componentDidMount() not working but hitting debugger
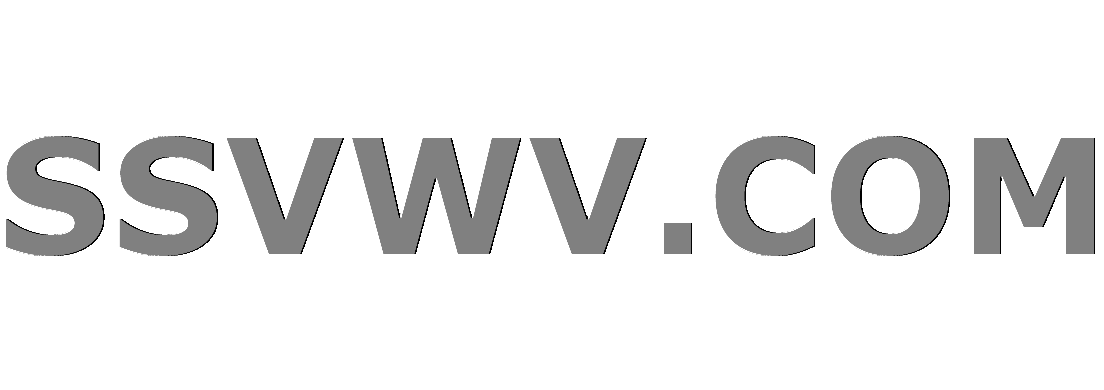
Multi tool use
componentDidMount() not working but hitting debugger
this is my first time posting anything here so I am very open to comments on the way I posted as well as the post itself. I am just 8 weeks into learning programming, so there is probably a lot wrong.
In this case, I specifically want help with the following code.
// import React, { Component } from 'react'
import React from 'react'
import { connect } from 'react-redux'
// import { Link } from 'react-router-dom'
import { getUnits } from '../reducers/units'
import { Menu, Container, Grid, Header,m} from 'semantic-ui-react'
class AllUnits extends React.Component {
componentDidMount() {
console.log ("component did mount!")
debugger
this.props.dispatch(getUnits())
debugger
}
units = () => {
debugger
return this.props.units.map( unit => {
<Grid textAlign='center' columns={1}>
<Grid.Row>
<Grid.Column>
<Menu fluid vertical>
<Menu.Item className='header'>{this.position} {unit.name}</Menu.Item>
</Menu>
</Grid.Column>
</Grid.Row>
</Grid>
})
}
render() {
debugger
return (
<Container>
<Header as="h3" textAlign="center">Units</Header>
{ this.units() }
</Container>
)
}
}
const mapStateToProps = (state) => {
return { units: state.units }
debugger
}
export default connect(mapStateToProps)(AllUnits);
Somehow it is hitting that debugger, but not logging anything. I know it is hitting my route and my component because it finds an error in some code later on, but it never never console.logs my message.
Thanks in advance.
Edit: By request, I have put in the whole component.
Edit2: I don't know if this is related, but when it gets through all of the debuggers in this component and one or two in the reducer, this is the error:
TypeError: Cannot read property 'id' of undefined
AllUnits.componentDidMount
src/components/AllUnits.js:13
10 | componentDidMount() {
11 | console.log ("component did mount!")
12 | debugger
> 13 | this.props.dispatch(getUnits())
14 | debugger
15 | }
16 |
And here is the reducer:
import axios from 'axios';
import { setFlash } from './flash';
import { setHeaders } from './headers';
import { dispatch } from 'react';
const GET_UNITS = 'GET_UNITS';
export const getUnits = (courseId) => {
return() => {
axios.get(`/api/courses/${courseId}/units`)
.then( res => {
debugger
dispatch({ type: GET_UNITS, units: res.data, headers: res.headers })
dispatch(setHeaders(res.headers))
})
.catch( err => {
dispatch(setHeaders(err.headers));
dispatch(setFlash('Failed To Retrieve Units', 'red'));
});
}
}
export default (state = , action) => {
switch (action.type) {
case GET_UNITS:
return action.units;
default:
return state;
}
};
Edit 3: It is now working with minor changes.
change 1:
const mapStateToProps = (state) => {
return { units: state.units, course: state.course }
}
change 2:
componentDidUpdate(prevProps) {
const { dispatch, course } = this.props
if (prevProps.course.id !== course.id)
dispatch(getUnits(course.id))
}
apparently when it mounted, it didn't have the props it needed which is part of why courses wasn't defined. It rendered before it grabbed courses.
@devserkan I've updated with the entire component. When you say "this is not possible if your component renders correctly", what are a couple of the most common reasons that my component might not mount correctly?
– TheLionPear
Jun 29 at 22:14
There are lots of thing is going on here :) First of all getUnits is an action creator, you can import it wherever you want but it is confusing. Secondly, if you will use your action creator like this you should use a middleware something like redux-thunk. Also, as far as I know
dispatch
does not belong to react
, rather than react
it is a part of redux
. Also, in your units function there is a map, but it does not return antyhing, no return there. This is not your problem right now but keep this in your mind.– devserkan
Jun 29 at 22:27
dispatch
react
react
redux
And when I say "impossible" if your component renders somehow, if you see something at least then componentDidMount should run, other than that if render fails then it won't run.
– devserkan
Jun 29 at 22:28
I am not sure how you are running the app but probably the
console.log
is hit correctly. Only the logs are probably not logged to where you expect. Don't bother with code, no problem is there. Inspect your build system.– Sulthan
Jun 30 at 5:57
console.log
1 Answer
1
Your getUnits
action that you dispatch inside componentDidMount
expects courseId
param, but you are passing none.
getUnits
componentDidMount
courseId
TypeError: Cannot read property 'id' of undefined
componentDidMount() {
console.log ("component did mount!")
debugger
// missing param, any props we can use here?
this.props.dispatch(getUnits(/* missing courseId param */))
debugger
}
// Action that expects courseId param
export const getUnits = (courseId) => { ... }
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Welcome. Actually this is not possible if your component renders correctly. Does it? Also, if it possible put your other parts of component, so people can see what is going on here.
– devserkan
Jun 29 at 22:02