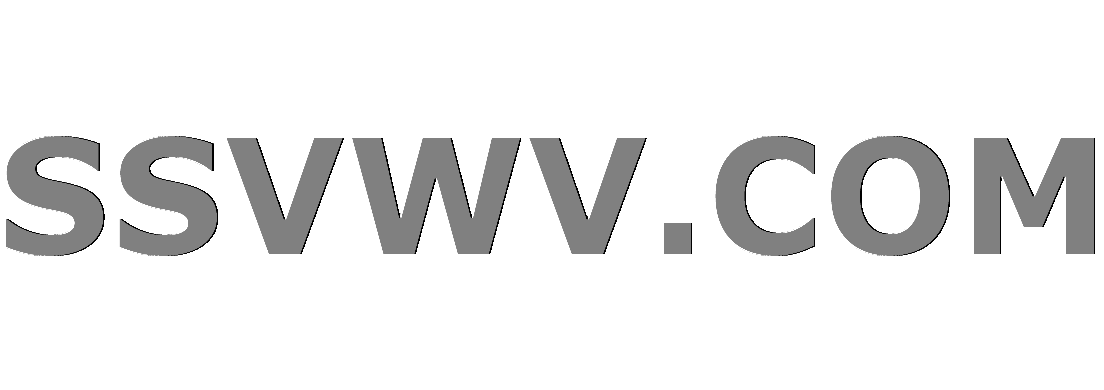
Multi tool use
Return type in asynchronous function using mongoose produces error
I am getting an error while trying to compile the following code:
public getCompanies(): Array<ICompany>{
Company.find({}, (err, data)=>{
if (err) console.log(err);
let companyArray = new Array();
for (let company of data){
companyArray.push(company);
}
return companyArray;
});
}
There is an error with the return type of the function: error TS2355: A function whose declared type is neither 'void' nor 'any' must return a value.
Got the same error using then/catch functions.
Is there a way to make this work without converting the return type to any?
Thank you in advance
1 Answer
1
The compiler is right about your function not actually returning any value.
The problem is that Company.find
takes a callback which is executed at a later date when the requested query competes. So everything you write after (err, data)=>{
will get not get executed when you call getCompanies
, and the return
you have is not a return from getCompanies
, but rather a return from an anonymous function that get executed later.
Company.find
(err, data)=>{
getCompanies
return
getCompanies
You can either use a similar approach (passing in a callback) to be notified of when the query completes:
class foo {
public getCompanies(onCompleted: (data: ICompany)=> void): void{
Company.find({}, (err, data)=>{
if (err) console.log(err);
let companyArray = new Array();
for (let company of data){
companyArray.push(company);
}
onCompleted(companyArray);
});
}
public usage(){
this.getCompanies(data=>
{
console.log(data);
})
}
}
Or you can just return the DocumentQuery
that find
returns and use then
or async/await
to get the result:
DocumentQuery
find
then
async/await
class foo {
public getCompanies() {
return Company.find({}, (err, data)=>{
if (err) {
console.log(err);
}
let companyArray = new Array();
for (let company of data){
companyArray.push(company);
}
});
}
public async usageAsync(){
let data = await this.getCompanies();
console.log(data);
}
public usage(){
this.getCompanies()
.then (data=>
{
console.log(data);
})
}
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.