How to implement setTimeout before blocking while in nodeJS
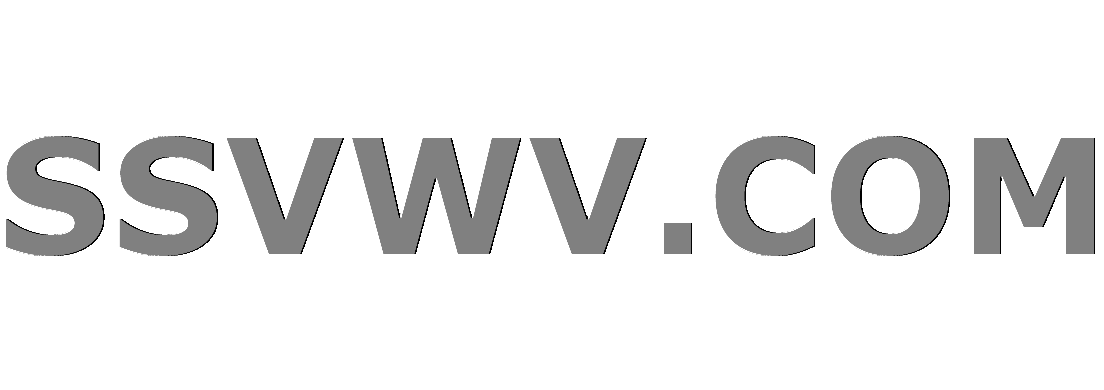
Multi tool use
How to implement setTimeout before blocking while in nodeJS
I have blocking code like this:
let a = 0;
setTimeout(() => {
console.log(a);
process.exit();
}, 1000);
while (true) {
a++;
}
How can I use nexttick
function to unblock timeout? Or somethink like this to implement setTimeout before blocking while?
nexttick
while
Can you give me a example please
– drothouu
Jul 3 at 7:57
2 Answers
2
The callback you register in setTimeout will never be called, since you're blocking the main thread.
You could setInterval to achieve something similar, e.g.
let a = 2;
setTimeout(() => {
console.log(a);
process.exit();
}, 1000);
setInterval(() => {
// Square a
a = a * a;
}, 200);
Blocking the main thread isn't really a good idea in Node! There's usually a better way to do things in an asynchronous way.
Thank you for your reply, order of execution is important. What about when I want to fire
a = a * a;
– drothouu
Jul 3 at 8:06
a = a * a;
You can control order of execution by setting the interval length for setInterval and setTimeout, e.g. run setInterval 100 times before setTimeout callback fires once!
– Terry Lennox
Jul 3 at 8:13
Node gets locked when you run synchronous code, meaning if you have a long-running loop it may be helpful to structure it in an asynchronous way so it becomes non-blocking.
while(true) {
doSomeSyncronousStuff();
}
should become something like
let someDelay = 0;
let loop = function(continueRunning){
doSomeSyncronousStuff();
if(continueRunning) {
// delay of as little as 0 pushes execution to at least next tick
setTimeout(loop,someDelay,continueRunning);
}
};
loop(true);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I would suggest not making a blocking
while
loop in the first place. Consider using callbacks or promises between loop iterations or reviewing why you need to loop this way in the first place– serakfalcon
Jul 3 at 7:55