What is the best way to integrate Identity with my app database?
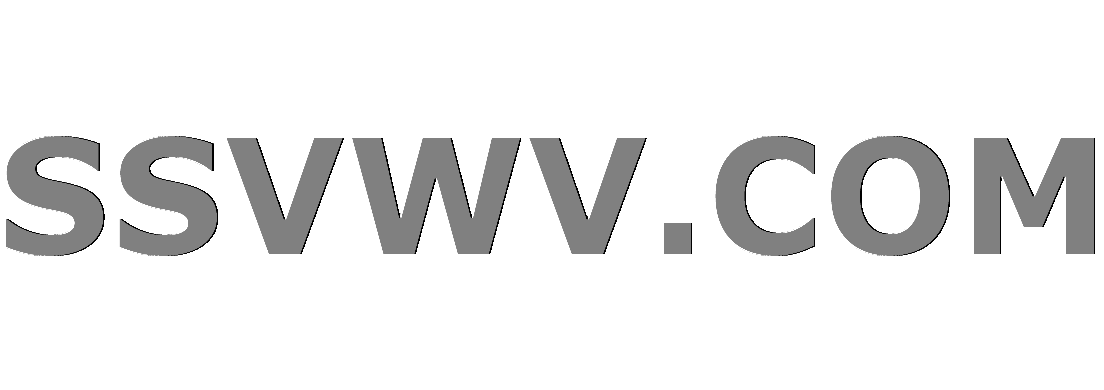
Multi tool use
What is the best way to integrate Identity with my app database?
I'm learning Asp.net Core and Entity Framework Core and I created a basic web app with a database with code first approach. My app has a context (AppContext) and to use Identity I have defined a new Context (IdentityContext), which uses the same database.
I want to associate every Identity's User (AppUser from IdentityContext) with one Employee (from AppContext), but when I try it EF Core create a new table for it.
public class AppUser : IdentityUser
{
public Employee Employee { get; set; }
}
public class Employee
{
public long Id { get; set; }
public string AspNetUserId { get; set; }
public AppUser AppUser { get; set; }
}
AppContext
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Employee>(employee =>
{
employee.HasIndex(e => e.AspNetUserId);
employee
.HasOne(e => e.AppUser)
.WithOne(au => au.Employee)
.HasForeignKey<Employee>(e=>e.AspNetUserId);
});
}
I have a lot of questions about it:
Any suggestion or reference will be appreciated.
Thank you.
1 Answer
1
I think it's ok to have two separated context, each context has a just
one concern. Is it ok? or should I copy-paste my AppContext on the
IdentityContext?
It's perfectly fine. The problem is that you want to separate them, but then NOT let them have their own concerns.
You're currently telling your IdentityContext that it should have both the AppUser and the Employee, and that there is a relationship between them. Then the context will take care of both of them unless you explicitly tell it not to. But right now you are explicitly telling it to.
It can pretty much be summed up to this simple rule: Separated contexts -> Separated data.
How can I tell to my AppContext that doesn't create a new table for
the AppUser, and use the table created by the IdentityContext?
You really shouldn't. Don't expect Entity Framework to handle relationships between contexts. It pretty much won't. If your entities have a connection, they belong in the same context. You can see it as being different databases. Querying between two different databases isn't that smooth.
You could however still separate them, by telling the configuration to ignore the referenced entities like this:
modelBuilder.Entity<AppUser>().Ignore(e => e.Employee);
However, it will probably only be confusing since those entities won't get tracked or mapped unless you do it yourself. Thus, I recommend either sticking with an Id as the reference/foreign key and let them live their own lives, or combining them and executing your current plan. Sticking to one context is what I'd recommend if you're new in the game.
There's any alternative?
I like the idea of separating one context for the identity, as some logic for that could be related to the UI Layer, and that it's actually quite rare to need any references to other entities on the user. It does come with a little extra effort with migrations and such, but all in all it works quite well. You can still easily fetch related entities that belongs to the user by some neat generic methods and interfaces.
But not even I would do it if I had less than 15-20 entities. It's just not worth it. The benefits or too few in such a small project.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.