Save QPainter after called update
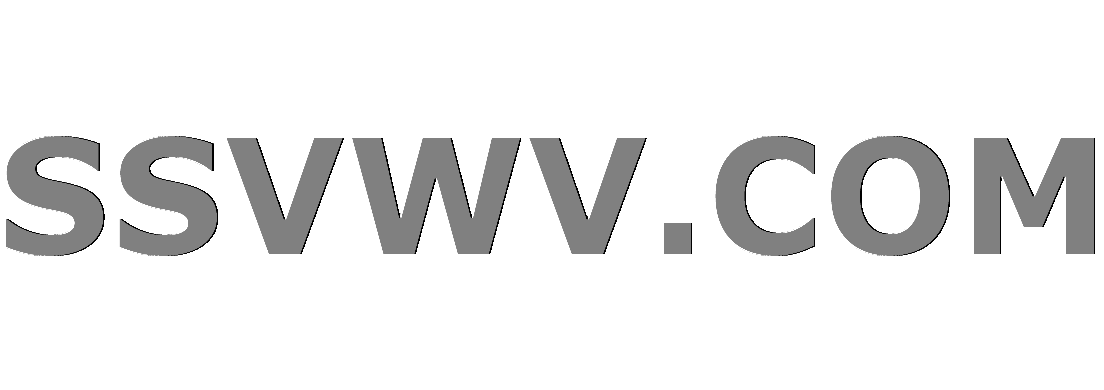
Multi tool use
Save QPainter after called update
I want to draw straight lines on qml , but every time I draw a line, the previous disappears, I wonder if it has any thing to do with the update method, any ways to solve this problem.
main.qml
MouseArea{
id:fidpoint
anchors.fill: parent
onPressed: {
switch(addstate.currentText){
case 'Track':
map.setTstart(mouseX,mouseY);
draw_line.setColor("black");
draw_line.setStart(Qt.point(mouseX,mouseY));
draw_line.setEnd(Qt.point(mouseX,mouseY));
draw_line.update();
break;
}
}
onReleased: {
switch(addstate.currentText){
case 'Track':
map.addTrack(mouseX,mouseY);
draw_line.setColor("black");
draw_line.setEnd(Qt.point(mouseX,mouseY));
draw_line.update();
break;
}
}
onPositionChanged: {
switch(addstate.currentText){
case 'Track':
draw_line.setColor("black");
draw_line.setEnd(Qt.point(mouseX,mouseY));
draw_line.update();
break;
}
}
}
draw_line is the Object's id that I registered to qml from main.cpp
paint.cpp
void Paint::paint(QPainter *painter)
{
QPen pen(m_color, 2);
painter->setPen(pen);
painter->setRenderHint(QPainter::Antialiasing,true);
painter->drawLine(startNode,endNode);
}
paint is the class that inherits from QQuickPaintedItem
QQuickPaintedItem
1 Answer
1
A possible option would be to create an image and save the changes there, but this would solve your problem in the short term. A better option is that draw_line draw only one line, then add it as a child of the item you want the canvas to be.
lineitem.h
#ifndef LINEITEM_H
#define LINEITEM_H
#include <QQuickPaintedItem>
class LineItem: public QQuickPaintedItem
{
Q_OBJECT
Q_PROPERTY(QPoint startPos READ startPos WRITE setStartPos NOTIFY startPosChanged)
Q_PROPERTY(QPoint endPos READ endPos WRITE setEndPos NOTIFY endPosChanged)
Q_PROPERTY(QColor lineColor READ lineColor WRITE setLineColor NOTIFY lineColorChanged)
public:
using QQuickPaintedItem::QQuickPaintedItem;
void paint(QPainter *painter);
QPoint startPos() const;
void setStartPos(const QPoint &startPos);
QPoint endPos() const;
void setEndPos(const QPoint &endPos);
QColor lineColor() const;
void setLineColor(const QColor &lineColor);
signals:
void startPosChanged();
void endPosChanged();
void lineColorChanged();
private:
QPoint m_startPos;
QPoint m_endPos;
QColor m_lineColor;
};
#endif // LINEITEM_H
lineitem.cpp
#include "lineitem.h"
#include <QPainter>
void LineItem::paint(QPainter *painter)
{
painter->setRenderHint(QPainter::Antialiasing, true);
QPen pen(m_lineColor, 2);
painter->setPen(pen);
painter->drawLine(m_startPos, m_endPos);
}
QPoint LineItem::startPos() const
{
return m_startPos;
}
void LineItem::setStartPos(const QPoint &startPos)
{
if(m_startPos == startPos)
return;
m_startPos = startPos;
emit startPosChanged();
update();
}
QPoint LineItem::endPos() const
{
return m_endPos;
}
void LineItem::setEndPos(const QPoint &endPos)
{
if(m_endPos == endPos)
return;
m_endPos = endPos;
emit endPosChanged();
update();
}
QColor LineItem::lineColor() const
{
return m_lineColor;
}
void LineItem::setLineColor(const QColor &lineColor)
{
if(m_lineColor == lineColor)
return;
m_lineColor = lineColor;
emit lineColorChanged();
update();
}
main.qml
import QtQuick 2.9
import QtQuick.Window 2.2
import com.eyllanesc.org 1.0
Window {
id: win
visible: true
width: 640
height: 480
title: qsTr("Hello World")
property LineItem currentItem: null
Rectangle{
id: canvas
anchors.fill: parent
MouseArea{
anchors.fill: parent
onPressed: {
currentItem = create_lineitem(canvas)
currentItem.lineColor = "green"
currentItem.anchors.fill = canvas
currentItem.startPos = Qt.point(mouseX,mouseY)
currentItem.endPos = Qt.point(mouseX,mouseY)
}
onReleased: currentItem.endPos = Qt.point(mouseX,mouseY)
onPositionChanged: currentItem.endPos = Qt.point(mouseX,mouseY)
}
}
function create_lineitem(parentItem, color) {
return Qt.createQmlObject('import com.eyllanesc.org 1.0; LineItem{}',
parentItem);
}
}
main.cpp
#include "lineitem.h"
#include <QGuiApplication>
#include <QQmlApplicationEngine>
int main(int argc, char *argv)
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
qmlRegisterType<LineItem>("com.eyllanesc.org", 1, 0, "LineItem");
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (engine.rootObjects().isEmpty())
return -1;
return app.exec();
}
The complete example can be found in the following link.
@Changlin I have updated the example, try it back and tell me if you have problems, I recommend downloading the files.
– eyllanesc
Jul 3 at 3:45
It worked, thanks! Just another question to ask, if I got several sets of start and end points, how can I call the LineItem class to draw the points out?
– Changlin
Jul 3 at 5:06
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
@Changlin What version of Qt do you use? An Item has a painting space, this is not unlimited, with anchors.fill = stable parent that the item occupies all the space of the father. Have you downloaded my code, have you modified it?
– eyllanesc
Jul 3 at 3:41