Uncaught TypeError: _this2.props.selectBook is not a function
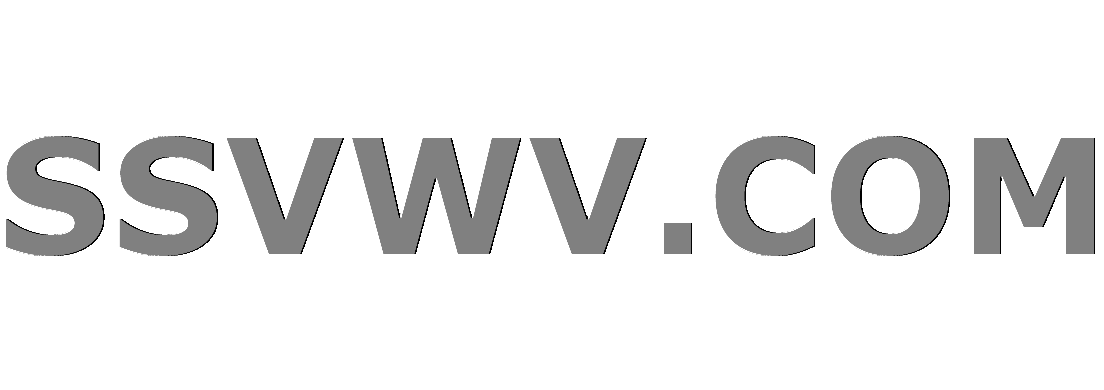
Multi tool use
Uncaught TypeError: _this2.props.selectBook is not a function
im a newbie for reactjs and was following a react basic course on udemy.
I get the following error on my console log. Can anybody assist with me?.
bundle.js:21818 Uncaught TypeError: _this2.props.selectBook is not a function
Any help would be appreciated. Thanks.
containers/book-list.js
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { selectBook } from '../actions/index';
import { bindActionCreators } from 'redux';
class BookList extends Component {
renderList() {
return this.props.books.map((book) => {
return (
<li
key={book.title}
onClick={() => this.props.selectBook(book)}
className="list-group-item">
{book.title}
</li>
);
});
}
render() {
return (
<ul className="list-group col-sm-4">
{this.renderList()}
</ul>
)
}
}
function mapStateToProps(state) {
return {
books: state.books
};
}
//Anythin returned from this function will end up as props
// on the BookList container
function mapDispatchToProps(dispatch) {
// whenever selectBook is called, the result should be passed
// to all of our reducers
return bindActionCreators({ selectBook: selectBook }, dispatch);
}
// Promote BookList from a component to a container - it needs to know
// about this new dispatch method, selectBook. Make it available
// as a prop.
export default connect(mapStateToProps)(BookList);
actions/index.js
export function selectBook(book) {
console.log('A book has been selected:', book.title);
}
components/app.js
import React, { Component } from 'react';
import BookList from '../containers/book-list';
export default class App extends Component {
render() {
return (
);
}
}
how can i show multiple files? @kinduser
– Cody
Jul 16 '17 at 22:00
Post the file where you are using
selectBook
variable.– kind user
Jul 16 '17 at 22:01
selectBook
added in the question description @kinduser thanks for quick prompt.
– Cody
Jul 16 '17 at 22:04
Why did you place
selectBook
function inside actions
folder? It's misleading. Actions are - let's say - reserved for redux. Anyways - try to move that function into book-list
file and see if the error persists.– kind user
Jul 16 '17 at 22:08
selectBook
actions
book-list
4 Answers
4
Found the answer myself.
// didnt have included the mapDispatchToProps function call in below lines.
export default connect(mapStateToProps, mapDispatchToProps)(BookList);
this is what did it for me, I am following Stephen Grider's udemy course
– Charles Harring
Dec 18 '17 at 17:19
Use import selectBook from '../actions/index'
instead of import { selectBook } from '../actions/index';
import selectBook from '../actions/index'
import { selectBook } from '../actions/index';
Make sure to export selectBook action so that it's available inside the application
export function selectBook() {...}
seems to be already the case
– Gabriel Bleu
Nov 3 '17 at 16:38
For someone who already included the mapDispatchToProps
function call, i.e. export default connect(mapStateToProps, mapDispatchToProps)(BookList);
mapDispatchToProps
export default connect(mapStateToProps, mapDispatchToProps)(BookList);
Look through actions/index.js and see if you're using default export or not.
If not you would require {}
around while importing selectBook.
{}
if (using default export) {
import selectBook from '../actions/index';
}
else {
import { selectBook } from '../actions/index';
}
Cheers.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Provide whole code.
– kind user
Jul 16 '17 at 21:50