Spring Boot - Request Mapping won't accept trailing slash or multiple directories
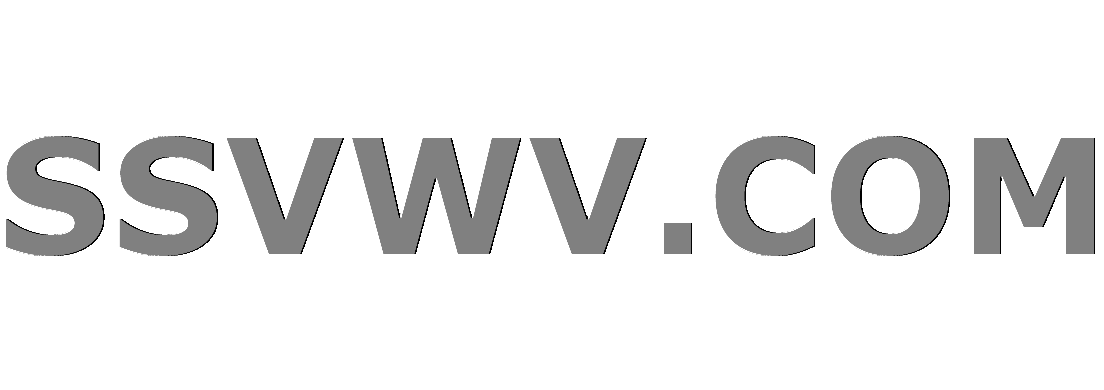
Multi tool use
Spring Boot - Request Mapping won't accept trailing slash or multiple directories
When RequestMapping in my controller, I'm able to map an html file to "/" and another to "/users". However, trying to map to "/users/" or "/users/test" won't work. In the console it'll say that the endpoint was mapped, but on trying to access it I'll get the 404 error page.
package com.bridge.Bitter.controllers;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class BitterController {
//works
@RequestMapping(value="/")
public String getMainPage(){
return "main.html";
}
//works
@RequestMapping(value="/users")
public String getUsersPage(){
return "users.html";
}
//doesn't work, Whitelabel error page
@RequestMapping(value="/users/")
public String getUsersSlashPage(){
return "users.html";
}
//doesn't work, Whitelabel error page
@RequestMapping(value="/users/test")
public String getUsersTestPage(){
return "users.html";
}
}
My application.properties only contains "spring.data.rest.basePath=/api".
If I change from @Controller to @Rest Controller, The following occurs:
package com.bridge.Bitter.controllers;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.RequestMapping;
@RestController
public class BitterController {
//works
@RequestMapping(value="/")
public String getMainPage(){
return "main.html";
}
//returns a webpage with the text "users.html" on it instead of serving the html
@RequestMapping(value="/users")
public String getUsersPage(){
return "users.html";
}
//returns a webpage with the text "users.html" on it instead of serving the html
@RequestMapping(value="/users/")
public String getUsersSlashPage(){
return "users.html";
}
//returns a webpage with the text "users.html" on it instead of serving the html
@RequestMapping(value="/users/test")
public String getUsersTestPage(){
return "users.html";
}
}
Changing the functions from returning strings to returning
new ModelAndView("user.html")
works for /users but will then 404 error for /users/ and /users/test.
1 Answer
1
You're trying to map the same path twice. /users is treated the same as /users/, so Spring can not resolve which controller method should handle the request.
You can just try this:
package com.bridge.Bitter.controllers;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class BitterController {
@RequestMapping("/")
public String getMainPage(){
return "main.html";
}
@RequestMapping("/users")
public String getUsersPage(){
return "users.html";
}
@RequestMapping("/users/test")
public String getUsersTestPage(){
return "users.html";
}
}
In the other hand, when you use @RestController annotation, you always return a response text in JSON format, that's why you always get the same result.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Even if I'm only mapping one, as in my controller only contains the mapping for /users/, it won't map to localhost:8080/users/. Same for if I JUST have /users/test. However, /users works. Method names shouldn't have been the same, that was a mistake on my part in the question.
– Justin Auger
Jul 3 at 21:42